


Streamlined code structure: Sharing of optimization tips for removing else in Go language
When writing code in Go language, if-else statements are often used to make conditional judgments. However, in some cases, we can optimize the code structure and remove the else keyword to make the code more concise and readable. Here are some specific examples of other optimization techniques for removing else.
Example 1: Use if to return directly
func isPositive(num int) bool { if num >= 0 { return true } return false }
can be simplified to:
func isPositive(num int) bool { if num >= 0 { return true } return false }
Example 2: Nested if statement
func checkAge(age int) string { if age < 18 { return "未成年" } else { return "成年" } }
can be simplified to:
func checkAge(age int) string { if age < 18 { return "未成年" } return "成年" }
Example 3: Continuous execution of conditional judgment
func checkNum(num int) { if num < 0 { fmt.Println("负数") } else if num > 0 { fmt.Println("正数") } else { fmt.Println("零") } }
can be simplified to:
func checkNum(num int) { if num < 0 { fmt.Println("负数") return } if num > 0 { fmt.Println("正数") return } fmt.Println("零") }
Through the above example, we can see how to remove the else keyword to streamline the Go language code structure , making the code more compact and concise. Of course, in actual development, flexible application of these optimization techniques according to specific circumstances can improve the readability and maintainability of the code.
The above is the detailed content of Streamlined code structure: Sharing of optimization tips for removing else in Go language. For more information, please follow other related articles on the PHP Chinese website!
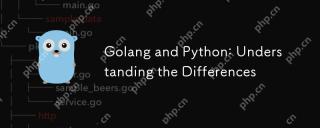
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
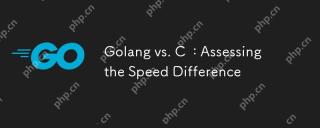
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
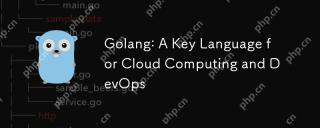
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.
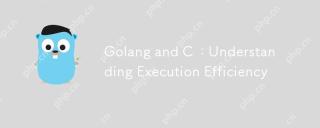
Golang and C each have their own advantages in performance efficiency. 1) Golang improves efficiency through goroutine and garbage collection, but may introduce pause time. 2) C realizes high performance through manual memory management and optimization, but developers need to deal with memory leaks and other issues. When choosing, you need to consider project requirements and team technology stack.
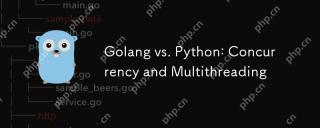
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
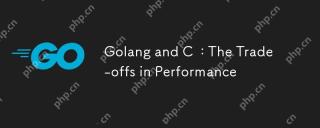
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
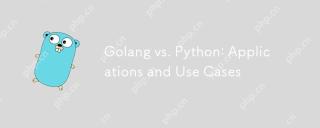
ChooseGolangforhighperformanceandconcurrency,idealforbackendservicesandnetworkprogramming;selectPythonforrapiddevelopment,datascience,andmachinelearningduetoitsversatilityandextensivelibraries.

Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
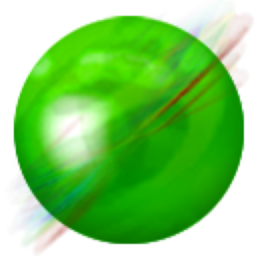
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
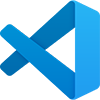
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.