


Higher-order function - If a function receives parameters or returns a function, then we can call this function a higher-order function. As we all know, JavaScript is a weakly typed language: JavaScript functions do not strongly define or type-check input parameters or output values of the function. Then the function can become a parameter or an output value, which embodies the JavaScript Native support for higher-order functions.
1. Higher-order functions whose parameters are functions:
function funcTest(f){ //简易判断一下实参是否为函数 if((typeof f)==”function”){ f(); }} funcTest(function(){ });
This is a simple higher-order function that takes parameters as functions. When calling funcTest, input a function as a parameter, and execute the input anonymous function inside funcTest. Of course, such a code snippet has no practical meaning.
1. Higher-order functions whose return values are functions:
function funcTest(){ return function(){}; } var f=funcTest();
Calling funcTest returns a function.
2. A more complicated example:
//Number类型相加 function addInt(a,b){ return parseInt(a)+parseInt(b); } //String类型相加 function addString(a,b){ return a.toString()+ b.toString(); } function add(type){ if(type==="string"){ return addString; }else{ return addInt; } } var data1=add("string")("1","2"); //12 var data2=add("int")("1","2"); //3
The above example implements the separation of String type addition and Number type addition. When the add function is called, if the input parameter is "string", a string splicing function is output; if the input parameter is "int", a digital addition function is output.
3. The actual role of higher-order functions:
The above code example basically explains what higher-order functions are. Let’s take a look at how higher-order functions relate to our actual programming:
1, callback function
function callback(value){ alert(value); } function funcTest(value,f) //f实参检测,检查f是否为函数 if(typeof callback==='function'){ f(value);}}funcTest(‘1',callback); //1
The example is when funcTest is called, the callback function will be called internally in funcTest, that is, the callback is implemented.
2, Data screening and sorting algorithm
var arr=[0,2,11,9,7,5]; //排序算法 function funcComp(a,b){ if(a<b){ return -1; }else if(a>b){ return 1; }else{ return 0; } } //过滤算法 function funcFilter(item,index,array){ return item>=5; } //数组顺序排列 arr.sort(funcComp); alert(arr.join(',')); //0,2,5,7,9,11 //筛选数组 var arrFilter=arr.filter(funcFilter); alert(arr.join(‘,')) //5,7,9,11
3, DOM element event definition
<html><title></title> <body><input type=”button” value=”ClickMe” id=”myBtn” > <script type=”text/javascript> var btnClick=document.getElementById(“myBtn”); //测试环境为FireFox btnClick. addEventListener(“click”,function(e){ alert(“I'm a button!”); //I'm a button},false); </script> </body> </html>
In the above example, a button with the id myBtn is defined in the document, and the js script adds a click event to it, where the second parameter of addEventListener is a function.
Conclusion: High-order functions are not a patent of JavaScript, but they are definitely a powerful tool for JavaScript programming. Higher-order functions are actually a re-abstraction of basic algorithms. We can use this to improve the abstraction of the code, achieve maximum code reuse, and write code that is simpler and more conducive to refactoring.
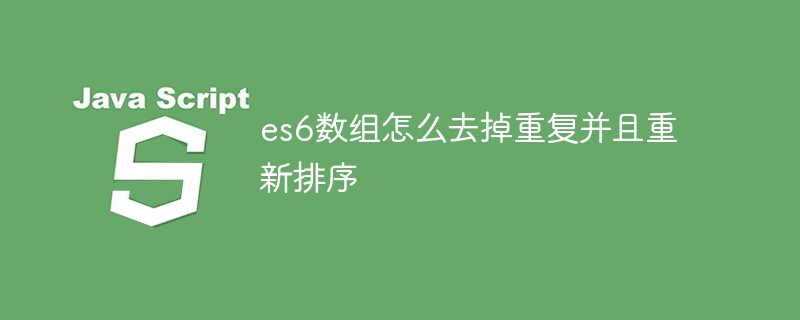
去掉重复并排序的方法:1、使用“Array.from(new Set(arr))”或者“[…new Set(arr)]”语句,去掉数组中的重复元素,返回去重后的新数组;2、利用sort()对去重数组进行排序,语法“去重数组.sort()”。
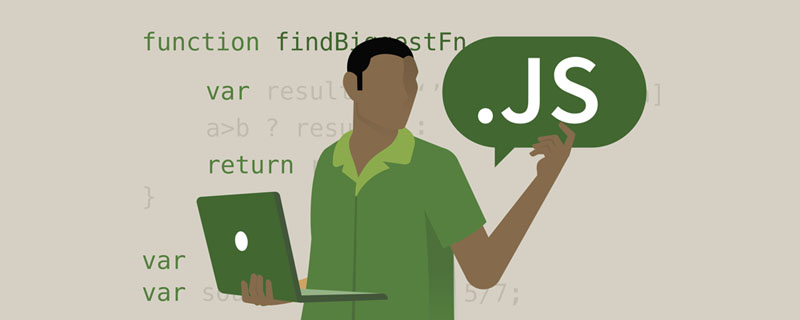
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于Symbol类型、隐藏属性及全局注册表的相关问题,包括了Symbol类型的描述、Symbol不会隐式转字符串等问题,下面一起来看一下,希望对大家有帮助。
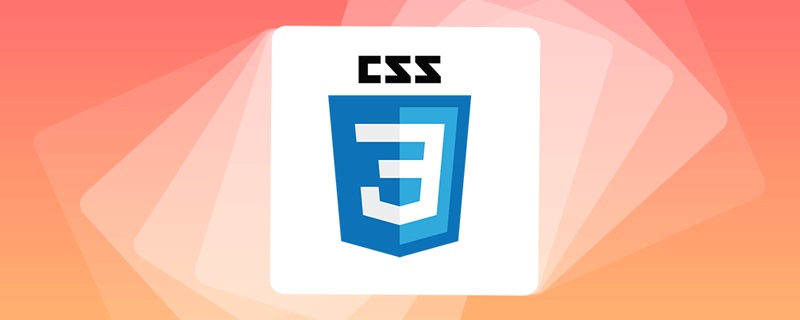
怎么制作文字轮播与图片轮播?大家第一想到的是不是利用js,其实利用纯CSS也能实现文字轮播与图片轮播,下面来看看实现方法,希望对大家有所帮助!
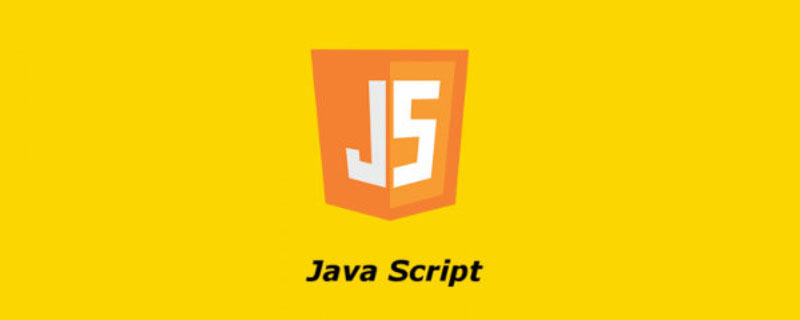
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于对象的构造函数和new操作符,构造函数是所有对象的成员方法中,最早被调用的那个,下面一起来看一下吧,希望对大家有帮助。
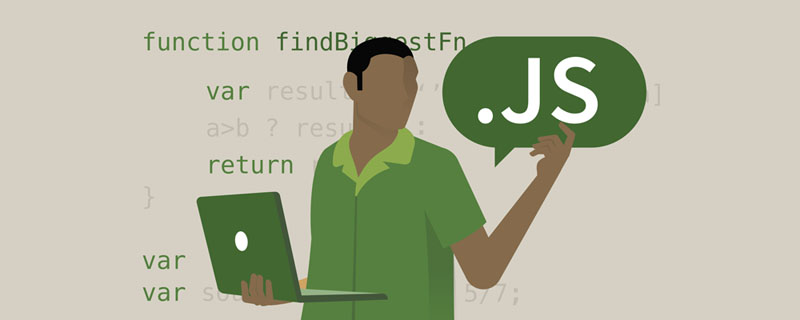
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于面向对象的相关问题,包括了属性描述符、数据描述符、存取描述符等等内容,下面一起来看一下,希望对大家有帮助。
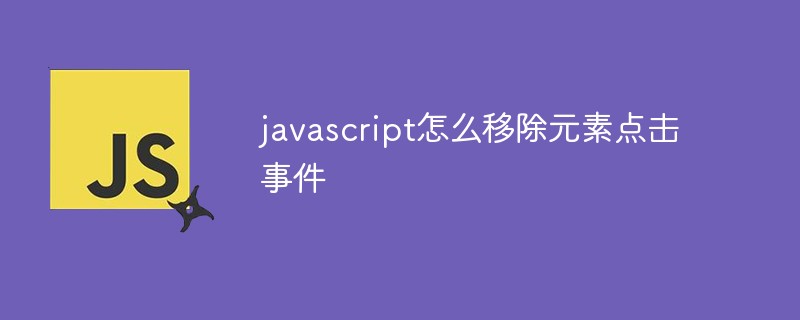
方法:1、利用“点击元素对象.unbind("click");”方法,该方法可以移除被选元素的事件处理程序;2、利用“点击元素对象.off("click");”方法,该方法可以移除通过on()方法添加的事件处理程序。
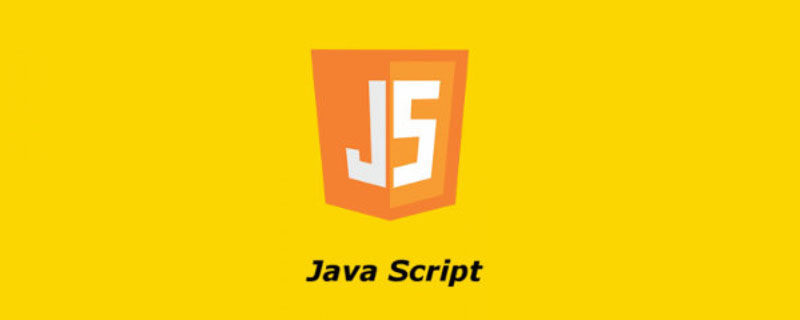
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于BOM操作的相关问题,包括了window对象的常见事件、JavaScript执行机制等等相关内容,下面一起来看一下,希望对大家有帮助。
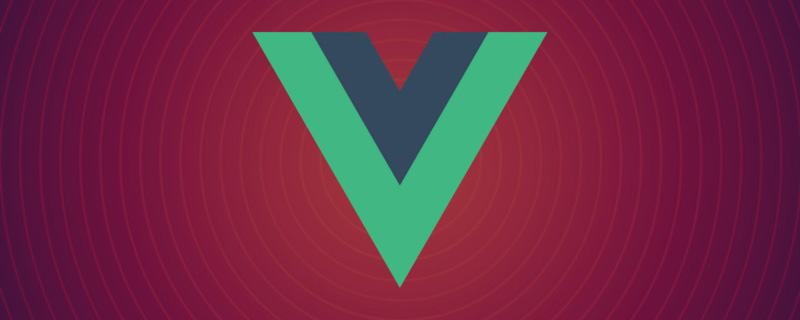
本篇文章整理了20+Vue面试题分享给大家,同时附上答案解析。有一定的参考价值,有需要的朋友可以参考一下,希望对大家有所帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
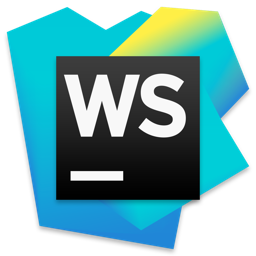
WebStorm Mac version
Useful JavaScript development tools
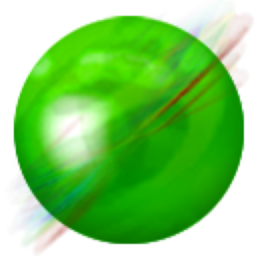
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
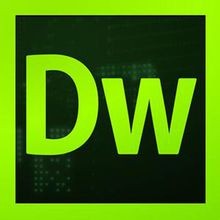
Dreamweaver CS6
Visual web development tools
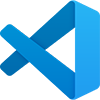
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 English version
Recommended: Win version, supports code prompts!
