An in-depth analysis of the generic nature of interfaces in Golang
In-depth analysis of the generic nature of interfaces in Golang
In the field of programming, generics is an important concept, which allows programmers to write more flexibly and Universal code. However, unlike some other programming languages, Golang does not provide native generic support. This creates some challenges for programmers, especially when working with interfaces. This article will deeply analyze the generic nature of interfaces in Golang and use specific code examples to help readers better understand.
1. Interface in Golang
In Golang, an interface is an abstract data type that defines a collection of methods. Any type that implements these methods can be called an implementation type of the interface. Interfaces provide a flexible way to implement polymorphism, making code more versatile and extensible.
For example, we define a simple interface Animal
:
type Animal interface { Speak() string }
Any type that implements the Speak()
method in the Animal
interface can be regarded as an implementation type of the Animal
interface. This means that we can define various types of animals, such as dogs, cats, etc., and they can all implement the Animal
interface:
type Dog struct{} func (d Dog) Speak() string { return "woof woof woof" } type Cat struct{} func (c Cat) Speak() string { return "meow meow meow" }
Next, we can implement dynamic calling through the interface:
func LetAnimalSpeak(animal Animal) { fmt.Println(animal.Speak()) } func main() { dog := Dog{} cat := Cat{} LetAnimalSpeak(dog) LetAnimalSpeak(cat) }
In the above code, we define the LetAnimalSpeak
function, which accepts a parameter of Animal
interface type, and then calls the parameter's Speak()
method. This way we can dynamically make sounds for different types of animals.
2. Generic nature of interfaces
Although Golang does not have native generic support, through interfaces, we can realize generic characteristics to a certain extent. Interfaces allow us to hide specific implementation types, thereby achieving abstraction and generalization of code.
Next, let’s take a more complex example to explore the generic nature of interfaces. Suppose we have a requirement for a generic stack structure. We need to implement a general stack structure that can store any type of data.
First, we define a generic interface Stack
:
type Stack interface { Push(interface{}) Pop() interface{} }
Then, we can define a specific type of stack structure GenericStack
, which implements the Stack
interface:
type GenericStack struct { data[]interface{} } func (s *GenericStack) Push(item interface{}) { s.data = append(s.data, item) } func (s *GenericStack) Pop() interface{} { if len(s.data) == 0 { return nil } lastIndex := len(s.data) - 1 item := s.data[lastIndex] s.data = s.data[:lastIndex] return item }
Next, we can use this generic stack structure to store different types of data:
func main() { stack := &GenericStack{} stack.Push(1) stack.Push("hello") stack.Push(true) fmt.Println(stack.Pop()) // true fmt.Println(stack.Pop()) // hello fmt.Println(stack.Pop()) // 1 }
In the above code, we define a generic stack structureGenericStack
, which can store any type of data. By defining the interface and specific implementation, we successfully implemented a universal stack structure and made it have generic characteristics.
Summary
This article deeply analyzes the generic nature of interfaces in Golang, and helps readers understand the flexibility and versatility of interfaces through specific code examples. Although Golang does not have native generic support, through interfaces, we can implement generic-like features and improve code reusability and scalability. I hope this article will help readers use interfaces and implement generic code in Golang.
The word count of this article is approximately 1043 words.
The above is the detailed content of An in-depth analysis of the generic nature of interfaces in Golang. For more information, please follow other related articles on the PHP Chinese website!
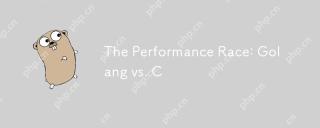
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
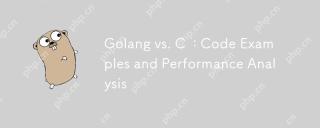
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
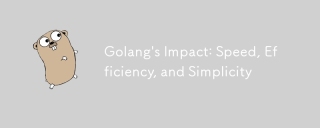
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
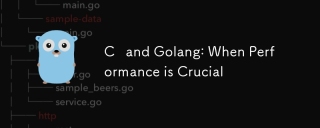
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
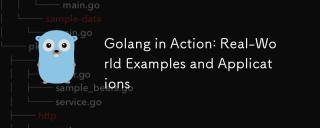
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
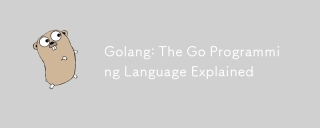
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
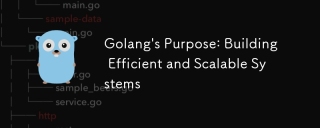
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
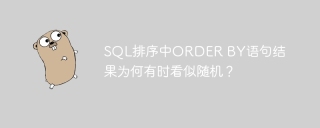
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
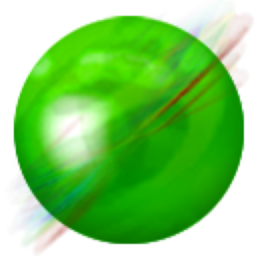
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
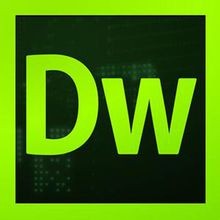
Dreamweaver CS6
Visual web development tools
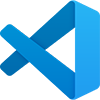
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
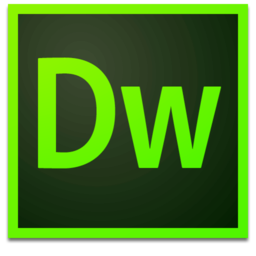
Dreamweaver Mac version
Visual web development tools