Deeply understand the technical features and value of Go language
Go language is a programming language developed by Google. It was first released in 2009 and has received widespread attention for its simplicity, efficiency, and ease of learning. The Go language is designed to handle applications with excellent concurrent performance, while also having fast compilation speed and concise coding style. This article will delve into the technical features and value of the Go language, and attach specific code examples to further illustrate.
First, the concurrency model of Go language is very powerful. The Go language provides a simple and effective concurrent programming model through goroutines and channels. Goroutine is a lightweight thread that can be easily created and managed in the program and has low overhead. Through goroutine, we can implement highly concurrent programs and improve the response speed and performance of the program.
The following is a simple goroutine sample code:
package main import ( "fmt" "time" ) func hello() { fmt.Println("Hello, goroutine!") } func main() { go hello() time.Sleep(1 * time.Second) }
In this code, we define a hello()
function, and then pass go hello ()
to create a goroutine and execute the hello()
function in it. Use time.Sleep(1 * time.Second)
to wait for the goroutine execution to complete. Running this code, we will see the output "Hello, goroutine!".
Second, the standard library of Go language is rich in functions. The standard library of the Go language provides many powerful functions and tools, including support for network, file, database and other operations, as well as concurrent programming, data structures, encryption and decryption and other functions. This allows developers to easily use these libraries to implement various functions, greatly improving development efficiency.
The following is a code example that uses the http package in the Go language standard library to create a simple web server:
package main import ( "fmt" "net/http" ) func handler(w http.ResponseWriter, r *http.Request) { fmt.Fprintf(w, "Hello, World!") } func main() { http.HandleFunc("/", handler) http.ListenAndServe(":8080", nil) }
In this code, we use http.HandleFunc ()
function to register a handler function handler
, and then call http.ListenAndServe(":8080", nil)
to start a Web server listening on port 8080. When there is a request for access, the server will call the handler
function and return "Hello, World!".
Third, the compilation speed of Go language is fast. The compiler of the Go language is very fast and can compile and build the code in a few seconds. For developers, this means they can iterate and debug code faster, improving development efficiency.
In summary, the Go language has technical features such as a powerful concurrency model, a rich standard library, and fast compilation speed. These features give the Go language significant advantages in developing high-performance, concurrent applications. . Through the code examples introduced in this article, readers can have a deeper understanding and mastery of the technical features and value of the Go language. I hope this article can provide some help for readers in the learning and application of the Go language.
The above is the detailed content of Deeply understand the technical features and value of Go language. For more information, please follow other related articles on the PHP Chinese website!
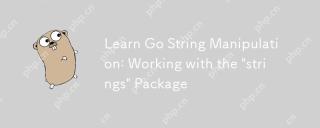
Go's "strings" package provides rich features to make string operation efficient and simple. 1) Use strings.Contains() to check substrings. 2) strings.Split() can be used to parse data, but it should be used with caution to avoid performance problems. 3) strings.Join() is suitable for formatting strings, but for small datasets, looping = is more efficient. 4) For large strings, it is more efficient to build strings using strings.Builder.
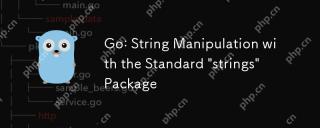
Go uses the "strings" package for string operations. 1) Use strings.Join function to splice strings. 2) Use the strings.Contains function to find substrings. 3) Use the strings.Replace function to replace strings. These functions are efficient and easy to use and are suitable for various string processing tasks.
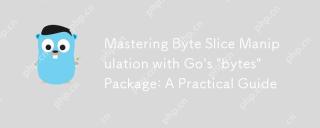
ThebytespackageinGoisessentialforefficientbyteslicemanipulation,offeringfunctionslikeContains,Index,andReplaceforsearchingandmodifyingbinarydata.Itenhancesperformanceandcodereadability,makingitavitaltoolforhandlingbinarydata,networkprotocols,andfileI
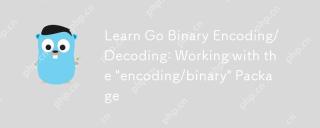
Go uses the "encoding/binary" package for binary encoding and decoding. 1) This package provides binary.Write and binary.Read functions for writing and reading data. 2) Pay attention to choosing the correct endian (such as BigEndian or LittleEndian). 3) Data alignment and error handling are also key to ensure the correctness and performance of the data.
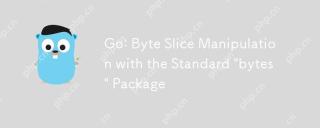
The"bytes"packageinGooffersefficientfunctionsformanipulatingbyteslices.1)Usebytes.Joinforconcatenatingslices,2)bytes.Bufferforincrementalwriting,3)bytes.Indexorbytes.IndexByteforsearching,4)bytes.Readerforreadinginchunks,and5)bytes.SplitNor
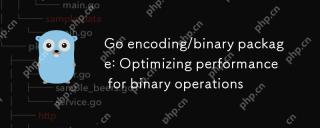
Theencoding/binarypackageinGoiseffectiveforoptimizingbinaryoperationsduetoitssupportforendiannessandefficientdatahandling.Toenhanceperformance:1)Usebinary.NativeEndianfornativeendiannesstoavoidbyteswapping.2)BatchReadandWriteoperationstoreduceI/Oover
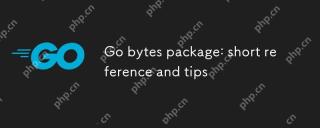
Go's bytes package is mainly used to efficiently process byte slices. 1) Using bytes.Buffer can efficiently perform string splicing to avoid unnecessary memory allocation. 2) The bytes.Equal function is used to quickly compare byte slices. 3) The bytes.Index, bytes.Split and bytes.ReplaceAll functions can be used to search and manipulate byte slices, but performance issues need to be paid attention to.
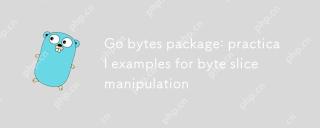
The byte package provides a variety of functions to efficiently process byte slices. 1) Use bytes.Contains to check the byte sequence. 2) Use bytes.Split to split byte slices. 3) Replace the byte sequence bytes.Replace. 4) Use bytes.Join to connect multiple byte slices. 5) Use bytes.Buffer to build data. 6) Combined bytes.Map for error processing and data verification.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
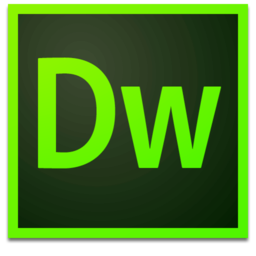
Dreamweaver Mac version
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
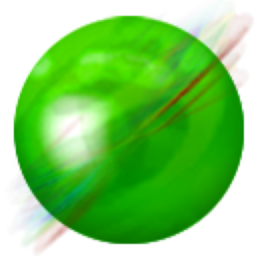
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
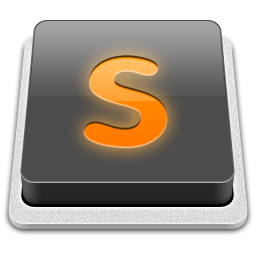
SublimeText3 Mac version
God-level code editing software (SublimeText3)
