How to read binary data using Golang
In Golang, we can use os
and encoding/binary
package to read binary data. Next, we will introduce in detail how to implement the method of reading binary data through these two packages, and attach specific code examples.
Use the os
package to read binary data
First, we need to use the os.Open
function to open a binary file and ensure that the file is opened successfully , and then create a byte slice to store the read binary data.
package main import ( "fmt" "os" ) func main() { file, err := os.Open("binary_file.bin") if err != nil { fmt.Println("File opening failed:", err) return } defer file.Close() fileInfo, _ := file.Stat() fileSize := fileInfo.Size() data := make([]byte, fileSize) _, err = file.Read(data) if err != nil { fmt.Println("Failed to read file:", err) return } fmt.Printf("Read binary data: %v ", data) }
Parsing binary data using the encoding/binary
package
Once we have read the binary data into byte slices, we can then use encoding/ binary
package to parse these binary data. We need to use the binary.Read
function and pass a io.Reader
and a target variable to parse the binary data into the specified type.
The following example demonstrates how to read a uint16 type data from a byte slice.
package main import ( "encoding/binary" "fmt" ) func main() { data := []byte{0x03, 0xE8} // 1000 (binary) = 3E8 (hex) = 1000 (decimal) var num uint16 err := binary.Read(bytes.NewReader(data), binary.LittleEndian, &num) if err != nil { fmt.Println("Failed to parse binary data:", err) return } fmt.Printf("Parsed uint16 data: %d ", num) }
Through the above code example, we can see how to use the os
and encoding/binary
packages in Golang to read and parse binary data. In practical applications, we can perform corresponding processing and analysis according to specific needs and binary data formats.
The above is the detailed content of How to read binary data using Golang. For more information, please follow other related articles on the PHP Chinese website!
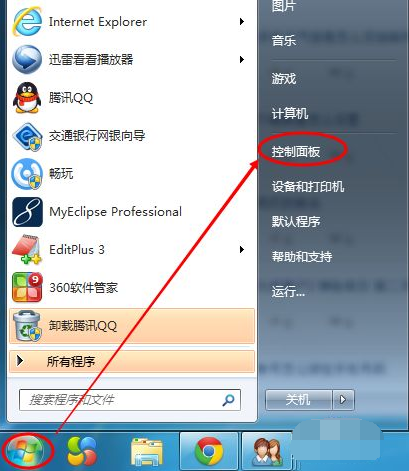
不同的电脑系统在调整屏幕亮度的操作方法上会有些不同,最近就有使用win7系统的网友不知道win7怎么调整屏幕亮度,看久了电脑眼睛比较酸痛。下面小编就教下大家win7调整屏幕亮度的方法。具体的操作步骤如下:1、点击win7电脑左下角的“开始”,在弹出的开始菜单中选择“控制面板”打开。2、在打开的控制面板中找到“电源选项”打开。3、也可以用鼠标右键电脑右下角的电源图标,在弹出的菜单中,点击“调整屏幕亮度”,如下图所示。两种方法都可以用。4、在打开的电源选项窗口的最下面可以看到屏幕亮度调整的滚动条,直
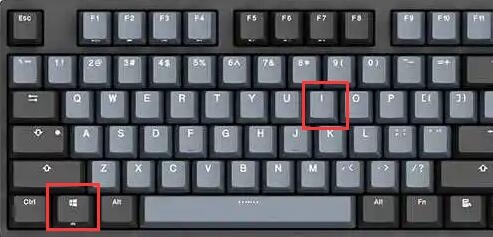
如果我们手头没有手机,只有电脑,但我们必须拍照,我们可以使用电脑内置的监控摄像头拍照,那么如何打开win10监控摄像头,事实上,我们只需要下载一个相机应用程序。打开win10监控摄像头的具体方法。win10监控摄像头打开照片的方法:1.首先,盘快捷键Win+i打开设置。2.打开后,进入个人隐私设置。3.然后在相机手机权限下打开访问限制。4.打开后,您只需打开相机应用软件。(如果没有,可以去微软店下载一个)5.打开后,如果计算机内置监控摄像头或组装了外部监控摄像头,则可以拍照。(因为人们没有安装摄
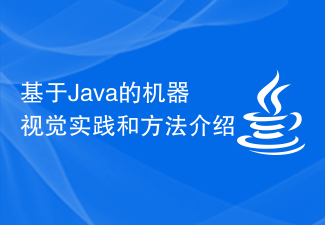
随着科技的不断发展,机器视觉技术在各个领域得到了广泛应用,如工业自动化、医疗诊断、安防监控等。Java作为一种流行的编程语言,其在机器视觉领域也有着重要的应用。本文将介绍基于Java的机器视觉实践和相关方法。一、Java在机器视觉中的应用Java作为一种跨平台的编程语言,具有跨操作系统、易于维护、高度可扩展等优点,对于机器视觉的应用具有一定的优越性。Java
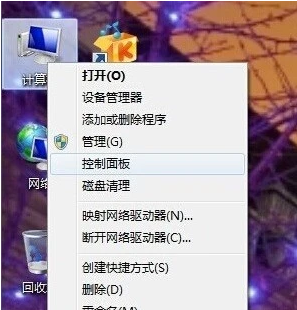
目前有很多屏幕亮度调整软件,我们可以通过使用软件进行快速调整或者通过显示器上自带的亮度功能进行调整。以下是详细的Win7屏幕亮度调整方式,您可以通过教程中的方法进行快速调整即可。Win7系统电脑怎么调节屏幕亮度教程:1、依次点击“计算机—右键—控制面板”,如果没有也可以在搜索框中进行搜索。2、点击控制面板下的“硬件和声音”,或者点击“外观和个性化”都可以。3、点击“NVIDIA控制面板”,有些显卡可能是AMD或者Intel的,请根据实际情况选择。4、调节图示中亮度滑块即可。5、还有一种方法,就是
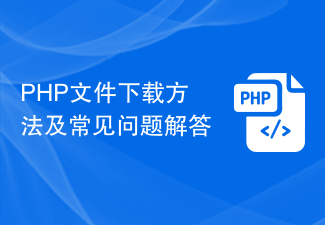
PHP是一个广泛使用的服务器端编程语言,它的许多功能和特性可以将其用于各种任务,包括文件下载。在本文中,我们将了解如何使用PHP创建文件下载脚本,并解决文件下载过程中可能出现的常见问题。一、文件下载方法要在PHP中下载文件,我们需要创建一个PHP脚本。让我们看一下如何实现这一点。创建下载文件的链接通过HTML或PHP在页面上创建一个链接,让用户能够下载文件。
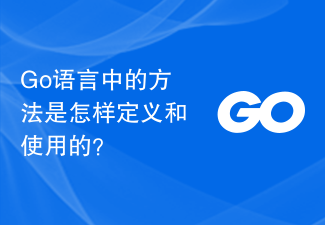
Go语言是近年来备受青睐的编程语言,因其简洁、高效、并发等特点而备受开发者喜爱。其中,方法(Method)也是Go语言中非常重要的概念。接下来,本文就将详细介绍Go语言中方法的定义和使用。一、方法的定义Go语言中的方法是带有接收器(Receiver)的函数,它是一个与某个类型绑定的函数。接收器可以是值类型或者指针类型。用于接收者的参数可以在方法名
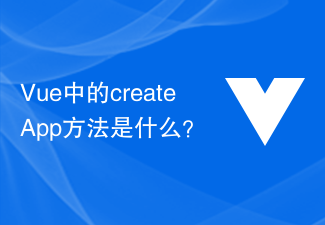
随着前端开发的快速发展,越来越多的框架被用来构建复杂的Web应用程序。Vue.js是流行的前端框架之一,它提供了许多功能和工具来简化开发人员构建高质量的Web应用程序。createApp()方法是Vue.js中的一个核心方法之一,它提供了一种简单的方式来创建Vue实例和应用程序。本文将深入探讨Vue中createApp方法的作用,其如何使用以及使用时需要了解
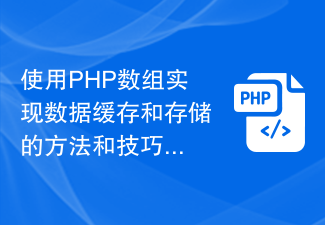
使用PHP数组实现数据缓存和存储的方法和技巧随着互联网的发展和数据量的急剧增长,数据缓存和存储成为了我们在开发过程中必须要考虑的问题之一。PHP作为一门广泛应用的编程语言,也提供了丰富的方法和技巧来实现数据缓存和存储。其中,使用PHP数组进行数据缓存和存储是一种简单而高效的方法。一、数据缓存数据缓存的目的是为了减少对数据库或其他外部数据源的访问次数,从而提高


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Chinese version
Chinese version, very easy to use

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
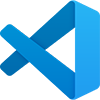
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft