How to check if a certain value exists in an array in PHP
php editor Baicao teaches you how to check whether a certain value exists in an array. In PHP, you can use the in_array() function to determine whether an array contains a specified value. This function accepts two parameters, the first parameter is the value to be found, and the second parameter is the array to be found. Returns true if the specified value is found, false otherwise. Using this function can quickly and easily check whether a certain value exists in the array, making your code more efficient and concise.
How to check whether a certain value exists in an array in PHP
In php, checking whether a value exists in an array is a common task. There are several ways to achieve this:
1. Use in_array() function
grammar:
in_array($value, $array, $strict = false)
- $value: The value to find.
- $array: The array to search.
- $strict (optional): Specify whether to perform strict comparison (case and type sensitive).
Example:
$arr = array("apple", "banana", "cherry"); // Check if "banana" exists in the array if (in_array("banana", $arr)) { echo "exist"; } else { echo "does not exist"; }
2. Use array_key_exists() function
grammar:
array_key_exists($key, $array)
- $key: The key to search for.
- $array: The array to search.
Example:
$arr = array("fruit" => "apple", "color" => "red"); // Check if the "fruit" key exists in the array if (array_key_exists("fruit", $arr)) { echo "exist"; } else { echo "does not exist"; }
3. Use isset() function
grammar:
isset($array[$key])
- $array: The array to search.
- $key: The key to search for.
Example:
$arr = array("fruit" => "apple", "color" => "red"); // Check if the "fruit" key exists in the array and has been assigned a value if (isset($arr["fruit"])) { echo "exist"; } else { echo "does not exist"; }
Choose the appropriate method
Which method to choose depends on the specific situation:
- in_array(): Case and type sensitive when values need to be compared.
- array_key_exists(): When it is necessary to check whether a specific key exists.
- isset(): When it is necessary to check whether the key exists and has been assigned a value.
Precautions
- These methods distinguish variable types. If you want to do a type-insensitive comparison, you can use the === or !== operators.
- For large arrays, in_array() may be slower than array_key_exists() and isset().
The above is the detailed content of How to check if a certain value exists in an array in PHP. For more information, please follow other related articles on the PHP Chinese website!
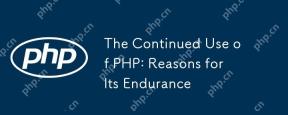
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
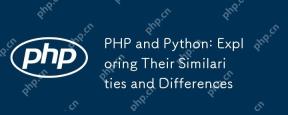
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
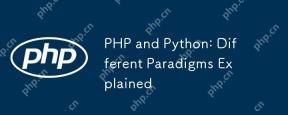
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
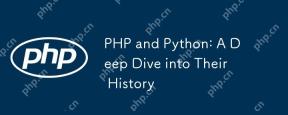
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
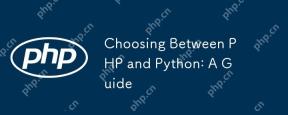
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
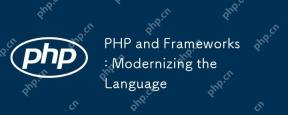
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
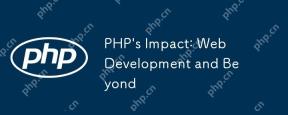
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
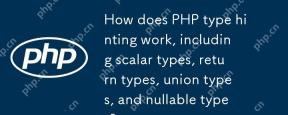
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
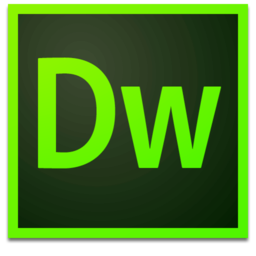
Dreamweaver Mac version
Visual web development tools
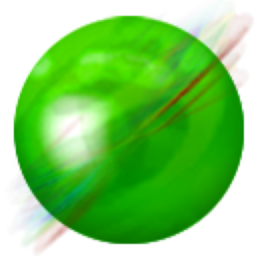
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
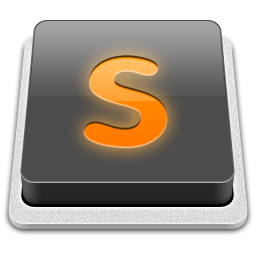
SublimeText3 Mac version
God-level code editing software (SublimeText3)