


Detailed explanation of using for loop to perform flip operation in Go language
Title: Detailed analysis of using for loop to flip operations in Go language
In Go language, using for loop to flip data structures such as arrays and slices is a common requirement. Through a for loop, you can traverse the elements in an array or slice and reverse the order of the elements. This article will introduce in detail how to use for loops to implement data flipping operations, and give specific code examples.
1. Flip the array
First, let’s look at how to use a for loop to flip the array. Suppose we have an array of integers arr
, and we want to rearrange the elements in the array in reverse order. This operation can be achieved through a for loop and temporary variables. The specific code is as follows:
package main import "fmt" func reverseArray(arr []int) { n := len(arr) for i := 0; i < n/2; i++ { arr[i], arr[n-1-i] = arr[n-1-i], arr[i] } } func main() { arr := []int{1, 2, 3, 4, 5} fmt.Println("原始数组:", arr) reverseArray(arr) fmt.Println("翻转后的数组:", arr) }
In the above code, we define a reverseArray
function to implement the flip operation of the array. In the main
function, we define an integer array arr
, and then call the reverseArray
function to flip the array. After running the program, you can see the flipped array result output.
2. Flip slices
In addition to arrays, we often need to flip slices. Similar to arrays, slices can be flipped through a for loop. The following is a sample code for flipping a slice:
package main import "fmt" func reverseSlice(slice []int) { n := len(slice) for i := 0; i < n/2; i++ { slice[i], slice[n-1-i] = slice[n-1-i], slice[i] } } func main() { slice := []int{6, 7, 8, 9, 10} fmt.Println("原始切片:", slice) reverseSlice(slice) fmt.Println("翻转后的切片:", slice) }
In the above code, we define a reverseSlice
function to flip the elements in the slice, and use it in the main
function The slices are flipped. By running the program, you can see the result output after flipping the slice elements.
Through the above code example, we can clearly understand how to use for loops to implement flip operations of arrays and slices. This method is simple and efficient, and can meet the common needs for data structure flipping in daily development. I hope that readers can become more proficient in using the for loop in Go language by reading this article.
The above is the detailed content of Detailed explanation of using for loop to perform flip operation in Go language. For more information, please follow other related articles on the PHP Chinese website!
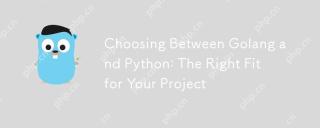
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
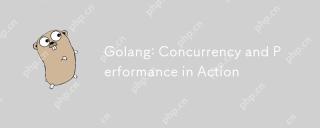
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
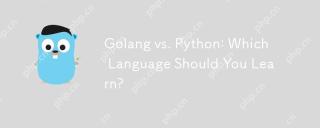
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
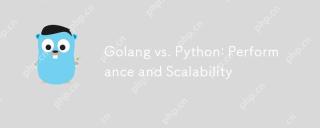
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
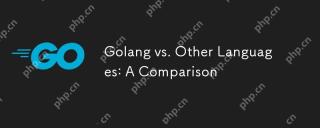
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
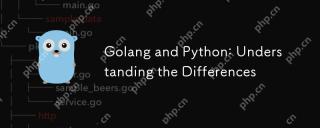
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
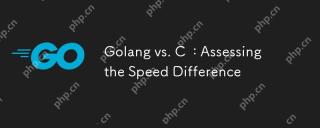
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
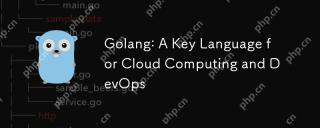
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
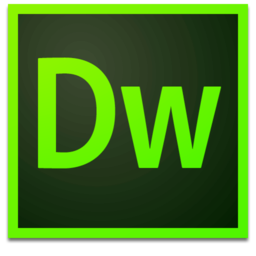
Dreamweaver Mac version
Visual web development tools
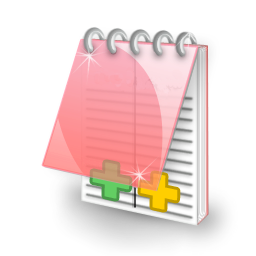
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
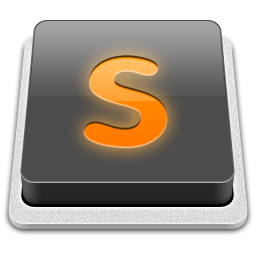
SublimeText3 Mac version
God-level code editing software (SublimeText3)