What are the main application areas of JavaScript?
What are the main application areas of JavaScript?
JavaScript is a scripting language widely used in web development, which can add interactivity and dynamic effects to web pages. In addition to being widely used in web development, JavaScript can also be used in various other fields. The main application areas of JavaScript and corresponding code examples will be introduced in detail below.
1. Web development
The most common application field of JavaScript is in web development. JavaScript can realize dynamic effects, interactive functions, form verification, etc. of web pages. The following is a sample code for a simple web page pop-up window:
<!DOCTYPE html> <html> <head> <title>JavaScript弹窗示例</title> </head> <body> <button onclick="alert('Hello, World!')">点击弹窗</button> </body> </html>
2. Web application development
JavaScript can be used to develop various types of web applications, including single page applications (SPA) and Responsive web applications. Through frameworks such as React, Angular, Vue.js, etc., developers can use JavaScript to build complex front-end applications. The following is an example of a simple counter developed using the React framework:
import React, { useState } from "react"; function Counter() { const [count, setCount] = useState(0); return ( <div> <p>Count: {count}</p> <button onClick={() => setCount(count + 1)}>增加</button> </div> ); } export default Counter;
3. Back-end development
In addition to being widely used in front-end development, JavaScript can also be used for back-end development. Node.js is a JavaScript-based runtime environment that allows JavaScript to run on the server side and provides the functions required for back-end development. The following is a simple Node.js server code example:
const http = require('http'); const server = http.createServer((req, res) => { res.statusCode = 200; res.setHeader('Content-Type', 'text/plain'); res.end('Hello, World! '); }); server.listen(3000, '127.0.0.1', () => { console.log('Server running at http://127.0.0.1:3000/'); });
4. Game development
JavaScript can also be used to develop simple Web games. Through technologies such as Canvas or WebGL, various kind of game effect. The following is a simple example of using Canvas to draw graphics:
<!DOCTYPE html> <html> <head> <title>Canvas绘制图形示例</title> </head> <body> <canvas id="myCanvas" width="200" height="100"></canvas> <script> const canvas = document.getElementById('myCanvas'); const ctx = canvas.getContext('2d'); ctx.fillStyle = 'blue'; ctx.fillRect(10, 10, 50, 50); </script> </body> </html>
5. Mobile application development
JavaScript can also be used to develop mobile applications. Through frameworks such as React Native or Ionic, developers can Build cross-platform mobile apps using JavaScript. The following is a simple React Native sample code:
import React from 'react'; import { View, Text, StyleSheet } from 'react-native'; const App = () => { return ( <View style={styles.container}> <Text>Hello, World!</Text> </View> ); } const styles = StyleSheet.create({ container: { flex: 1, justifyContent: 'center', alignItems: 'center', }, }); export default App;
Summary: JavaScript is a powerful and multi-purpose scripting language that is widely used in web development, web application development, back-end development, game development and mobile Application development and other fields. Through different frameworks and technologies, developers can use JavaScript to achieve various complex functions and effects.
The above is the detailed content of What are the main application areas of JavaScript?. For more information, please follow other related articles on the PHP Chinese website!

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
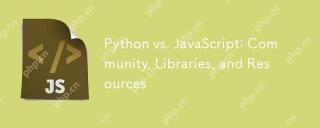
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
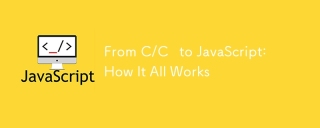
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
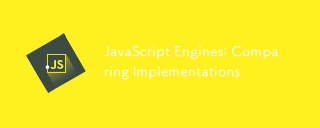
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
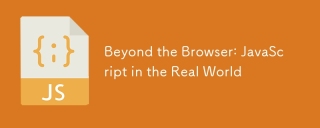
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
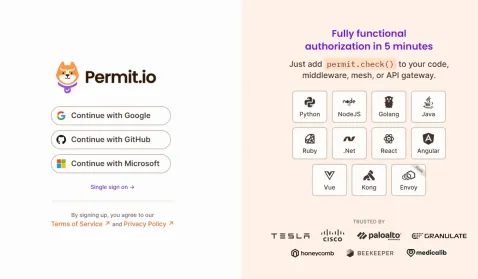
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
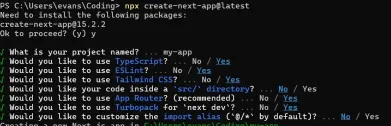
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
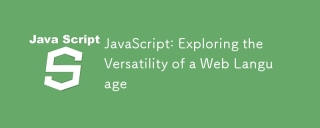
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
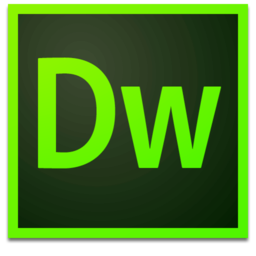
Dreamweaver Mac version
Visual web development tools
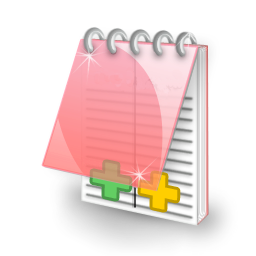
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Atom editor mac version download
The most popular open source editor
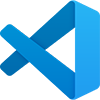
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
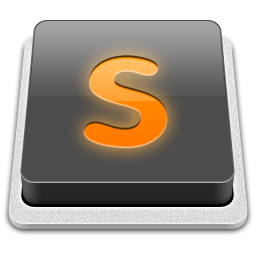
SublimeText3 Mac version
God-level code editing software (SublimeText3)