


In-depth understanding of the application scenarios of MySQL stored procedures
In-depth understanding of the application scenarios of MySQL stored procedures
MySQL is a commonly used relational database management system, widely used in various Web applications and enterprise information systems . Stored procedure is an important database object in MySQL. It is a set of pre-compiled SQL statements and data processing logic that can be called and reused multiple times.
The application scenarios of stored procedures are very wide. It can be used to simplify complex data operations, improve database performance, enhance data security, implement business logic encapsulation, etc. Let's use specific code examples to deeply understand the application scenarios of MySQL stored procedures.
- Simplify complex data operations
Stored procedures can encapsulate a series of SQL statements to simplify complex data operations. For example, we can create a stored procedure to calculate the average salary of a certain department:
DELIMITER // CREATE PROCEDURE calculate_avg_salary (IN department_id INT) BEGIN DECLARE avg_salary DECIMAL(10, 2); SELECT AVG(salary) INTO avg_salary FROM employees WHERE department_id = department_id; SELECT avg_salary; END // DELIMITER ;
Then get the average salary of a certain department by calling the stored procedure:
CALL calculate_avg_salary(1);
- Improve the database Performance
Stored procedures can reduce network transmission volume and reduce the compilation time of SQL statements, thereby improving database performance. For example, we can create a stored procedure to insert large amounts of data in batches:
DELIMITER // CREATE PROCEDURE bulk_insert_data () BEGIN DECLARE i INT DEFAULT 1; WHILE i <= 10000 DO INSERT INTO test_table (id, name) VALUES (i, CONCAT('Name ', i)); SET i = i + 1; END WHILE; END // DELIMITER ;
Then insert data in batches by calling the stored procedure:
CALL bulk_insert_data();
- Enhance data security
Stored procedures can limit users' operating permissions on the database, and can implement data verification and filtering through stored procedures. For example, we can create a stored procedure to update the employee's salary while limiting the salary increase to no more than 10%:
DELIMITER // CREATE PROCEDURE update_employee_salary (IN employee_id INT, IN new_salary DECIMAL(10, 2)) BEGIN DECLARE old_salary DECIMAL(10, 2); SELECT salary INTO old_salary FROM employees WHERE id = employee_id; IF new_salary > old_salary * 1.1 THEN SIGNAL SQLSTATE '45000' SET MESSAGE_TEXT = 'Salary increase is too high'; ELSE UPDATE employees SET salary = new_salary WHERE id = employee_id; END IF; END // DELIMITER ;
Then update the employee's salary by calling the stored procedure:
CALL update_employee_salary(1, 5500.00);
- Implement business logic encapsulation
Stored procedures can encapsulate business logic on the database side to improve code reusability and maintainability. For example, we can create a stored procedure to calculate the employee's year-end bonus:
DELIMITER // CREATE PROCEDURE calculate_bonus (IN employee_id INT) BEGIN DECLARE salary DECIMAL(10, 2); DECLARE bonus DECIMAL(10, 2); SELECT salary INTO salary FROM employees WHERE id = employee_id; IF salary > 5000.00 THEN SET bonus = salary * 0.1; ELSE SET bonus = salary * 0.05; END IF; SELECT bonus; END // DELIMITER ;
Then calculate the employee's year-end bonus by calling the stored procedure:
CALL calculate_bonus(1);
In summary, MySQL stored procedures have many advantages , can help us simplify complex data operations, improve database performance, enhance data security, and implement business logic encapsulation, etc. Through the above specific code examples, I hope readers can have a deeper understanding of the application scenarios of MySQL stored procedures and use them flexibly in actual projects.
The above is the detailed content of In-depth understanding of the application scenarios of MySQL stored procedures. For more information, please follow other related articles on the PHP Chinese website!
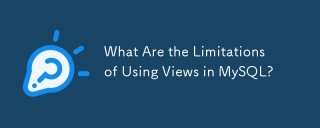
MySQLviewshavelimitations:1)Theydon'tsupportallSQLoperations,restrictingdatamanipulationthroughviewswithjoinsorsubqueries.2)Theycanimpactperformance,especiallywithcomplexqueriesorlargedatasets.3)Viewsdon'tstoredata,potentiallyleadingtooutdatedinforma
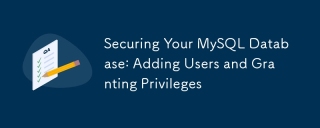
ProperusermanagementinMySQLiscrucialforenhancingsecurityandensuringefficientdatabaseoperation.1)UseCREATEUSERtoaddusers,specifyingconnectionsourcewith@'localhost'or@'%'.2)GrantspecificprivilegeswithGRANT,usingleastprivilegeprincipletominimizerisks.3)
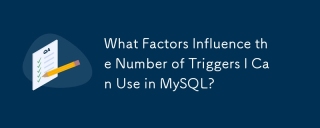
MySQLdoesn'timposeahardlimitontriggers,butpracticalfactorsdeterminetheireffectiveuse:1)Serverconfigurationimpactstriggermanagement;2)Complextriggersincreasesystemload;3)Largertablesslowtriggerperformance;4)Highconcurrencycancausetriggercontention;5)M
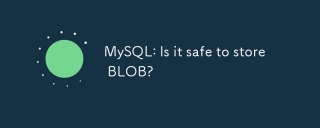
Yes,it'ssafetostoreBLOBdatainMySQL,butconsiderthesefactors:1)StorageSpace:BLOBscanconsumesignificantspace,potentiallyincreasingcostsandslowingperformance.2)Performance:LargerrowsizesduetoBLOBsmayslowdownqueries.3)BackupandRecovery:Theseprocessescanbe
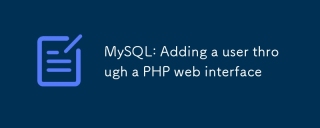
Adding MySQL users through the PHP web interface can use MySQLi extensions. The steps are as follows: 1. Connect to the MySQL database and use the MySQLi extension. 2. Create a user, use the CREATEUSER statement, and use the PASSWORD() function to encrypt the password. 3. Prevent SQL injection and use the mysqli_real_escape_string() function to process user input. 4. Assign permissions to new users and use the GRANT statement.
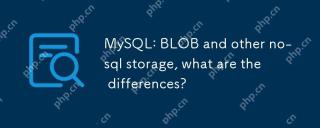
MySQL'sBLOBissuitableforstoringbinarydatawithinarelationaldatabase,whileNoSQLoptionslikeMongoDB,Redis,andCassandraofferflexible,scalablesolutionsforunstructureddata.BLOBissimplerbutcanslowdownperformancewithlargedata;NoSQLprovidesbetterscalabilityand
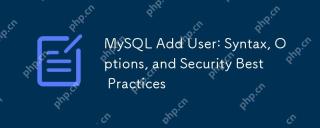
ToaddauserinMySQL,use:CREATEUSER'username'@'host'IDENTIFIEDBY'password';Here'showtodoitsecurely:1)Choosethehostcarefullytocontrolaccess.2)SetresourcelimitswithoptionslikeMAX_QUERIES_PER_HOUR.3)Usestrong,uniquepasswords.4)EnforceSSL/TLSconnectionswith
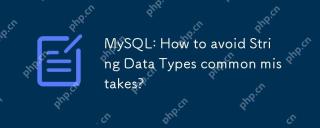
ToavoidcommonmistakeswithstringdatatypesinMySQL,understandstringtypenuances,choosetherighttype,andmanageencodingandcollationsettingseffectively.1)UseCHARforfixed-lengthstrings,VARCHARforvariable-length,andTEXT/BLOBforlargerdata.2)Setcorrectcharacters


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Atom editor mac version download
The most popular open source editor

Notepad++7.3.1
Easy-to-use and free code editor
