PHP7 OAuth2 extension installation method sharing
Title: PHP7 OAuth2 extension installation method sharing, with specific code examples
OAuth2 is a common authorization framework used to protect APIs. By using the OAuth2 authorization framework, developers can implement secure user authentication and access control. In PHP development, installing the OAuth2 extension is a crucial step. This article will share how to install the OAuth2 extension in PHP7, and attach specific code examples.
Install OAuth2 extension
- Check PHP version
First, make sure your PHP version is PHP7 or higher. The OAuth2 extension is available for PHP7 and above.
- Install necessary dependencies
Before installing the OAuth2 extension, you need to ensure that the system has some necessary dependencies installed. These dependencies can be installed using the following command:
sudo apt-get install php7.0-dev sudo apt-get install libpcre3-dev
- Download the OAuth2 extension
Then, download the source code of the OAuth2 extension from the official repository. The source code of the OAuth2 extension can be cloned from GitHub using the following command:
git clone https://github.com/bshaffer/oauth2-server-php.git
- Compile and Install
Go into the OAuth2 extension source code directory, and Execute the following commands to compile and install:
cd oauth2-server-php phpize ./configure make sudo make install
- Enable OAuth2 extension
Edit the PHP configuration file (php.ini) and add the following lines to enable OAuth2 extension:
extension=oauth2.so
- Restart PHP
Finally, restart the PHP service to make the configuration take effect:
sudo service php7.0-fpm restart
Code example
A simple OAuth2 authentication example is provided below to demonstrate the use of OAuth2 extension:
<?php require 'vendor/autoload.php'; use OAuth2Request; use OAuth2Response; // 认证服务器的配置 $dsn = 'mysql:dbname=oauth2;host=localhost'; $username = 'root'; $password = ''; // 创建OAuth2服务器 $server = new OAuth2Server(new OAuth2StoragePdo(array('dsn' => $dsn, 'username' => $username, 'password' => $password))); // 添加支持的授权类型 $server->addGrantType(new OAuth2GrantTypeAuthorizationCode($server)); // 处理请求 $request = OAuth2Request::createFromGlobals(); $response = new OAuth2Response(); // 验证请求是否有效 if (!$server->validateAuthorizeRequest($request, $response)) { $response->send(); die; } // 显示认证页面 if (empty($_POST)) { exit(' <form method="post"> <label>Do you authorize the app to access your information?</label><br /> <input type="submit" name="authorized" value="yes"> <input type="submit" name="authorized" value="no"> </form> '); } // 处理用户授权 $server->handleAuthorizeRequest($request, $response, ($_POST['authorized'] === 'yes'), null); $response->send();
The above is a simple OAuth2 authentication example, covering the common usage of OAuth2 authorization code. Please make appropriate modifications and extensions according to actual needs.
So far, we have shared how to install the OAuth2 extension in PHP7 and provided a simple code example. Hope this helps, and wish you develop more secure and reliable applications using OAuth2 extensions!
The above is the detailed content of PHP7 OAuth2 extension installation method sharing. For more information, please follow other related articles on the PHP Chinese website!
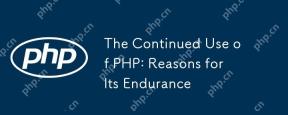
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
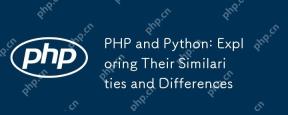
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
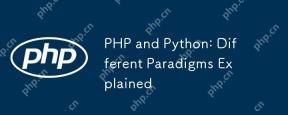
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
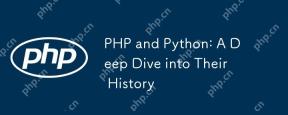
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
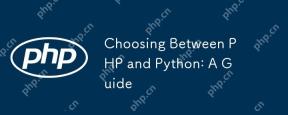
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
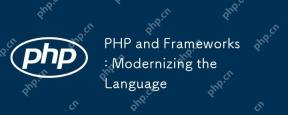
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
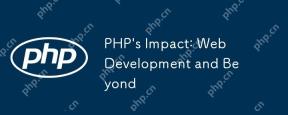
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
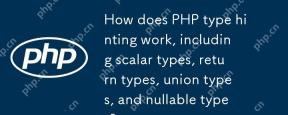
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
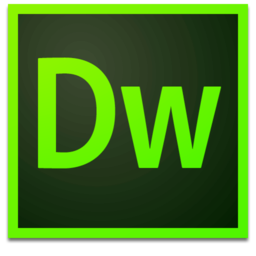
Dreamweaver Mac version
Visual web development tools
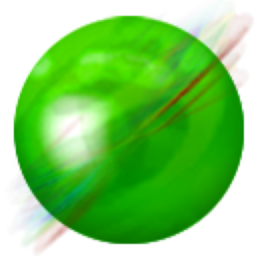
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
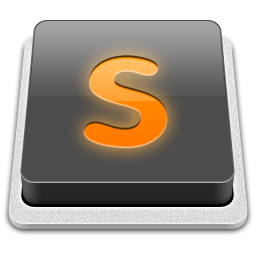
SublimeText3 Mac version
God-level code editing software (SublimeText3)