


When writing PHP code, using classes (Class) is a very common practice. By using classes, we can encapsulate related functions and data in a single unit, making the code clearer, easier to read, and easier to maintain. This article will introduce the usage of PHP Class in detail and provide specific code examples to help readers better understand how to apply classes in actual projects to optimize code.
1. Create and use classes
In PHP, you can use the keyword class
to define a class and define properties and methods in the class. Here is a simple example:
class User { public $name; public $email; public function __construct($name, $email) { $this->name = $name; $this->email = $email; } public function greet() { return "Hello, my name is {$this->name} and my email is {$this->email}."; } } $user = new User("Alice", "alice@example.com"); echo $user->greet();
In the above example, we have defined a class named User
which contains $name
and $email
Two properties, as well as a __construct
constructor and a greet
method. Creating an instance of a class and calling methods on that instance makes it easy to access and manipulate the properties of the class.
2. Class inheritance and overriding
PHP supports class inheritance. Subclasses can inherit the properties and methods of the parent class, and can change the parent class through override. Behavior. The following is an example of inheritance and overriding:
class Admin extends User { public function greet() { return "Hello, my name is {$this->name}, I am an administrator."; } } $admin = new Admin("Bob", "bob@example.com"); echo $admin->greet();
In the above example, we define a Admin
class, which inherits from the User
class, and overrides Wrote the greet
method. In this way, we can flexibly extend and customize the functions of the class to implement more complex logic.
3. Static properties and methods of classes
In addition to instance properties and methods, PHP also supports static properties and methods, which can be accessed and called at the class level without creating a class Example. Here is an example of static properties and methods:
class Math { public static $pi = 3.14; public static function doublePi() { return self::$pi * 2; } } echo Math::$pi; // 输出 3.14 echo Math::doublePi(); // 输出 6.28
In the above example, we have defined a Math
class which contains a static property $pi
and A static method doublePi
. Through Math::$pi
and Math::doublePi()
, you can directly access static properties and call static methods without creating an instance of the class.
4. Use of namespaces
In order to avoid class name conflicts and organize code, PHP provides the namespace function. By using namespaces, classes can be grouped in different namespaces to avoid class name conflicts. Here is an example of a namespace:
namespace MyProject; class MyClass { // 类的定义 }
In the above example, we have defined the MyClass
class in the MyProject
namespace. When referencing this class in other files, you can use the use
keyword to specify the namespace to avoid class name conflicts.
Conclusion
By rationally using classes and object-oriented programming ideas, PHP code can be made clearer, modular and easier to maintain. In actual projects, rational design and use of classes are important means to improve code quality and development efficiency. I hope that the usage of PHP Class introduced in this article can help readers better understand and apply classes, and achieve excellent code structure and logical design in actual projects.
The above is the detailed content of Detailed explanation of PHP Class usage: Make your code clearer and easier to read. For more information, please follow other related articles on the PHP Chinese website!
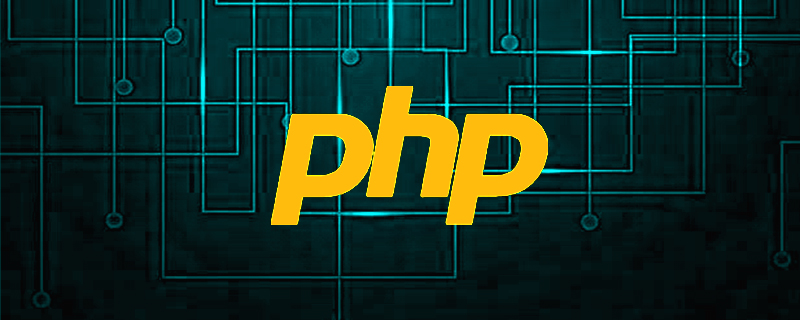
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
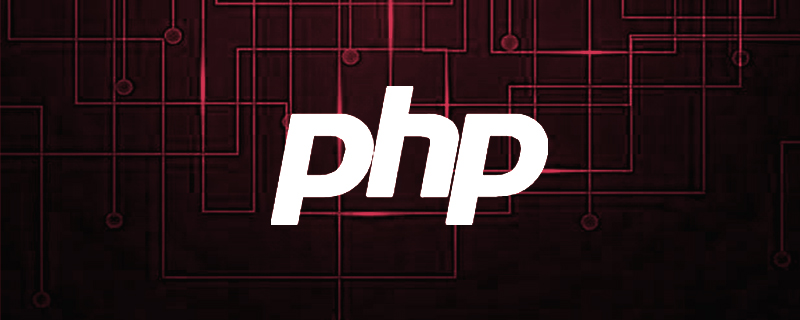
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
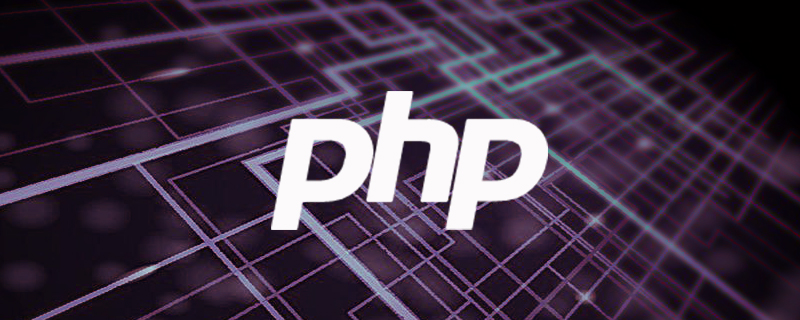
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
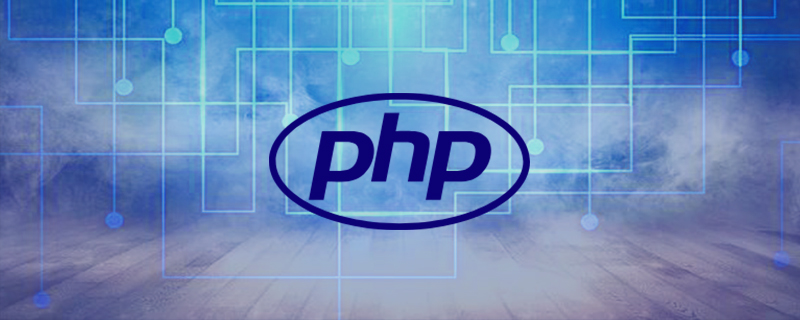
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
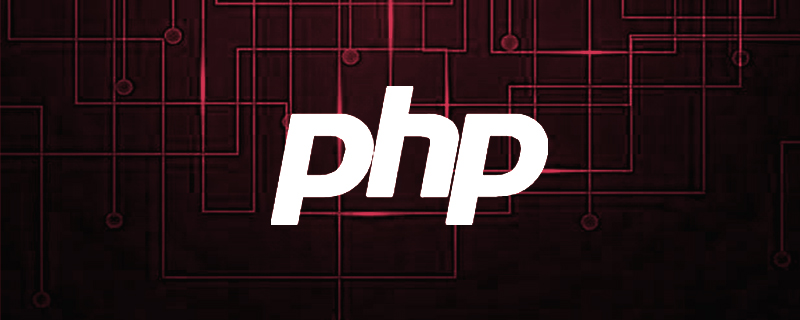
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
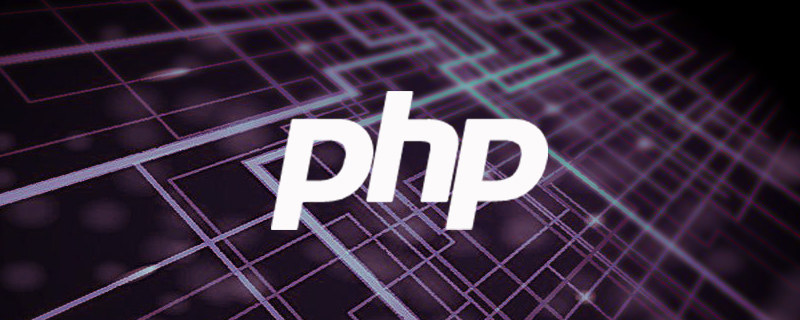
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。
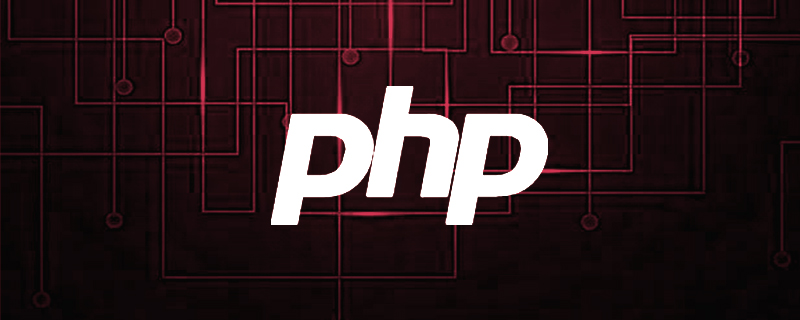
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
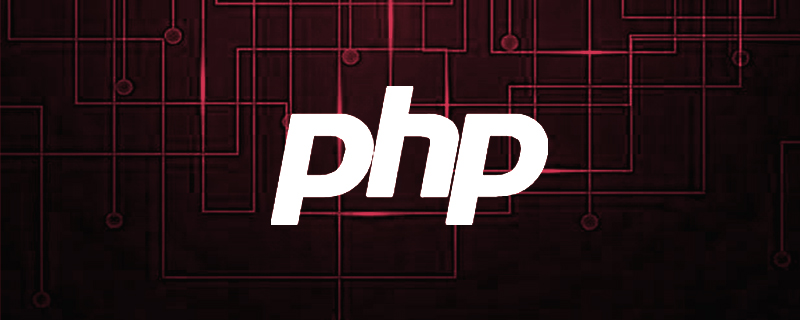
查找方法:1、用strpos(),语法“strpos("字符串值","查找子串")+1”;2、用stripos(),语法“strpos("字符串值","查找子串")+1”。因为字符串是从0开始计数的,因此两个函数获取的位置需要进行加1处理。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
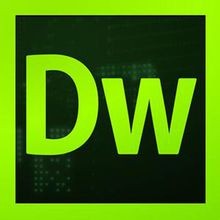
Dreamweaver CS6
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool