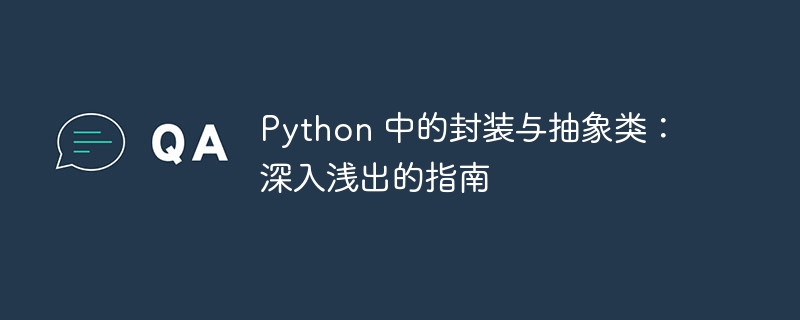
- Definition: Encapsulation is to hide data and methods in a class and expose only the necessary interfaces to control access to data.
- benefit:
- ImproveSecurity: Data can only be accessed through class methods, and external code cannot modify it directly.
- Improve maintainability: When modifying data inside the class, there is no need to worry about external code calls.
- Improved flexibility: Internal storage can be changed as needed without affecting external code.
Achieve encapsulation:
- Use access modifiers (
public
, protected
, private
) to control access permissions.
- Use the
self
variable inside the class to reference the instance.
- Avoid direct access to instance properties outside the class.
Abstract class
- Definition: An abstract class is a special class that limits class behavior. It contains abstract methods that cannot be instantiated.
- Purpose:
- Define an interface to force subclasses to implement these methods.
- Prevent the creation of instances that do not implement all abstract methods.
Implement abstract class:
- Use
@abstractmethod
decorator to mark abstract methods.
- Implement all abstract methods in subclasses.
- Abstract classes cannot be instantiated directly, only subclass instances can be created.
The difference between abstract methods and ordinary methods:
- Abstract methods are not implemented and must be implemented in subclasses.
- Common methods have been implemented in the parent class and can be inherited and overridden by subclasses.
Advantages of abstract classes:
- Ensure that all subclasses implement the required behavior.
- Improve code maintainability: avoid incomplete or inconsistent implementations by enforcing abstract methods.
- Promote interface consistency: Using the same abstract class in multiple modules can ensure interface consistency.
Disadvantages of abstract classes:
- Increased implementation complexity: all abstract methods must be implemented in subclasses.
- Possible lack of flexibility: subclasses cannot freely modify abstract methods.
The difference between encapsulation and abstract class
feature |
Encapsulation |
Abstract class |
Purpose |
Control data access |
Restrict class behavior |
Scope |
Internal class |
Parent class and subclass |
Mandatory |
Optional |
Mandatory |
Implementation |
Access modifier |
Abstract method |
Subclass |
Inheritable parent class methods |
All abstract methods must be implemented |
Instantiation |
Instanceable |
Not instantiable |
Application scenarios
Package:
- Sensitive data protection.
- Management of complex data.
- Improve code maintainability.
Abstract class:
- Define common interface.
- Ensure consistent behavior for inherited classes.
- Achieve polymorphism.
Best Practices
- Use encapsulation moderately and hide only necessary data.
- When using abstract classes, consider the implementation complexity and flexibility of subclasses.
- Use the
abstractmethod
suffix in abstract method names to improve code readability.
- When implementing an abstract method in a subclass, use
super()
to call the parent class implementation to take advantage of reuse.
The above is the detailed content of Encapsulation and abstract classes in Python: an in-depth guide. For more information, please follow other related articles on the PHP Chinese website!