How to parse string into multiple variables in PHP
php editor Baicao introduces you how to parse a string into multiple variables. In PHP, you can use the explode() function to split a string into an array according to the specified delimiter, and then use the list() function to assign the array elements to multiple variables in sequence. In addition, you can also use the parse_str() function to parse the string into variables. This function will assign the key-value pairs in the string to the corresponding variables. These methods can help you quickly and efficiently handle situations where you need to parse strings and improve programming efficiency.
PHP parses string into multiple variables
Introduction In php, parsing a string into multiple variables is a common need. This helps to extract specific data from a string and store it in a separate variable. There are several ways to accomplish this, such as using string manipulation functions, regular expressions, and third-party libraries.
Method 1: Use string manipulation functions PHP provides several string manipulation functions that can be used to parse strings into multiple variables. For example:
- explode(): Split the string into an array according to the specified delimiter.
- strtok(): Get the tokens in the string one by one and separate them using the specified delimiter.
- str_getcsv(): Parses a string into an array of values separated by commas, semicolons, or tabs.
Example:
<?php $str = "John Doe,30,male"; $arr = explode(",", $str); $name = $arr[0]; $age= $arr[1]; $gender = $arr[2];
Method 2: Use regular expressions
Regular expressions (regex) are a powerful pattern matching tool that can be used to extract specific data from strings. To parse a string into multiple variables using regular expressions, you can use the preg_match_all()
function.
Example:
<?php $str = "John Doe,30,male"; $pattern = "/(w ),s*(d ),s*(w )/"; preg_match_all($pattern, $str, $matches); $name = $matches[1][0]; $age= $matches[2][0]; $gender = $matches[3][0];
Method 3: Using third-party libraries For more complex or customized string parsing needs, you can use third-party libraries, such as:
- Laravel String: Provides powerful string manipulation functions, including string parsing.
- Str: A simple string parsing library that provides a variety of parsing options.
- PHP-Str: A comprehensive string processing library, including string parsing functions.
Example (using Laravel String):
<?php use IlluminateSupportStr; $str = "John Doe,30,male"; $arr = Str::of($str)->explode(","); $name = $arr[0]; $age= $arr[1]; $gender = $arr[2];
Method of choosing Choosing the parsing method to use depends on the complexity of the string, the data type required, and the specific requirements of your project. For simple strings, you can use string manipulation functions. For more complex strings or when custom parsing functionality is required, regular expressions or third-party libraries can be used.
Best Practices
- Use descriptive variable names to clearly represent the extracted data.
- Consider using type casting to ensure variables have the correct type.
- Error handling of strings that may be empty or invalid.
- Optimize Regular expressions to improve performance.
- When using third-party libraries, follow the best practices of the library documentation.
The above is the detailed content of How to parse string into multiple variables in PHP. For more information, please follow other related articles on the PHP Chinese website!
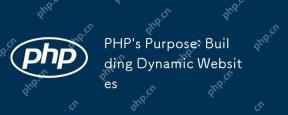
PHP is used to build dynamic websites, and its core functions include: 1. Generate dynamic content and generate web pages in real time by connecting with the database; 2. Process user interaction and form submissions, verify inputs and respond to operations; 3. Manage sessions and user authentication to provide a personalized experience; 4. Optimize performance and follow best practices to improve website efficiency and security.

PHP uses MySQLi and PDO extensions to interact in database operations and server-side logic processing, and processes server-side logic through functions such as session management. 1) Use MySQLi or PDO to connect to the database and execute SQL queries. 2) Handle HTTP requests and user status through session management and other functions. 3) Use transactions to ensure the atomicity of database operations. 4) Prevent SQL injection, use exception handling and closing connections for debugging. 5) Optimize performance through indexing and cache, write highly readable code and perform error handling.
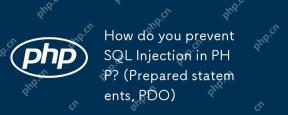
Using preprocessing statements and PDO in PHP can effectively prevent SQL injection attacks. 1) Use PDO to connect to the database and set the error mode. 2) Create preprocessing statements through the prepare method and pass data using placeholders and execute methods. 3) Process query results and ensure the security and performance of the code.
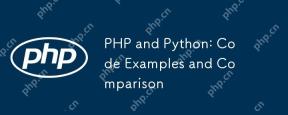
PHP and Python have their own advantages and disadvantages, and the choice depends on project needs and personal preferences. 1.PHP is suitable for rapid development and maintenance of large-scale web applications. 2. Python dominates the field of data science and machine learning.
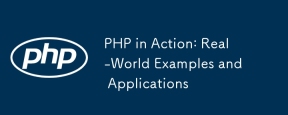
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
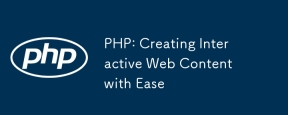
PHP makes it easy to create interactive web content. 1) Dynamically generate content by embedding HTML and display it in real time based on user input or database data. 2) Process form submission and generate dynamic output to ensure that htmlspecialchars is used to prevent XSS. 3) Use MySQL to create a user registration system, and use password_hash and preprocessing statements to enhance security. Mastering these techniques will improve the efficiency of web development.
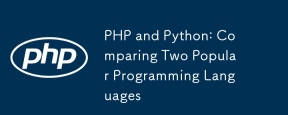
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
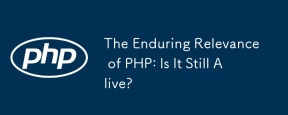
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
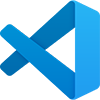
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Chinese version
Chinese version, very easy to use
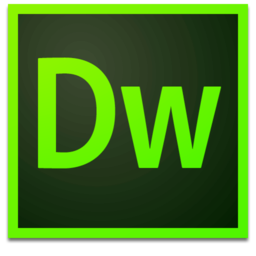
Dreamweaver Mac version
Visual web development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Atom editor mac version download
The most popular open source editor