PHP Form Processing: The Ultimate Guide from Zero to Mastery
Form creation
To create a PHP form, you can use the <f class="keylink">ORM></f>
element:
<form method="post" action="process.php"> <input type="text" name="username"> <input type="passWord" name="password"> <input type="submit" value="Submit"> </form>
-
The
-
method
attribute specifies the form submission method, and thepost
method is more safer because it hides the data in the Http request.
The -
action
attribute specifies the PHP script that handles the form.
form validation Validating user input is critical to ensuring data integrity and preventing malicious attacks. PHP provides built-in functions and classes for verification:
-
filter_var()
: Used to filter and validate variables such as emails, URLs, and integers. -
preg_match()
: Used to verify strings using regular expressions. -
<strong class="keylink">html</strong>specialchars()
: Used to escape HTML special characters to prevent cross-site scripting (XSS) attacks.
<?php if (empty($_POST["username"]) || empty($_POST["password"])) { echo "Missing required fields"; exit; } if (!filter_var($_POST["email"], FILTER_VALIDATE_EMAIL)) { echo "Invalid email address"; exit; } ?>
Form processing After validation, the data submitted by the form can be processed. You can use the following steps:
-
Get form data: Use
$_POST
or$_GET
Array to access the submitted data. - Process the data: Perform any necessary operations as needed, such as storing to a database or sending an email.
-
Redirect: Use the
header()
function to redirect the user to a new page, such as a success or error page.
<?php // Get form data $username = $_POST["username"]; $password = $_POST["password"]; // Data processing // ... // Redirect to success page header("Location: success.php");
Form Security Security is of the utmost importance when working with forms. The following measures should be taken:
- Use HTTPS: Use an encrypted connection to transmit data to prevent interception.
-
Prevent XSS attacks: Use the
htmlspecialchars()
function to escape user input. - Prevent CSRF attacks: Use tokens or asymmetric encryption.
- Verify the HTTP Referer header: Make sure the request comes from the expected source.
Best Practices The following best practices can help improve form processing:
- Use consistent validation rules: Develop and always follow clear validation rules.
- Provide a clear error message: Provide the user with a detailed error message explaining what the error is and how to fix it.
- Avoid duplicate submissions: Use tokens or other mechanisms to prevent users from repeatedly submitting the same form.
- Optimize performance: Separate form processing logic from HTML code and use database indexing and caching technologies.
Advanced Technology PHP provides more advanced form processing technology:
-
File upload: Use the
$_FILES
array to process uploaded files. - AJAX Forms: Dynamic form validation and processing using javascript and PHP.
- Form library: Use third-party libraries (such as FormHelper) to simplify form processing.
Summarize Mastering PHP form handling is critical to creating interactive and secure WEB applications. By following best practices and leveraging advanced technologies, developers can easily handle user input, ensuring data integrity and improving the user experience.
The above is the detailed content of PHP Form Processing: The Ultimate Guide from Zero to Mastery. For more information, please follow other related articles on the PHP Chinese website!
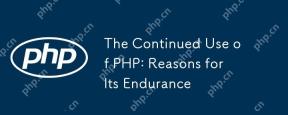
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
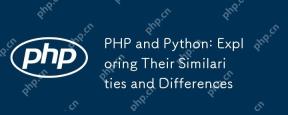
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
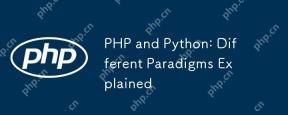
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
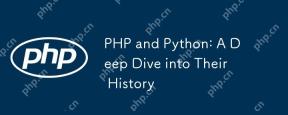
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
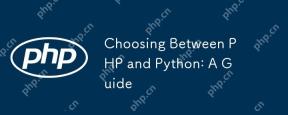
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
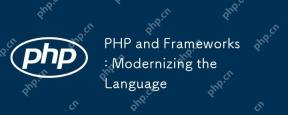
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
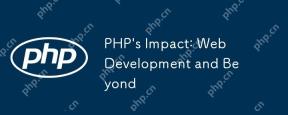
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
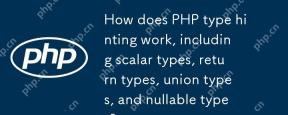
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
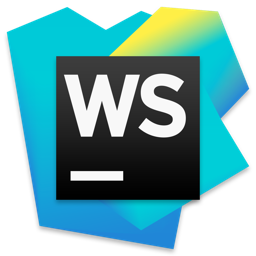
WebStorm Mac version
Useful JavaScript development tools
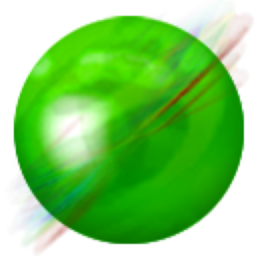
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.