How to determine whether the time is the previous day in Go language?
How to determine whether the time is the previous day in Go language?
In the Go language, we often need to operate and judge dates and times. Sometimes we need to determine whether a given time is the previous day, which is very common in some demand scenarios. This article will introduce how to determine whether the time is the previous day in Go language and provide specific code examples.
First, we need to import the "time" package in the Go language standard library to facilitate time operations. The code is as follows:
import ( "time" )
Next, we need to write a function that accepts a parameter of type time.Time, and then determines whether the time is the previous day. The following is a complete code example:
package main import ( "fmt" "time" ) func isYesterday(t time.Time) bool { now := time.Now() yesterday := now.AddDate(0, 0, -1) year, month, day := t.Date() yearNow, monthNow, dayNow := yesterday.Date() if year == yearNow && month == monthNow && day == dayNow { return true } return false } func main() { t := time.Date(2022, time.September, 21, 0, 0, 0, 0, time.UTC) if isYesterday(t) { fmt.Println("给定的时间是昨天!") } else { fmt.Println("给定的时间不是昨天!") } }
In the above code, we define a function named isYesterday, which accepts a parameter t of type time.Time and returns a Boolean value indicating that Whether the specified time is the previous day. We first get the current time now, and then get yesterday's time yesterday through now.AddDate(0, 0, -1). Next, we compare the parameter t with the year, month, and day of yesterday. If they are equal, it means that the given time is the previous day and return true; otherwise, return false.
In the main function, we create a time t, and then call the isYesterday function to determine whether the time is the previous day and output the result.
Summary: Through the introduction and code examples of this article, you should now know how to determine whether the time is the previous day in Go language. Hope this helps!
The above is the detailed content of How to determine whether the time is the previous day in Go language?. For more information, please follow other related articles on the PHP Chinese website!
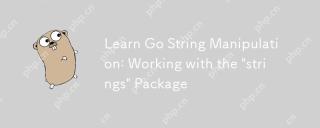
Go's "strings" package provides rich features to make string operation efficient and simple. 1) Use strings.Contains() to check substrings. 2) strings.Split() can be used to parse data, but it should be used with caution to avoid performance problems. 3) strings.Join() is suitable for formatting strings, but for small datasets, looping = is more efficient. 4) For large strings, it is more efficient to build strings using strings.Builder.
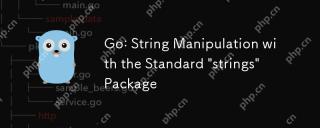
Go uses the "strings" package for string operations. 1) Use strings.Join function to splice strings. 2) Use the strings.Contains function to find substrings. 3) Use the strings.Replace function to replace strings. These functions are efficient and easy to use and are suitable for various string processing tasks.
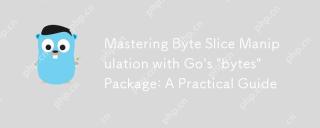
ThebytespackageinGoisessentialforefficientbyteslicemanipulation,offeringfunctionslikeContains,Index,andReplaceforsearchingandmodifyingbinarydata.Itenhancesperformanceandcodereadability,makingitavitaltoolforhandlingbinarydata,networkprotocols,andfileI
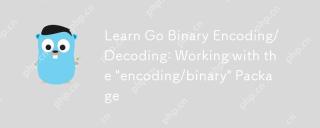
Go uses the "encoding/binary" package for binary encoding and decoding. 1) This package provides binary.Write and binary.Read functions for writing and reading data. 2) Pay attention to choosing the correct endian (such as BigEndian or LittleEndian). 3) Data alignment and error handling are also key to ensure the correctness and performance of the data.
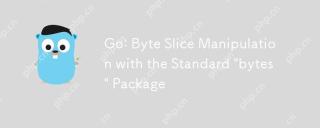
The"bytes"packageinGooffersefficientfunctionsformanipulatingbyteslices.1)Usebytes.Joinforconcatenatingslices,2)bytes.Bufferforincrementalwriting,3)bytes.Indexorbytes.IndexByteforsearching,4)bytes.Readerforreadinginchunks,and5)bytes.SplitNor
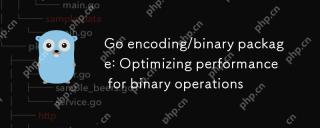
Theencoding/binarypackageinGoiseffectiveforoptimizingbinaryoperationsduetoitssupportforendiannessandefficientdatahandling.Toenhanceperformance:1)Usebinary.NativeEndianfornativeendiannesstoavoidbyteswapping.2)BatchReadandWriteoperationstoreduceI/Oover
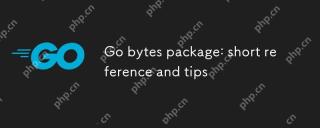
Go's bytes package is mainly used to efficiently process byte slices. 1) Using bytes.Buffer can efficiently perform string splicing to avoid unnecessary memory allocation. 2) The bytes.Equal function is used to quickly compare byte slices. 3) The bytes.Index, bytes.Split and bytes.ReplaceAll functions can be used to search and manipulate byte slices, but performance issues need to be paid attention to.
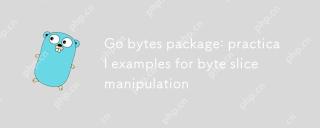
The byte package provides a variety of functions to efficiently process byte slices. 1) Use bytes.Contains to check the byte sequence. 2) Use bytes.Split to split byte slices. 3) Replace the byte sequence bytes.Replace. 4) Use bytes.Join to connect multiple byte slices. 5) Use bytes.Buffer to build data. 6) Combined bytes.Map for error processing and data verification.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 English version
Recommended: Win version, supports code prompts!

Atom editor mac version download
The most popular open source editor

Notepad++7.3.1
Easy-to-use and free code editor

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
