The Complete Guide to Formatting Placeholders in Golang
Complete Guide to Formatting Placeholders in Golang
In Golang programming, formatting placeholders are a very important part, they allow you to define the style of output and structure, making the output easier to read and understand. This article will introduce formatted placeholders in Golang in detail, including common placeholder types and usage, as well as specific code examples.
1. Common formatting placeholders
In Golang, the common formatting placeholders are as follows:
- %v: Default format. When printing a structure, print the value of each field.
- % v: Similar to %v, but when printing the structure, the names of the structure fields will be included.
- %#v: Print the value represented by Go syntax, that is, the Go syntax representation of the value will be printed.
- %T: The type of printed value.
- %t: Print Boolean value.
- %d: Print integer value.
- %f: Print floating point value.
- %s: Print string.
- %p: Print pointer address.
In addition to the common placeholders mentioned above, Golang also supports more other types of placeholders. You can choose the appropriate placeholder to format the output according to your needs.
2. Code Examples
The following are some specific code examples showing how to use formatted placeholders in Golang:
Example 1: Printing using %v Integers and strings
package main import "fmt" func main() { num := 10 str := "Hello, Golang!" fmt.Printf("num: %v ", num) fmt.Printf("str: %v ", str) }
Run the above code, it will output:
num: 10 str: Hello, Golang!
Example 2: Use %v to print structure fields
package main import "fmt" type Person struct { Name string Age int } func main() { p := Person{"Alice", 25} fmt.Printf("%+v ", p) }
Run the above code, it will output:
{Name:Alice Age:25}
Example 3: Use %d and %f to print integers and floating point numbers
package main import "fmt" func main() { num := 10 pi := 3.14159 fmt.Printf("num: %d ", num) fmt.Printf("pi: %f ", pi) }
Running the above code will output:
num: 10 pi: 3.141590
Through the above examples, you can better Understand the usage and function of formatted placeholders in Golang. Depending on your needs, you can choose appropriate placeholders to format the output to make the code clearer and easier to read.
Conclusion
Formatting placeholders play an important role in Golang programming. It can help you better organize the output content and make the output more precise and clear. I hope that through the introduction of this article, you can better master the use of formatted placeholders in Golang and improve the readability and maintainability of the code.
The above is the detailed content of The Complete Guide to Formatting Placeholders in Golang. For more information, please follow other related articles on the PHP Chinese website!
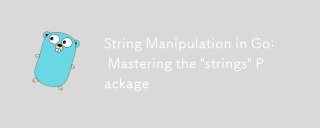
Mastering the strings package in Go language can improve text processing capabilities and development efficiency. 1) Use the Contains function to check substrings, 2) Use the Index function to find the substring position, 3) Join function efficiently splice string slices, 4) Replace function to replace substrings. Be careful to avoid common errors, such as not checking for empty strings and large string operation performance issues.
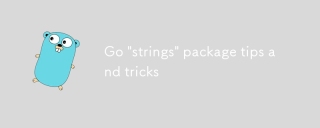
You should care about the strings package in Go because it simplifies string manipulation and makes the code clearer and more efficient. 1) Use strings.Join to efficiently splice strings; 2) Use strings.Fields to divide strings by blank characters; 3) Find substring positions through strings.Index and strings.LastIndex; 4) Use strings.ReplaceAll to replace strings; 5) Use strings.Builder to efficiently splice strings; 6) Always verify input to avoid unexpected results.
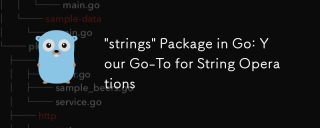
ThestringspackageinGoisessentialforefficientstringmanipulation.1)Itofferssimpleyetpowerfulfunctionsfortaskslikecheckingsubstringsandjoiningstrings.2)IthandlesUnicodewell,withfunctionslikestrings.Fieldsforwhitespace-separatedvalues.3)Forperformance,st
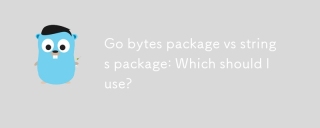
WhendecidingbetweenGo'sbytespackageandstringspackage,usebytes.Bufferforbinarydataandstrings.Builderforstringoperations.1)Usebytes.Bufferforworkingwithbyteslices,binarydata,appendingdifferentdatatypes,andwritingtoio.Writer.2)Usestrings.Builderforstrin
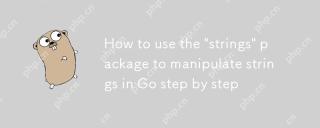
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
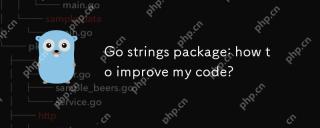
Using the Go language strings package can improve code quality. 1) Use strings.Join() to elegantly connect string arrays to avoid performance overhead. 2) Combine strings.Split() and strings.Contains() to process text and pay attention to case sensitivity issues. 3) Avoid abuse of strings.Replace() and consider using regular expressions for a large number of substitutions. 4) Use strings.Builder to improve the performance of frequently splicing strings.
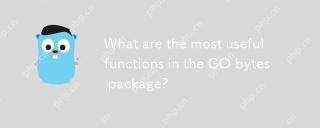
Go's bytes package provides a variety of practical functions to handle byte slicing. 1.bytes.Contains is used to check whether the byte slice contains a specific sequence. 2.bytes.Split is used to split byte slices into smallerpieces. 3.bytes.Join is used to concatenate multiple byte slices into one. 4.bytes.TrimSpace is used to remove the front and back blanks of byte slices. 5.bytes.Equal is used to compare whether two byte slices are equal. 6.bytes.Index is used to find the starting index of sub-slices in largerslices.
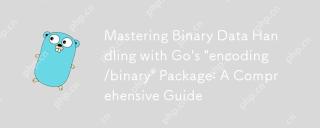
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor
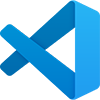
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
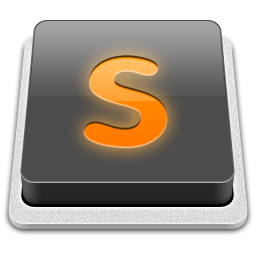
SublimeText3 Mac version
God-level code editing software (SublimeText3)
