Golang is an open source programming language developed and launched by Google and is widely used in the development of large systems. But in addition to these large-scale projects, Golang has also shown strong application capabilities in the field of gadget development. This article will explore how Golang assists the development and application of gadgets and provide specific code examples.
1. Introduction
Golang is a statically typed, compiled language with an efficient concurrent programming model and excellent performance. This makes Golang very suitable for developing small tools, such as command line tools, batch programs, etc. Golang's robustness and simplicity make it popular in the field of gadget development. Next, we will use practical examples to illustrate how Golang facilitates the development of gadgets.
2. File operation tools
File operation is one of the common functions in gadgets. Taking a simple file copy tool as an example, we can use Golang's standard library to implement it.
package main import ( "fmt" "io" "os" ) func main() { sourceFile := "source.txt" destinationFile := "destination.txt" source, err := os.Open(sourceFile) if err != nil { fmt.Println("Open source file error:", err) return } defer source.Close() destination, err := os.Create(destinationFile) if err != nil { fmt.Println("Create destination file error:", err) return } defer destination.Close() _, err = io.Copy(destination, source) if err != nil { fmt.Println("Copy file error:", err) return } fmt.Println("File copied successfully!") }
The above code implements a simple file copy tool. We open the source and target files through the Open and Create functions in the os package, and then use the Copy function in the io package to copy the source file contents to the target file. Finally, we output "File copied successfully!" to indicate whether the copy operation was successful.
3. Network request tool
Another common gadget function is network request. Here is a sample code for a simple HTTP GET request tool:
package main import ( "fmt" "io/ioutil" "net/http" ) func main() { url := "https://jsonplaceholder.typicode.com/posts/1" response, err := http.Get(url) if err != nil { fmt.Println("HTTP GET error:", err) return } defer response.Body.Close() body, err := ioutil.ReadAll(response.Body) if err != nil { fmt.Println("Read response error:", err) return } fmt.Println("Response:", string(body)) }
The above code sends an HTTP GET request through the http package and outputs the response content. Through this small tool, we can easily obtain data from the remote server.
4. Command line tools
Golang also provides a third-party library cobra, which can help us develop command line tools more conveniently. Here is a sample code using the cobra library:
package main import ( "fmt" "github.com/spf13/cobra" ) func main() { var rootCmd = &cobra.Command{ Use: "mytool", Short: "A simple CLI tool", Long: `A simple command line tool in Golang`, Run: func(cmd *cobra.Command, args []string) { fmt.Println("Hello, this is my tool!") }, } if err := rootCmd.Execute(); err != nil { fmt.Println(err) } }
In this example, we define a command line tool named "mytool". When the user executes the tool, "Hello, this is my tool!" will be output. Through the cobra library, we can easily define commands, subcommands, parameters and help information, making the development of command line tools simpler and more elegant.
5. Summary
This article explores how Golang can help the development and application of gadgets through specific code examples. From file operations to network requests to command line tools, Golang demonstrates its simplicity, efficiency and power. It is these features that make Golang one of the preferred languages for gadget development. I hope this article will be helpful to readers who want to learn Golang gadget development.
The above is the detailed content of Discuss how Golang helps the development and application of gadgets. For more information, please follow other related articles on the PHP Chinese website!
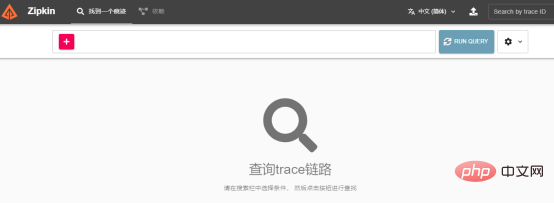
译者 | 李睿审校 | 孙淑娟随着Python越来越受欢迎,其局限性也越来越明显。一方面,编写Python应用程序并将其分发给没有安装Python的人员可能非常困难。解决这一问题的最常见方法是将程序与其所有支持库和文件以及Python运行时打包在一起。有一些工具可以做到这一点,例如PyInstaller,但它们需要大量的缓存才能正常工作。更重要的是,通常可以从生成的包中提取Python程序的源代码。在某些情况下,这会破坏交易。第三方项目Nuitka提供了一个激进的解决方案。它将Python程序编
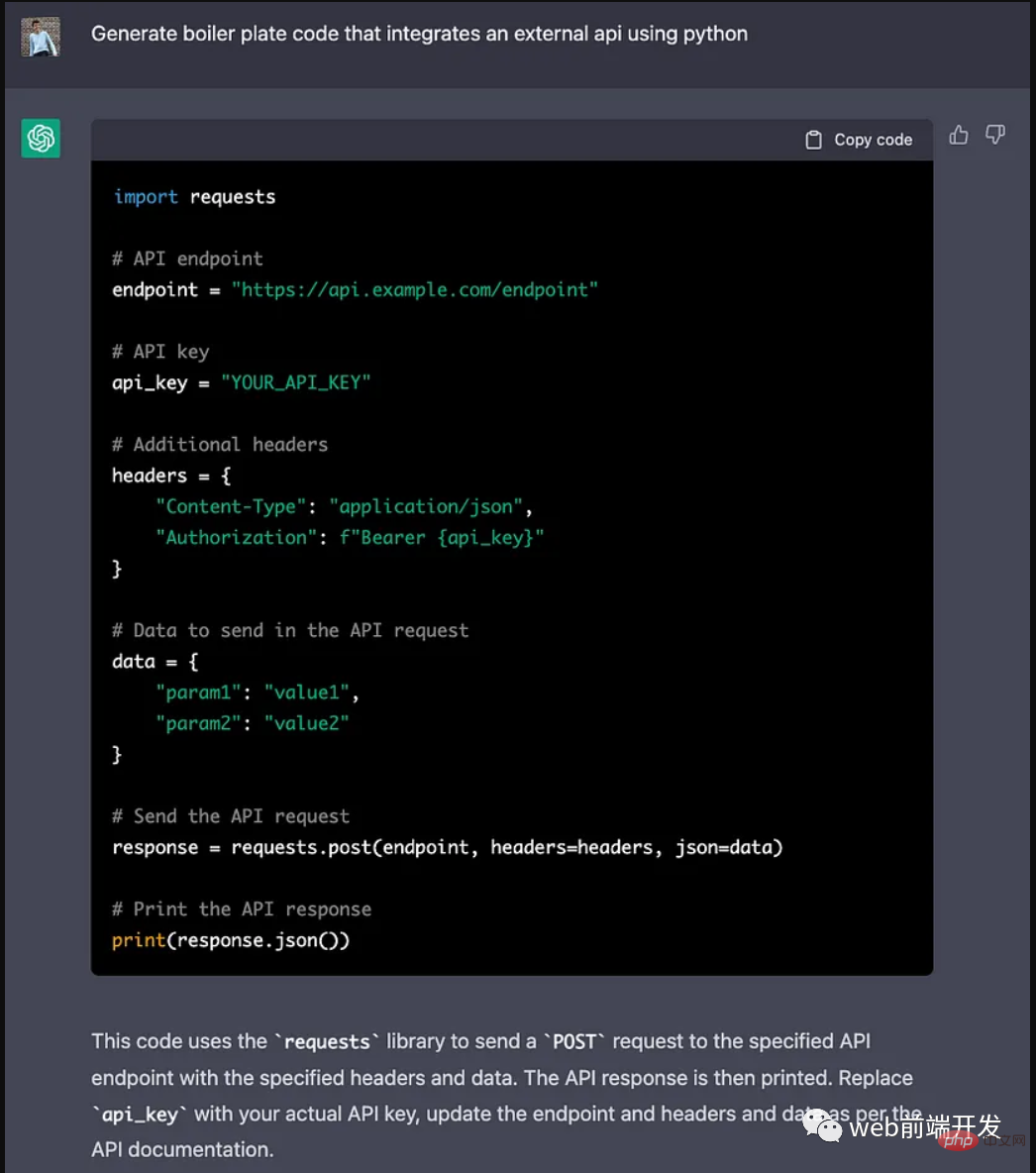
ChatGPT 目前彻底改变了开发代码的方式,然而,大多数软件开发人员和数据专家仍然没有使用 ChatGPT 来改进和简化他们的工作。这就是为什么我在这里概述 5 个不同的功能,以提高我们的日常工作速度和质量。我们可以在日常工作中使用它们。现在,我们一起来了解一下吧。注意:切勿在 ChatGPT 中使用关键代码或信息。01.生成项目代码的框架从头开始构建新项目时,ChatGPT 是我的秘密武器。只需几个提示,它就可以生成我需要的代码框架,包括我选择的技术、框架和版本。它不仅为我节省了至少一个小时
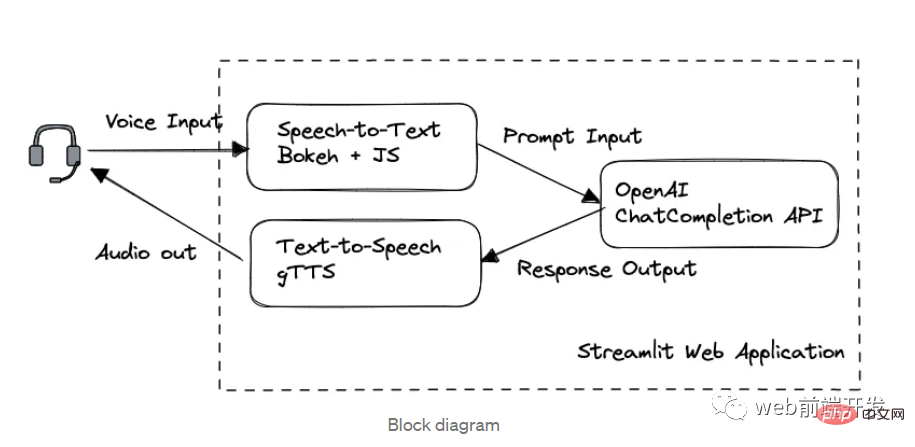
今天这篇文章的重点是使用 ChatGPT API 创建私人语音 Chatbot Web 应用程序。目的是探索和发现人工智能的更多潜在用例和商业机会。我将逐步指导您完成开发过程,以确保您理解并可以复制自己的过程。为什么需要不是每个人都欢迎基于打字的服务,想象一下仍在学习写作技巧的孩子或无法在屏幕上正确看到单词的老年人。基于语音的 AI Chatbot 是解决这个问题的方法,就像它如何帮助我的孩子要求他的语音 Chatbot 给他读睡前故事一样。鉴于现有可用的助手选项,例如,苹果的 Siri 和亚马
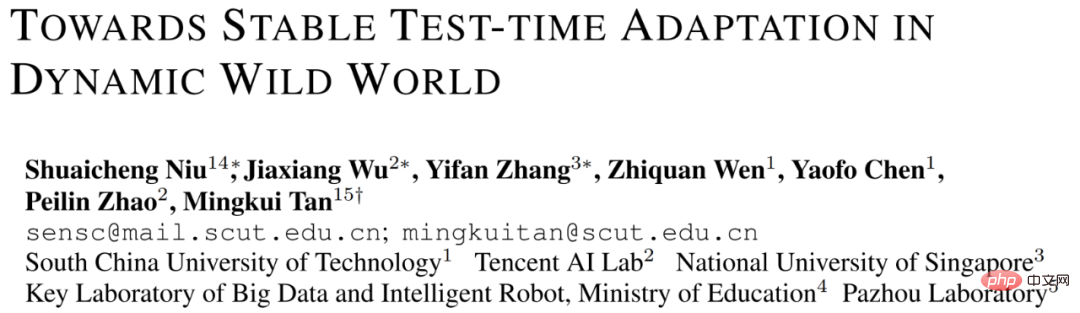
测试时自适应(Test-TimeAdaptation,TTA)方法在测试阶段指导模型进行快速无监督/自监督学习,是当前用于提升深度模型分布外泛化能力的一种强有效工具。然而在动态开放场景中,稳定性不足仍是现有TTA方法的一大短板,严重阻碍了其实际部署。为此,来自华南理工大学、腾讯AILab及新加坡国立大学的研究团队,从统一的角度对现有TTA方法在动态场景下不稳定原因进行分析,指出依赖于Batch的归一化层是导致不稳定的关键原因之一,另外测试数据流中某些具有噪声/大规模梯度的样本
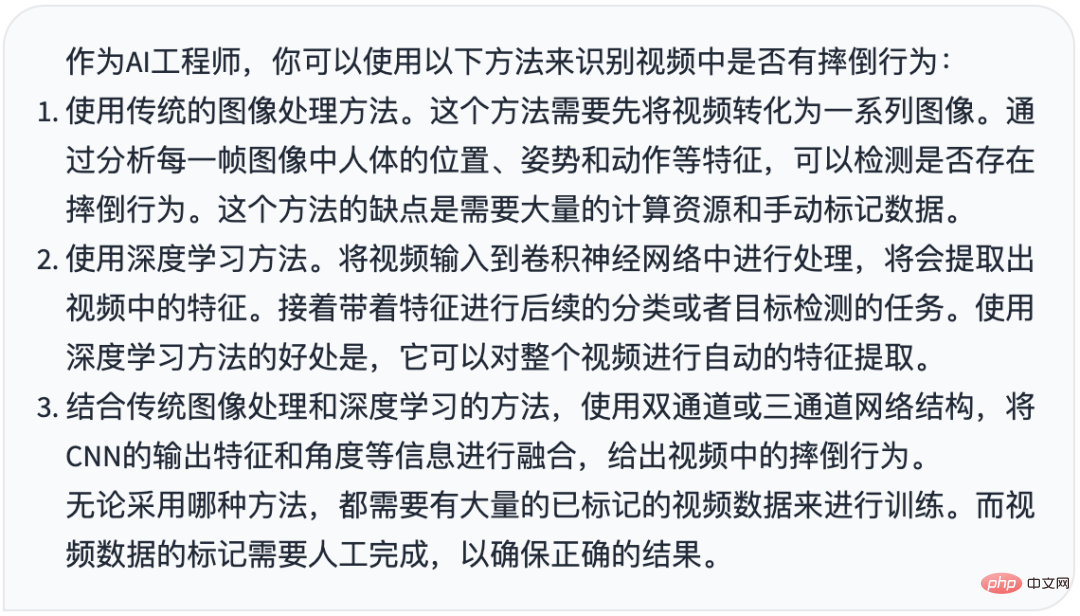
哈喽,大家好。之前给大家分享过摔倒识别、打架识别,今天以摔倒识别为例,我们看看能不能完全交给ChatGPT来做。让ChatGPT来做这件事,最核心的是如何向ChatGPT提问,把问题一股脑的直接丢给ChatGPT,如:用 Python 写个摔倒检测代码 是不可取的, 而是要像挤牙膏一样,一点一点引导ChatGPT得到准确的答案,从而才能真正让ChatGPT提高我们解决问题的效率。今天分享的摔倒识别案例,与ChatGPT对话的思路清晰,代码可用度高,按照GPT返回的结果完全可以开
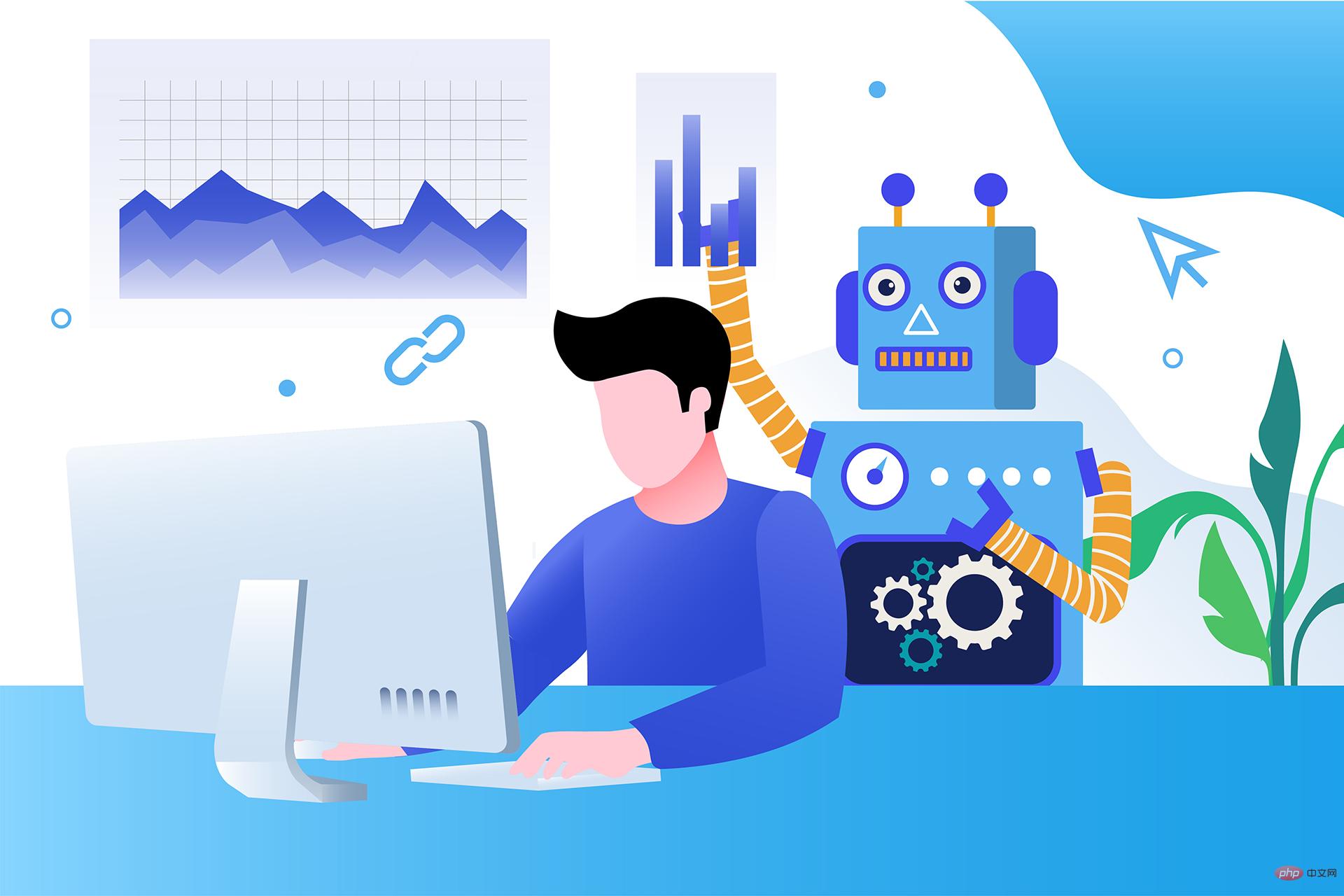
自 2020 年以来,内容开发领域已经感受到人工智能工具的存在。1.Jasper AI网址:https://www.jasper.ai在可用的 AI 文案写作工具中,Jasper 作为那些寻求通过内容生成赚钱的人来讲,它是经济实惠且高效的选择之一。该工具精通短格式和长格式内容均能完成。Jasper 拥有一系列功能,包括无需切换到模板即可快速生成内容的命令、用于创建文章的高效长格式编辑器,以及包含有助于创建各种类型内容的向导的内容工作流,例如,博客文章、销售文案和重写。Jasper Chat 是该
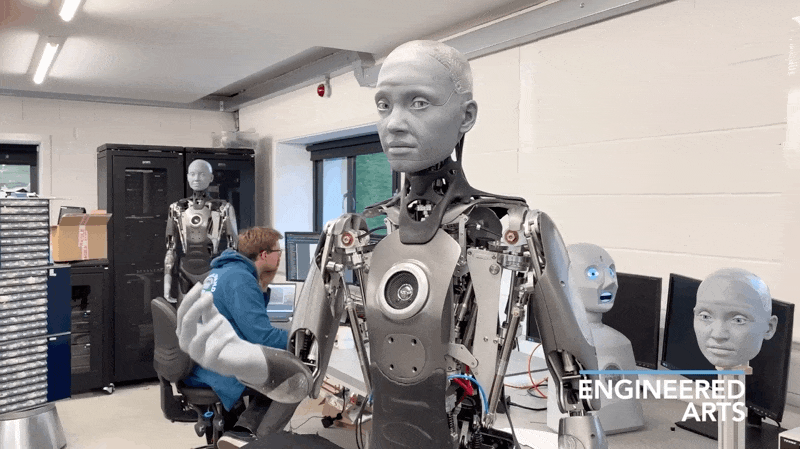
1970年,机器人专家森政弘(MasahiroMori)首次描述了「恐怖谷」的影响,这一概念对机器人领域产生了巨大影响。「恐怖谷」效应描述了当人类看到类似人类的物体,特别是机器人时所表现出的积极和消极反应。恐怖谷效应理论认为,机器人的外观和动作越像人,我们对它的同理心就越强。然而,在某些时候,机器人或虚拟人物变得过于逼真,但又不那么像人时,我们大脑的视觉处理系统就会被混淆。最终,我们会深深地陷入一种对机器人非常消极的情绪状态里。森政弘的假设指出:由于机器人与人类在外表、动作上相似,所以人类亦会对
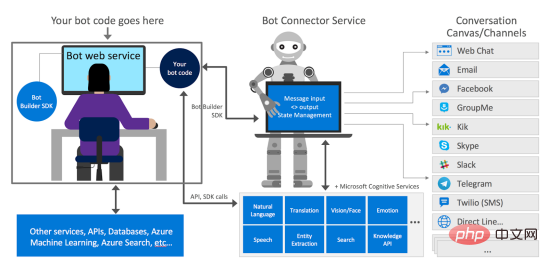
译者 | 李睿审校 | 孙淑娟信使、网络服务和其他软件都离不开机器人(bot)。而在软件开发和应用中,机器人是一种应用程序,旨在自动执行(或根据预设脚本执行)响应用户请求创建的操作。在本文中, NIX United公司的.NET开发人员Daniil Mikhov介绍了使用微软Azure Bot Services创建聊天机器人的一个例子。本文将对想要使用该服务开发聊天机器人的开发人员有所帮助。 为什么使用Azure Bot Services? 在Azure Bot Services上开发聊


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
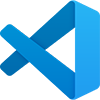
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
