PHP is a powerful server-side scripting language that is widely used in web development. In web development, we often need to interact with the database and execute query statements to obtain data. This article will introduce you to how to write query statements and usage examples in PHP.
1. Connect to the database
Before using PHP to query the database, you first need to establish a connection with the database. Generally, we will use the MySQL database as an example. The code to connect to the database is as follows:
$servername = "localhost"; $username = "root"; $password = ""; $dbname = "myDB"; // 创建连接 $conn = new mysqli($servername, $username, $password, $dbname); // 检测连接 if ($conn->connect_error) { die("连接失败: " . $conn->connect_error); }
2. Execute the query statement
Next, we can use PHP to execute the query statement to obtain data. The following is a simple query statement example to query all data in the table named "users":
$sql = "SELECT * FROM users"; $result = $conn->query($sql); if ($result->num_rows > 0) { // 输出数据 while($row = $result->fetch_assoc()) { echo "id: " . $row["id"]. " - Name: " . $row["name"]. " - Email: " . $row["email"]. "<br>"; } } else { echo "0 结果"; }
3. Parameterized query
In order to prevent SQL injection attacks, we should use parameters query. The following is an example of using parameterized query:
$stmt = $conn->prepare("SELECT * FROM users WHERE id = ?"); $stmt->bind_param("i", $id); $id = 1; $stmt->execute(); $result = $stmt->get_result(); if ($result->num_rows > 0) { while($row = $result->fetch_assoc()) { echo "id: " . $row["id"]. " - Name: " . $row["name"]. " - Email: " . $row["email"]. "<br>"; } } else { echo "0 结果"; } $stmt->close();
4. Close the connection
After completing the database query, we should close the connection with the database and release resources. The code to close the connection is as follows:
$conn->close();
Through the above examples, you can understand how to write query statements in PHP and learn how to use parameterized queries to improve data security. I wish you greater success in web development!
The above is the detailed content of PHP query statement usage example. For more information, please follow other related articles on the PHP Chinese website!
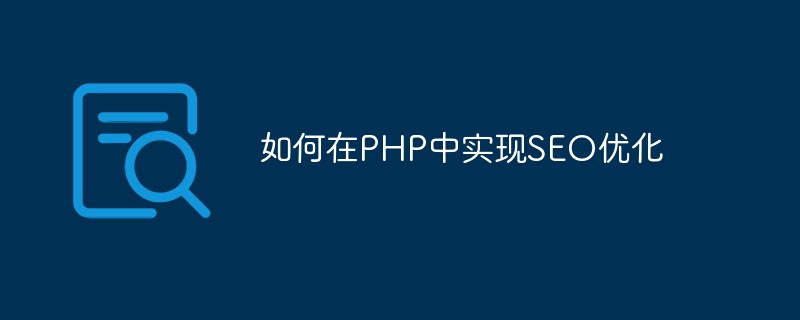
随着互联网的发展,SEO(SearchEngineOptimization,搜索引擎优化)已经成为了网站优化的重要一环。如果您想要使您的PHP网站在搜索引擎中获得更高的排名,就需要对SEO的内容有一定的了解了。本文将会介绍如何在PHP中实现SEO优化,内容包括网站结构优化、网页内容优化、外部链接优化,以及其他相关的优化技巧。一、网站结构优化网站结构对于S
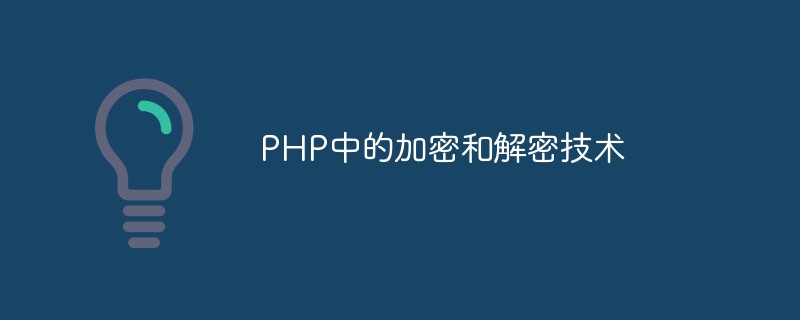
PHP是一种被广泛应用的Web开发语言,其加密和解密技术在数据安全性方面具有重要意义。本文将介绍PHP中的加密和解密技术,并探讨其在Web应用程序中的实际应用。一、加密技术加密技术是一种将普通文本转换为加密文本的过程。在PHP中,加密技术主要应用于传输数据的安全性,例如用户的登录信息、交易数据等。PHP中常见的加密技术如下:哈希加密哈希加密是将一个任意长度的
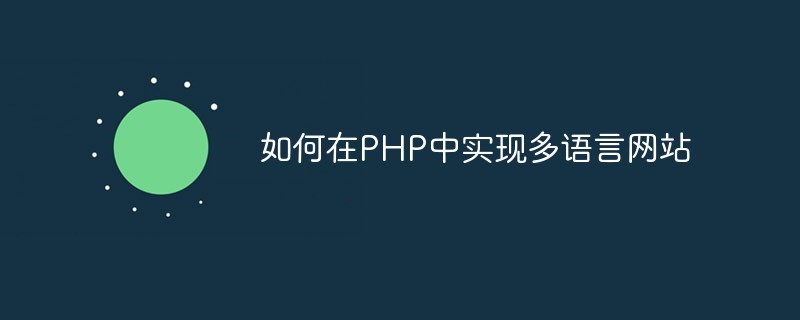
随着互联网的日益普及,越来越多的网站需要支持多语言。这是因为网站的受众群体可能来自不同的地区和文化背景,如果只提供单一语言的网站,可能会限制访问者的数量和体验。本文将介绍如何在PHP中实现多语言网站。一、语言文件的创建和设计语言文件是存储所有文本字符串及其对应翻译的文件,需要以特定的格式创建。在创建语言文件时,需要考虑以下几个方面:1.命名和存储位置文件名应
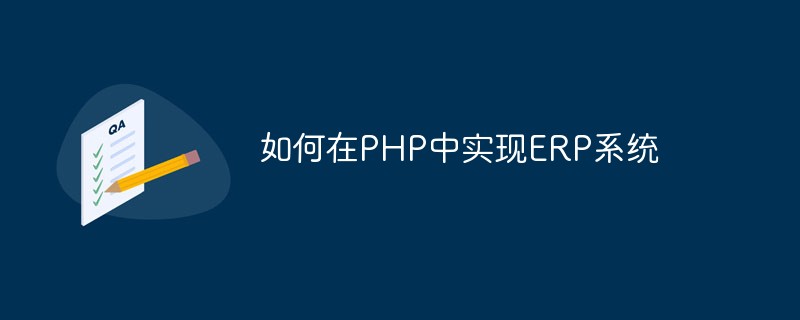
随着电子商务和企业管理的发展,许多企业开始寻找更好的方法来处理其日常业务流程。ERP系统是一种能够整合企业各种业务流程的软件工具。它提供了全面的功能,包括生产、销售、采购、库存、财务等方面,帮助企业提高效率、控制成本和提高客户满意度。而在PHP编程语言中,也能够实现ERP系统,这就需要我们掌握一些基本的知识和技术。下面,我们将深入探讨如何在PHP中实现ERP
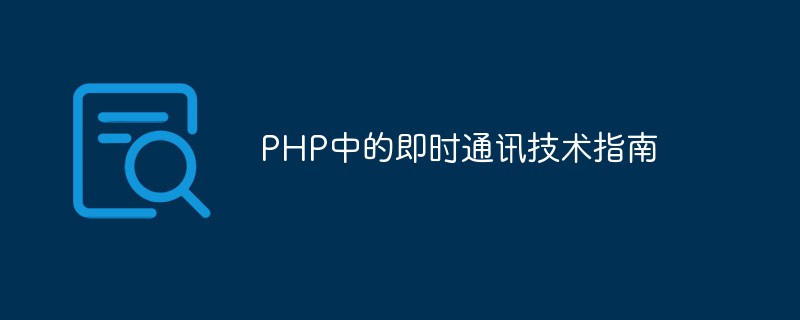
近年来,随着互联网技术的不断发展,即时通讯技术成为了各个领域中不可或缺的一部分,而在Web开发中,PHP作为一种广泛应用的服务器端脚本语言,也开始探索并应用即时通讯技术。本文将围绕PHP中的即时通讯技术,从通讯协议、技术方案、应用场景三个方面进行介绍和指南。一、通讯协议HTTP协议HTTP协议是Web开发中最常用的协议之一,适用于上传、下载、浏览网站等场景。
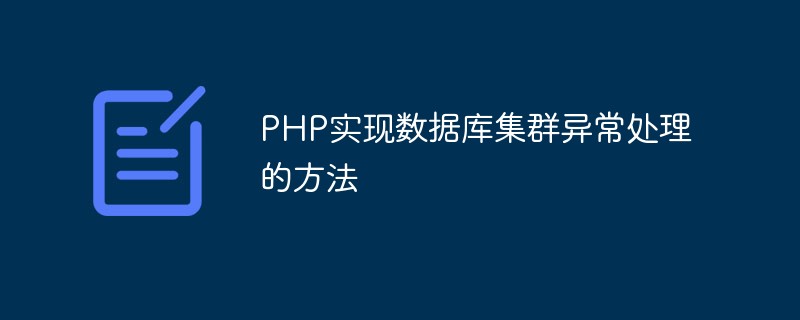
随着互联网的不断发展,越来越多的企业和组织开始规划数据库集群来满足其数据处理需求。数据库集群可能包含数百甚至数千个节点,因此在节点之间确保数据同步和协调非常重要。在该环境下,存在着很多的异常情况,如单节点故障,网络分区,数据同步错误等,并且需要实现实时检测和处理。本文将介绍如何使用PHP实现数据库集群异常处理。数据库集群的概述在数据库集群中,一个单独的
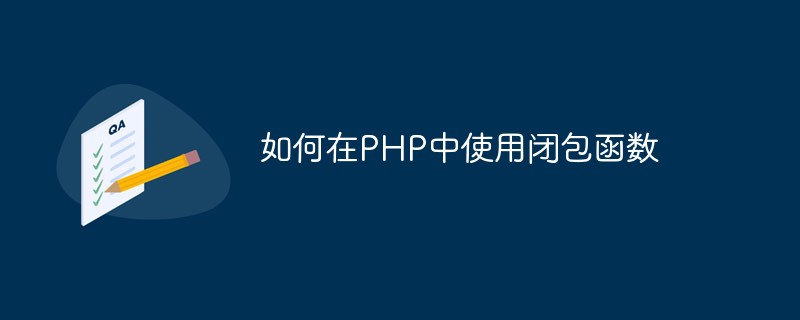
PHP闭包函数是指在声明函数时所定义的函数体内部所使用的变量和外部环境中的变量形成一个封闭的作用域,这种函数又被称为匿名函数。闭包函数在PHP中被广泛应用,可以用于实现事件处理、回调等一系列功能。本文将介绍如何在PHP中使用闭包函数,以及一些使用闭包函数的最佳实践。一、如何定义一个闭包函数定义一个闭包函数非常简单,只需要使用函数关键字followedby
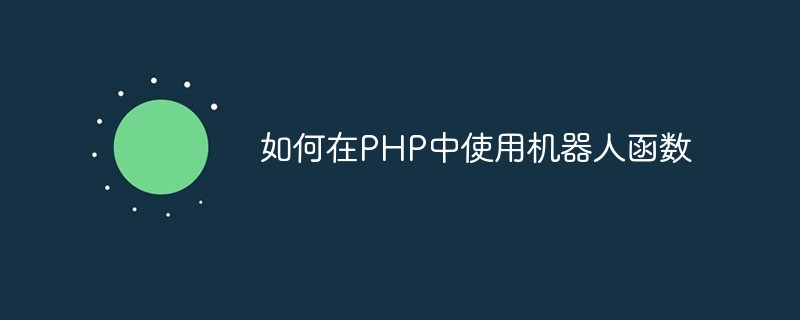
最近,随着人工智能技术的快速发展,机器人技术也逐渐得到了广泛的应用,其中,机器人函数成为了PHP编程语言中一个非常实用的工具。本文将介绍如何在PHP中使用机器人函数。什么是机器人函数机器人函数指在PHP编程语言中用于模拟机器人行为的一组函数。这些函数包括move()、turn()等,可以让我们编写出模拟机器人运动、转向等相关操作的代码。在实际应用中,机器人函


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
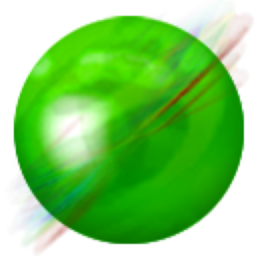
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
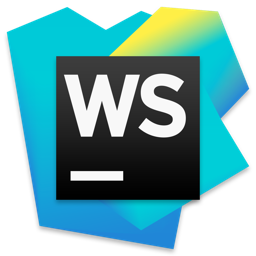
WebStorm Mac version
Useful JavaScript development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
