Implementation method of Golang space replacement function
Title: Implementation method of Golang space replacement function
In Golang, space replacement is a common string operation that can be used Clear whitespace characters from text or replace them with other characters. This article will introduce how to implement a space replacement function in Golang and give specific code examples.
1. Use the strings.Replace function
Golang’s standard library provides the strings
package, which contains some convenient string processing functions, including Replace
Function can be used to replace the specified substring in a string. The following is a sample code that uses the strings.Replace
function to implement space replacement:
package main import ( "fmt" "strings" ) func replaceSpaces(input string, replacement rune) string { return strings.Replace(input, " ", string(replacement), -1) } func main() { text := "Hello World, Golang is awesome!" replacedText := replaceSpaces(text, '_') fmt.Println(replacedText) }
In the above code, we define a function named replaceSpaces
, Used to replace spaces in the input string input
with the characters specified by the replacement
parameter. main
The example in function shows how to call this function and replace spaces with underscores.
2. Custom replacement function
In addition to using the standard library function, we can also implement a space replacement function ourselves. The following is a sample code for a custom space replacement function:
package main import ( "fmt" ) func customReplaceSpaces(input string, replacement byte) string { replaced := make([]byte, 0, len(input)) for _, char := range input { if char == ' ' { replaced = append(replaced, replacement) } else { replaced = append(replaced, byte(char)) } } return string(replaced) } func main() { text := "Hello World, Golang is awesome!" replacedText := customReplaceSpaces(text, '_') fmt.Println(replacedText) }
In this example, we define a custom replacement function customReplaceSpaces
, which will traverse the input string and Spaces are replaced with the specified characters. main
The example in function shows how to call this custom function and replace spaces with underscores.
Conclusion
It is not difficult to implement the space replacement function in Golang. You can choose to use the function provided by the standard library or customize the implementation. No matter which method is used, spaces can be replaced flexibly according to actual needs. I hope the content of this article can help readers better understand how to implement the space replacement function in Golang.
The above is the detailed content of Implementation method of Golang space replacement function. For more information, please follow other related articles on the PHP Chinese website!
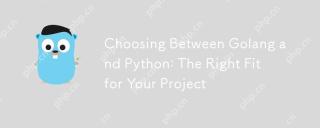
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
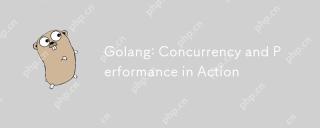
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
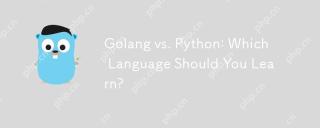
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
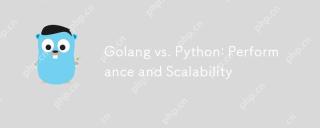
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
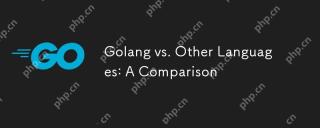
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
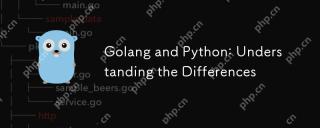
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
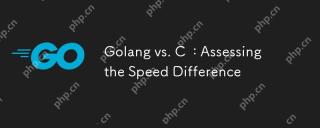
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
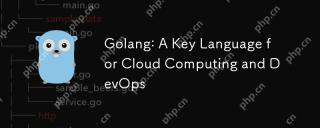
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
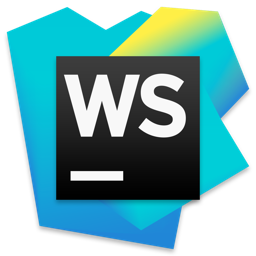
WebStorm Mac version
Useful JavaScript development tools
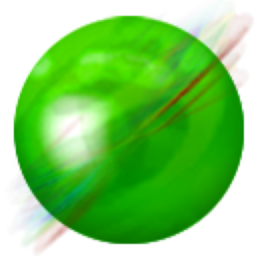
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.