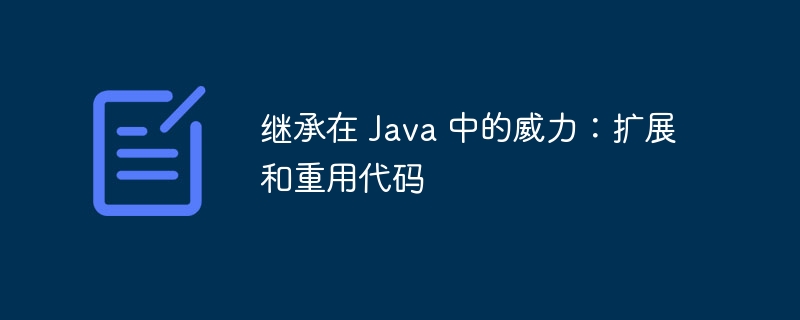
In Java programming, inheritance is a powerful tool for extending and reusing code. Through inheritance, a class can inherit properties and methods from another class, thereby enabling code reuse and extension. PHP editor Zimo will lead you to an in-depth discussion of the power of inheritance in Java and explain how to use inheritance correctly to improve the maintainability and flexibility of the code. Let's explore the secrets of inheritance and improve your Java programming skills!
- Through inheritance, a derived class (or subclass) can access and use all member variables and methods in the base class.
- Derived classes can extend these members as needed, adding new variables and methods to create more specific implementations.
- This avoids duplication of common code in derived classes, thus simplifying maintenance and updates.
Code reuse:
- Inheritance promotes code reuse by allowing derived classes to use the public interface defined in the base class.
- This reduces code redundancy and improves the maintainability and scalability of the application.
- Changes to code in the base class are automatically reflected in all derived classes, simplifying bug fixes and feature enhancements.
Example:
Consider an abstract class Shape that defines basic geometric properties and methods for all shapes. We can derive different shapes from Shape such as Circle, Square and Triangle.
// Shape class
abstract class Shape {
private int x;
private int y;
public Shape(int x, int y) {
this.x = x;
this.y = y;
}
public abstract double getArea();
}
// Circle class
class Circle extends Shape {
private double radius;
public Circle(int x, int y, double radius) {
super(x, y);
this.radius = radius;
}
@Override
public double getArea() {
return Math.PI * radius * radius;
}
}
Through inheritance, the Circle class can access and use the x and y coordinates defined in the Shape. It can also provide its own unique area calculation by overriding the getArea() method.
benefit:
- Code extension: The Circle class can easily extend the Shape class to add radius variables and methods for calculating area.
- Code reuse: The Circle class reuses the coordinate management code defined in the Shape class to avoid duplication.
- Simplified maintenance: If the coordinate management code in the Shape class needs to be changed, the Circle class will automatically inherit these changes, simplifying maintenance.
limitation:
-
Multiple inheritance: Java does not support multiple inheritance (inheriting from multiple base classes), which may limit code reuse in certain situations.
-
Coupling: Derived classes are tightly coupled with base classes, so changes to the base class may affect derived classes.
-
Rigidity: Derived classes cannot modify the implementation of the base class, which may limit flexibility.
Best Practices:
- Use inheritance only when needed and avoid excessive inheritance.
- Prioritize composition over inheritance for better flexibility.
- Use interfaces for loose coupling, allowing classes to implement multiple interfaces without inheriting them.
- Use abstract classes with caution to avoid creating class hierarchies that are difficult to instantiate.
The above is the detailed content of The power of inheritance in Java: extending and reusing code. For more information, please follow other related articles on the PHP Chinese website!