Research on the practical application of division operator in Golang
Exploration on the practical application of division operator in Golang
Golang is a fast, efficient, concurrency-supported programming language with flexible syntax and powerful standard library Enables developers to easily handle various quantity calculation tasks. The division operator is one of the commonly used operators in programming, and it also has rich practical application scenarios in Golang. This article will explore the practical application of the division operator in Golang and demonstrate its usage through specific code examples.
1. Integer division
In Golang, when two integers are divided, the result is also an integer, that is, the integer part is taken directly and the decimal part is ignored. This kind of integer division has a wide range of application scenarios, such as when performing mathematical operations, logical judgments and data processing.
package main import "fmt" func main() { a := 10 b := 3 result := a / b fmt.Println(result) // 输出结果为3 }
In the above code example, the result of dividing the integer 10 by the integer 3 is 3. The decimal part is directly discarded, and the result is the integer 3.
2. Floating point division
Different from integer division, when floating point numbers are divided, Golang will retain the decimal part and return a floating point result. Floating point division is often used in situations where precise decimal calculations are required, such as financial calculations, scientific calculations, etc.
package main import "fmt" func main() { a := 10.0 b := 3.0 result := a / b fmt.Println(result) // 输出结果为3.3333333333333335 }
In the above code example, the result of dividing the floating point number 10.0 by the floating point number 3.0 is 3.3333333333333335, and the decimal part is retained to the exact number of digits.
3. Overflow handling
In Golang, division operations may cause overflow. When the divisor is 0, a panic error will occur; when integers are divided, if the result exceeds the representation range of the integer, overflow will also occur.
package main import "fmt" func main() { a := 1 b := 0 // 除数为0,会引发panic错误 result := a / b fmt.Println(result) }
In the above code example, dividing the integer 1 by 0 will cause a panic error. To avoid this, you can do some bounds checking before the division operation.
Conclusion
The division operator has a wide range of application scenarios in Golang. Whether it is integer division or floating point division, it can be flexibly used in various data processing tasks. In actual development, rational use of division operators can effectively improve the accuracy and efficiency of the program. I hope this article can help readers better understand the practical application of division operators in Golang.
Through the explanation of the above code examples, I believe that readers will have a deeper understanding of the use of division operators in Golang. It is hoped that readers can flexibly use the division operator in daily programming practice to improve the efficiency and reliability of the code.
The above is the detailed content of Research on the practical application of division operator in Golang. For more information, please follow other related articles on the PHP Chinese website!
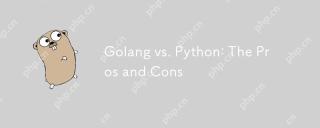
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
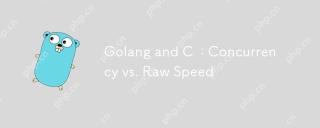
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
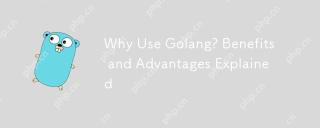
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
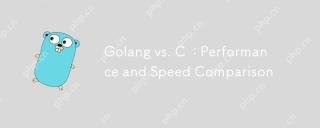
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
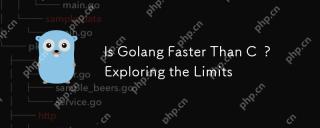
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
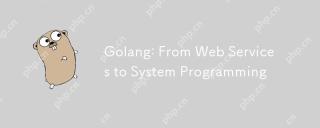
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
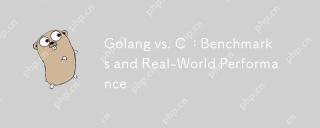
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
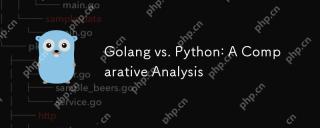
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Zend Studio 13.0.1
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.