


Linux process structure analysis: introduction to important components
Title: Analysis of Linux Process Structure: Introduction to Important Components
In the Linux operating system, the process is one of the most basic concepts in the operating system. A process is an execution instance of a program. It has its own memory space, code, data, execution status and other information in the operating system. Understanding the structure of the Linux process is of great significance to understanding the operating mechanism and scheduling principles of the operating system. This article will focus on the important components of the Linux process, including process control block (PCB), program segment, data segment, stack segment, etc., and provide an in-depth analysis of its internal structure and functions through specific code examples.
- Process Control Block (PCB)
The process control block is an important data structure used by the operating system to manage processes. It saves various information about the process, including process Status, process number, parent process number, priority, program counter, stack pointer, open file list, etc. The information in the PCB plays an important role in the operating system's process scheduling, resource management and allocation. The following is an example of the structure of a simple Linux process control block:
struct task_struct { pid_t pid; // process number pid_t ppid; // parent process number int priority; // priority unsigned long pc; // program counter unsigned long sp; // stack pointer struct file *files; //Open file list // other members... };
- Program segment
The program segment is the part of the process that stores code. It contains the executable code of the process. In Linux, program segments are usually stored in the .text segment, which is read-only and contains the program's instructions and function codes. Here is a simple code example that demonstrates how to access data in a program segment:
#include <stdio.h> int main() { char *message = "Hello, Linux process!"; printf("%s ", message); return 0; }
In the above code, the string "Hello, Linux process!" is stored in the program segment, and the content of the string is accessed and output through the pointer message.
- Data segment
The data segment is the part of the process that stores static data and global variables. It includes various variables defined in the program. In Linux, data segments are usually stored in the .data segment, and the data in this segment can be accessed read and write. Here is a simple example of a data segment:
#include <stdio.h> int global_var = 10; int main() { int local_var = 20; printf("Global variable: %d, Local variable: %d ", global_var, local_var); return 0; }
In the above code, the global variable global_var and the local variable local_var are stored in the data segment and stack segment respectively, and their values are accessed through pointers and output.
- Stack segment
The stack segment is the part of the process that stores function calls and local variables. It is used to store function parameters, return addresses, temporary variables, etc. In Linux, stack segments are usually stored in stack memory, and each function call allocates a memory space on the stack. The following is a simple stack segment example:
#include <stdio.h> void func(int n) { int sum = 0; for (int i = 1; i <= n; i ) { sum = i; } printf("Sum from 1 to %d: %d ", n, sum); } int main() { func(5); return 0; }
In the above code, the parameter n, local variable sum and loop variable i in the function func are all stored in the stack segment, and the use of the stack is demonstrated through function calls.
Summary: The internal structure of a Linux process consists of process control blocks, program segments, data segments, and stack segments, which together constitute the running environment and execution status of the process. By in-depth understanding of the internal structure and functions of Linux processes, you can better understand the working principles and process management mechanisms of the operating system. We hope that the introduction and code examples of this article can help readers better understand the Linux process structure and its important components.
The above is the detailed content of Linux process structure analysis: introduction to important components. For more information, please follow other related articles on the PHP Chinese website!
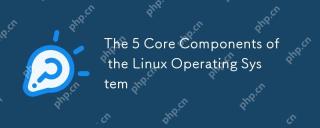
The five core components of the Linux operating system are: 1. Kernel, 2. System libraries, 3. System tools, 4. System services, 5. File system. These components work together to ensure the stable and efficient operation of the system, and together form a powerful and flexible operating system.
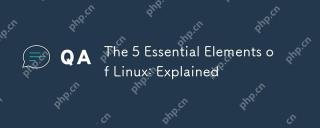
The five core elements of Linux are: 1. Kernel, 2. Command line interface, 3. File system, 4. Package management, 5. Community and open source. Together, these elements define the nature and functionality of Linux.
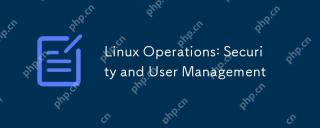
Linux user management and security can be achieved through the following steps: 1. Create users and groups, using commands such as sudouseradd-m-gdevelopers-s/bin/bashjohn. 2. Bulkly create users and set password policies, using the for loop and chpasswd commands. 3. Check and fix common errors, home directory and shell settings. 4. Implement best practices such as strong cryptographic policies, regular audits and the principle of minimum authority. 5. Optimize performance, use sudo and adjust PAM module configuration. Through these methods, users can be effectively managed and system security can be improved.
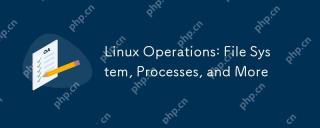
The core operations of Linux file system and process management include file system management and process control. 1) File system operations include creating, deleting, copying and moving files or directories, using commands such as mkdir, rmdir, cp and mv. 2) Process management involves starting, monitoring and killing processes, using commands such as ./my_script.sh&, top and kill.
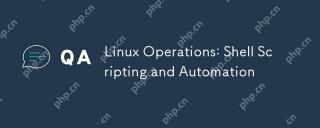
Shell scripts are powerful tools for automated execution of commands in Linux systems. 1) The shell script executes commands line by line through the interpreter to process variable substitution and conditional judgment. 2) The basic usage includes backup operations, such as using the tar command to back up the directory. 3) Advanced usage involves the use of functions and case statements to manage services. 4) Debugging skills include using set-x to enable debugging mode and set-e to exit when the command fails. 5) Performance optimization is recommended to avoid subshells, use arrays and optimization loops.
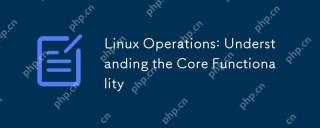
Linux is a Unix-based multi-user, multi-tasking operating system that emphasizes simplicity, modularity and openness. Its core functions include: file system: organized in a tree structure, supports multiple file systems such as ext4, XFS, Btrfs, and use df-T to view file system types. Process management: View the process through the ps command, manage the process using PID, involving priority settings and signal processing. Network configuration: Flexible setting of IP addresses and managing network services, and use sudoipaddradd to configure IP. These features are applied in real-life operations through basic commands and advanced script automation, improving efficiency and reducing errors.
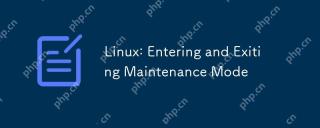
The methods to enter Linux maintenance mode include: 1. Edit the GRUB configuration file, add "single" or "1" parameters and update the GRUB configuration; 2. Edit the startup parameters in the GRUB menu, add "single" or "1". Exit maintenance mode only requires restarting the system. With these steps, you can quickly enter maintenance mode when needed and exit safely, ensuring system stability and security.
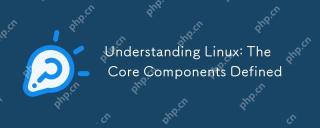
The core components of Linux include kernel, shell, file system, process management and memory management. 1) Kernel management system resources, 2) shell provides user interaction interface, 3) file system supports multiple formats, 4) Process management is implemented through system calls such as fork, and 5) memory management uses virtual memory technology.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
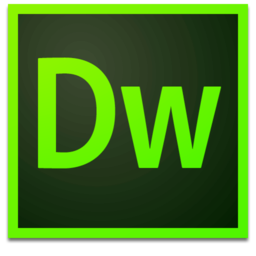
Dreamweaver Mac version
Visual web development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
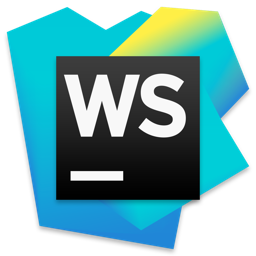
WebStorm Mac version
Useful JavaScript development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
