Go language generics analysis: What is the essence of its generics?
Go language generic analysis: What is the essence of its generics?
In recent years, the Go language has been criticized for its lack of generic support, which has become the focus of discussion among many developers. As the Go language community continues to develop and the need for generics grows, the Go language team introduced generics support in version 1.18. This move has triggered widespread discussion and expectations, so what is the essence of Go language generics? This article will analyze and discuss it from the perspective of actual code examples.
First, let’s look at a simple example that shows how the Go language handles variables of different types before the introduction of generics. Suppose we have a function that compares the size of two integers:
func compareInt(a, b int) int { if a > b { return 1 } else if a < b { return -1 } else { return 0 } }
This function can only compare variables of integer type. If we need to compare variables of other types, we need to write similar functions repeatedly, which not only increases the redundancy of the code, but is also not flexible and versatile enough.
After introducing generics, we can define a general comparison function that can compare variables of any type:
func compare[T comparable](a, b T) int { if a > b { return 1 } else if a < b { return -1 } else { return 0 } }
In this example, [T comparable]
indicates that the generic parameter T is a comparable type. In this way, we can use this compare
function to compare variables of any type without having to repeatedly write multiple functions.
In addition to defining generic functions, Go language generics also support generic types and generic interfaces. Let's look at a more complex example showing how to define a generic stack structure:
package main import "fmt" type Stack[T any] struct { data[]T } func (s *Stack[T]) Push(item T) { s.data = append(s.data, item) } func (s *Stack[T]) Pop() T { if len(s.data) == 0 { panic("stack is empty") } item := s.data[len(s.data)-1] s.data = s.data[:len(s.data)-1] return item } func main() { stack := Stack[int]{} stack.Push(1) stack.Push(2) fmt.Println(stack.Pop()) // Output: 2 fmt.Println(stack.Pop()) // Output: 1 }
In this example, we define a Stack
structure, in which the element type T
is any type. By defining the generic methods Push
and Pop
, we can implement a general stack structure that can store elements of any type. In the main
function, we demonstrate how to use a generic stack structure to conveniently store and operate different types of data.
In general, the Go language's generics essentially provide a more flexible and versatile solution while maintaining the simplicity and efficiency of the language. Through generics, we can avoid writing redundant code and improve code reusability and maintainability. Although the implementation of generics in Go language has some differences from other languages, it undoubtedly brings broader possibilities to the development of Go language. In future development, with the support of generics, we can handle various complex data structures and algorithms more flexibly, injecting new vitality and creativity into the Go language ecosystem.
The above is the detailed content of Go language generics analysis: What is the essence of its generics?. For more information, please follow other related articles on the PHP Chinese website!
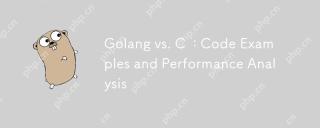
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
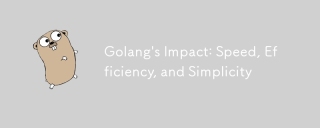
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
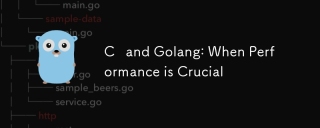
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
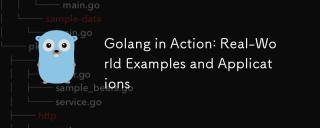
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
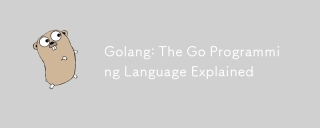
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
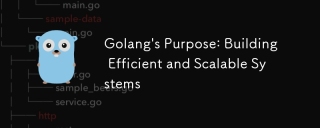
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
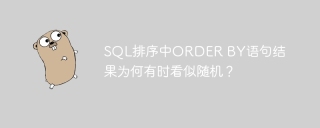
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
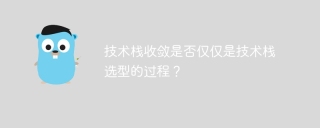
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
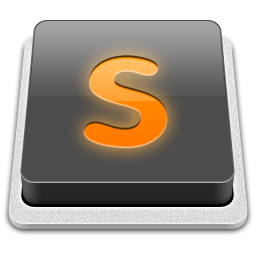
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
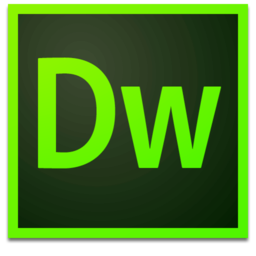
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool