Learn how to implement singly linked list in Go language from scratch
Learn the implementation method of singly linked list in Go language from scratch
When learning data structures and algorithms, singly linked list is one of the basic and important data structures. This article will introduce how to use Go language to implement a singly linked list, and help readers better understand this data structure through specific code examples.
What is a singly linked list
A singly linked list is a linear data structure consisting of a series of nodes. Each node contains data and a pointer to the next node. The pointer of the last node points to null.
Basic operations of singly linked lists
Singly linked lists usually support several basic operations, including insertion, deletion and search. Now we will implement these operations step by step.
Create node structure
First, we need to define the node structure of a singly linked list:
type Node struct { data interface{} next *Node }
In the above structure, the data
field is used to store the data of the node, and the next
field is a pointer to the next node.
Initialize the linked list
Next, we need to define a LinkedList
structure to represent a singly linked list and provide some basic operation methods:
type LinkedList struct { head *Node } func NewLinkedList() *LinkedList { return &LinkedList{} }
Insert node
Method to implement inserting a node at the head of a singly linked list:
func (list *LinkedList) Insert(data interface{}) { newNode := &Node{data: data} if list.head == nil { list.head = newNode } else { newNode.next = list.head list.head = newNode } }
Delete node
Method to implement deletion of node with specified data:
func (list *LinkedList) Delete(data interface{}) { if list.head == nil { return } if list.head.data == data { list.head = list.head.next return } prev := list.head current := list.head.next for current != nil { if current.data == data { prev.next = current.next return } prev = current current = current.next } }
Find nodes
Method to implement finding nodes with specified data:
func (list *LinkedList) Search(data interface{}) bool { current := list.head for current != nil { if current.data == data { return true } current = current.next } return false }
Complete example
The following is a complete example code that demonstrates how to create a singly linked list, insert nodes, delete nodes and find nodes:
package main import "fmt" type Node struct { data interface{} next *Node } type LinkedList struct { head *Node } func NewLinkedList() *LinkedList { return &LinkedList{} } func (list *LinkedList) Insert(data interface{}) { newNode := &Node{data: data} if list.head == nil { list.head = newNode } else { newNode.next = list.head list.head = newNode } } func (list *LinkedList) Delete(data interface{}) { if list.head == nil { return } if list.head.data == data { list.head = list.head.next return } prev := list.head current := list.head.next for current != nil { if current.data == data { prev.next = current.next return } prev = current current = current.next } } func (list *LinkedList) Search(data interface{}) bool { current := list.head for current != nil { if current.data == data { return true } current = current.next } return false } func main() { list := NewLinkedList() list.Insert(1) list.Insert(2) list.Insert(3) fmt.Println(list.Search(2)) // Output: true list.Delete(2) fmt.Println(list.Search(2)) // Output: false }
总结
通过上面的代码示例,我们了解了如何使用Go语言实现单链表的基本操作。掌握了单链表的实现方法之后,读者可以进一步学习更复杂的数据结构以及相关算法,加深对计算机科学的理解和应用。希朐本文对读者有所帮助,谢谢阅读!
The above is the detailed content of Learn how to implement singly linked list in Go language from scratch. For more information, please follow other related articles on the PHP Chinese website!
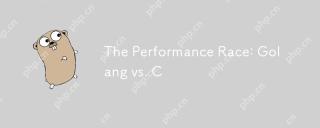
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
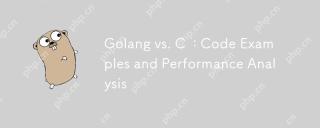
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
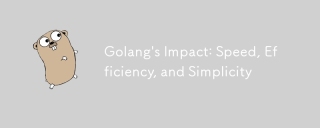
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
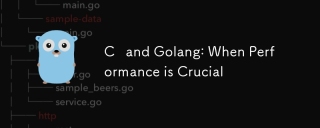
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
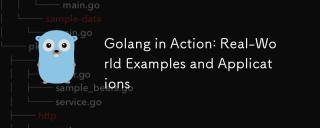
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
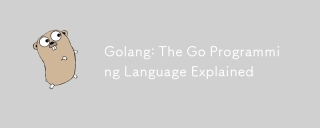
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
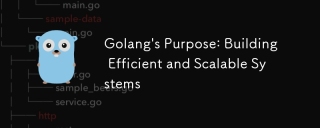
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
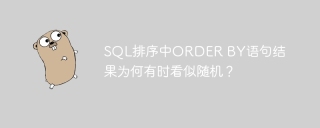
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
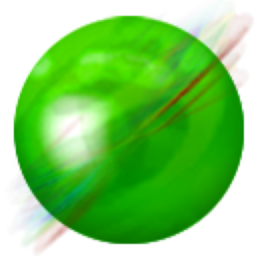
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Zend Studio 13.0.1
Powerful PHP integrated development environment

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor