


Comparison of similarities and differences between C language and Go programming language
Comparison of similarities and differences between C language and Go programming language
With the continuous development of computer science, various programming languages emerge in endlessly, of which C language and Go programming language are the two Two widely used programming languages, each with unique characteristics and advantages. This article will compare C language and Go programming language in terms of syntax structure, concurrent programming, performance, etc., and provide specific code examples.
- Comparison of syntax structures
C language is a procedural programming language with relatively simple and intuitive syntax, suitable for system-level programming and embedded development. The code example in C language is as follows:
#include <stdio.h> int main() { printf("Hello, World! "); return 0; }
The Go programming language is a statically typed, concurrency-supported programming language with concise syntax, support for garbage collection, and is suitable for building distributed systems and network services. The code example of Go language is as follows:
package main import "fmt" func main() { fmt.Println("Hello, World!") }
It can be seen that the Go language is more concise in grammatical structure than the C language, and supports package management, automatic memory management and other features, making its programming more efficient.
- Concurrent programming comparison
In terms of concurrent programming, the Go programming language has natural concurrency support, and concurrent programming can be easily achieved through goroutine and channel. The following is an example of concurrent programming in Go language:
package main import ( "fmt" "time" ) func worker(id int, jobs <-chan int, results chan<- int) { for j := range jobs { fmt.Println("Worker", id, "processing job", j) time.Sleep(time.Second) results <- j * 2 } } func main() { jobs := make(chan int, 5) results := make(chan int, 5) for w := 1; w <= 3; w { go worker(w, jobs, results) } for j := 1; j <= 5; j { jobs <- j } close(jobs) for a := 1; a <= 5; a { <-results } }
In contrast, C language does not directly support concurrent programming and needs to be implemented through the thread mechanism or library provided by the operating system. The following is an example of concurrent programming using the POSIX thread library in C language:
#include <stdio.h> #include <pthread.h> void *worker(void *arg) { int *job = (int *)arg; printf("Worker processing job %d ", *job); // Simulate processing sleep(1); return NULL; } int main() { pthread_t thread; int job = 1; pthread_create(&thread, NULL, worker, &job); // Wait for thread to finish pthread_join(thread, NULL); return 0; }
- Performance comparison
In terms of performance, C language is generally considered to be one of the best performing programming languages. It natively supports pointer operations and can perform more precise operations. Control memory and hardware resources. The following is an example of calculating the Fibonacci sequence in C language:
#include <stdio.h> int fibonacci(int n) { if (n <= 1) { return n; } return fibonacci(n-1) fibonacci(n-2); } int main() { int n = 10; int result = fibonacci(n); printf("Fibonacci(%d) = %d ", n, result); return 0; }
In contrast, although the performance of the Go programming language is not as good as that of the C language, it performs well in practical applications, especially in concurrent programming and network programming. The following is an example of calculating the Fibonacci sequence in Go language:
package main import "fmt" func fibonacci(n int) int { if n <= 1 { return n } return fibonacci(n-1) fibonacci(n-2) } func main() { n := 10 result := fibonacci(n) fmt.Printf("Fibonacci(%d) = %d ", n, result) }
To sum up, C language and Go programming language have their own advantages and characteristics in terms of syntax structure, concurrent programming and performance. Developers can choose appropriate programming languages for development based on specific application scenarios and needs. I hope this article can help readers better understand the similarities and differences between the C language and the Go programming language.
The above is the detailed content of Comparison of similarities and differences between C language and Go programming language. For more information, please follow other related articles on the PHP Chinese website!
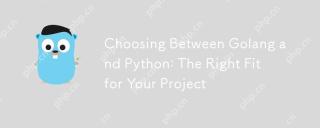
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
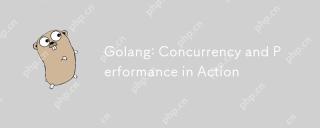
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
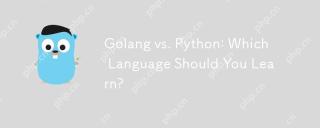
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
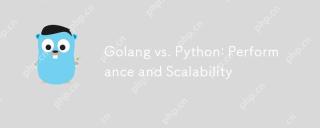
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
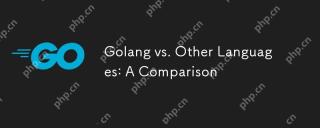
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
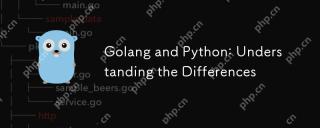
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
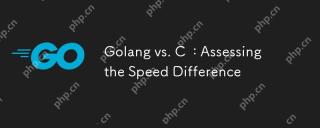
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
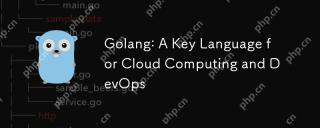
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
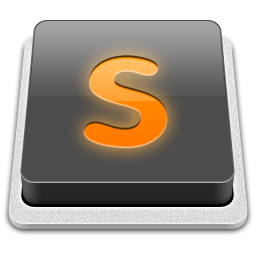
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.