Golang is a popular programming language with a unique design concept in concurrent programming. In Golang, the management of the stack (heap and stack) is a very important task and is crucial to understanding the operating mechanism of the Golang program. This article will delve into the differences in stacks in Golang and demonstrate the differences and connections between them through concrete code examples.
In computer science, stacks are two common memory allocation methods. They have different characteristics in memory management and data storage. In Golang, the heap is used to store dynamically allocated memory, while the stack is used to store local variables and function parameters when calling functions. There are significant differences between heap and stack in terms of memory allocation method, life cycle and access speed.
First, let us take a look at the memory allocation methods of heap and stack in Golang. In Golang, the heap is manually managed by programmers, using the new
or make
keywords for dynamic memory allocation. The stack is automatically managed by the compiler and is used to store local variables and function parameters when calling functions. The following is a simple code example:
package main import "fmt" func main() { // 声明一个变量在栈中 var x int = 10 fmt.Println("x的值为:", x) // 声明一个变量在堆中 y := new(int) *y = 20 fmt.Println("y的值为:", *y) }
In the above code example, the variable x
is stored in the stack, while the variable y
is stored in the heap. When we use the new
keyword to allocate memory for y
, we actually allocate memory space in the heap and point the address of the y
variable to this block memory space. This distinction has important implications for memory management of Golang programs.
Secondly, there are obvious differences in the life cycles of heap and stack. In Golang, the memory allocated on the heap requires programmers to manually manage its life cycle, and the memory needs to be manually released after use to avoid memory leaks. The memory allocated on the stack is automatically released at the end of the function call, without the need for manual management by the programmer. This automatic management feature makes Golang safer and more reliable in handling memory.
Finally, there is also a difference in access speed between heap and stack. Memory allocated on the stack is accessed faster because it is allocated contiguously and can be accessed directly through pointers. The access speed of memory allocated on the heap is relatively slow because it is allocated discontinuously and requires indirect access through pointers. Therefore, in scenarios with high performance requirements, trying to use the memory allocated on the stack can improve the execution efficiency of the program.
To sum up, the differences in stacks in Golang are reflected in the memory allocation method, life cycle and access speed. In actual programming, programmers need to choose appropriate memory allocation methods based on specific needs and reasonably manage memory resources to improve program performance and stability. Only by deeply understanding the characteristics of the stack in Golang can we better write efficient and robust Golang programs.
The above is the detailed content of A deep dive into the differences in stacks in Golang. For more information, please follow other related articles on the PHP Chinese website!
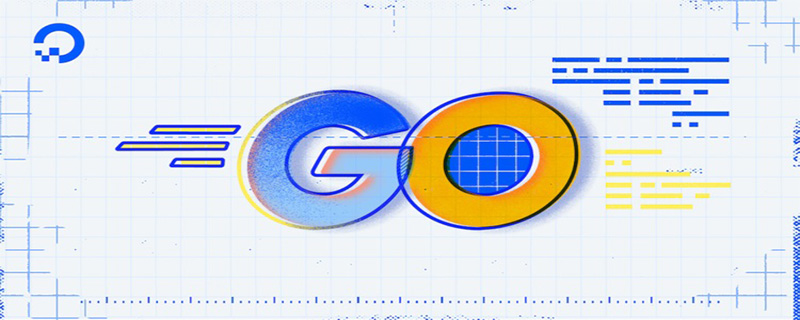
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
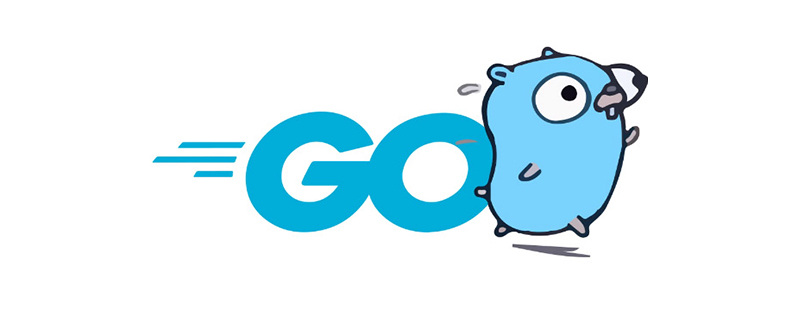
本篇文章带大家了解一下golang 的几种常用的基本数据类型,如整型,浮点型,字符,字符串,布尔型等,并介绍了一些常用的类型转换操作。
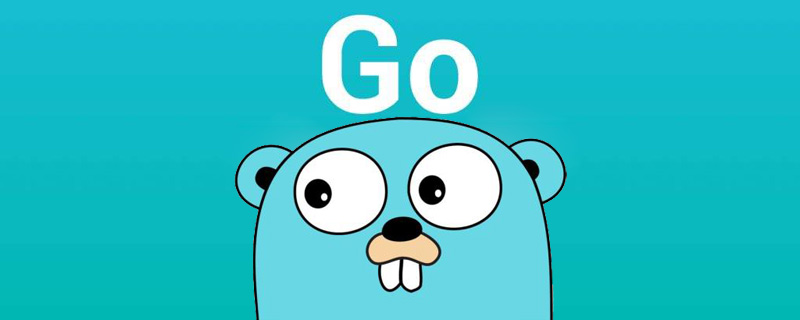
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
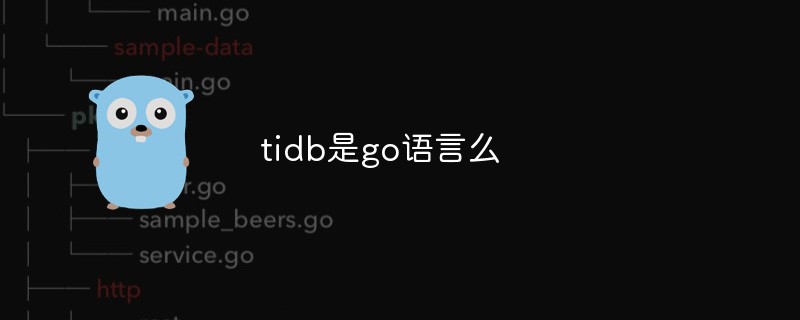
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
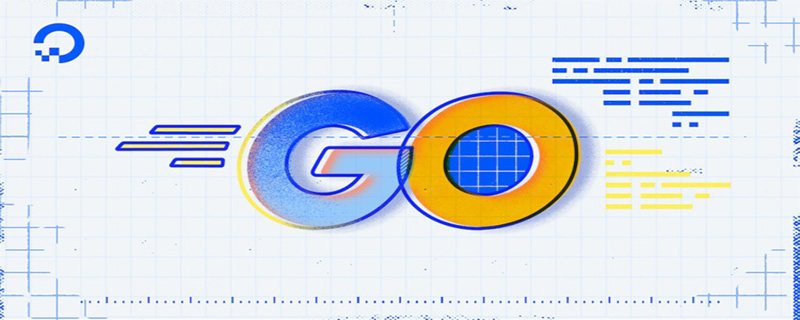
在写 Go 的过程中经常对比这两种语言的特性,踩了不少坑,也发现了不少有意思的地方,下面本篇就来聊聊 Go 自带的 HttpClient 的超时机制,希望对大家有所帮助。
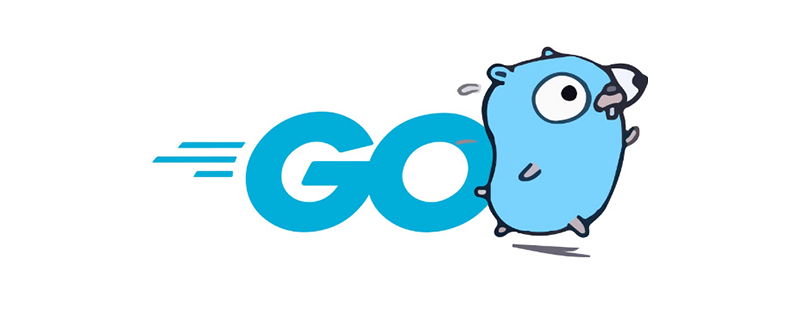
go语言需要编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言,也就说Go语言程序在运行之前需要通过编译器生成二进制机器码(二进制的可执行文件),随后二进制文件才能在目标机器上运行。
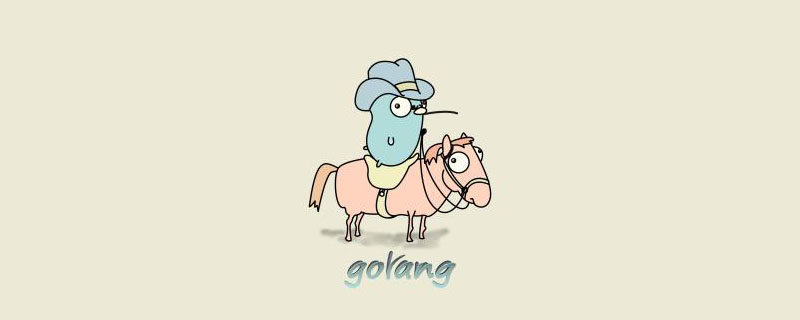
删除map元素的两种方法:1、使用delete()函数从map中删除指定键值对,语法“delete(map, 键名)”;2、重新创建一个新的map对象,可以清空map中的所有元素,语法“var mapname map[keytype]valuetype”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
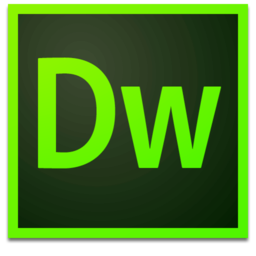
Dreamweaver Mac version
Visual web development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
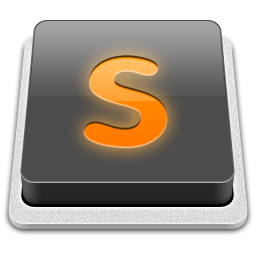
SublimeText3 Mac version
God-level code editing software (SublimeText3)
