Go language is a fast, concise, safe and efficient programming language that has an increasing influence in the field of software development in recent years. In the Go language, function is a very important concept. It is the most basic unit of the program. This article will introduce the basic concepts and common usage of Go language functions, and attach specific code examples.
1. Definition of function
In Go language, the definition format of function is as follows:
func 函数名(参数列表) 返回值列表 { // 函数体 }
Among them, func
is the keyword to define the function , the function name can be any legal identifier, the parameter list is enclosed in parentheses, if there are multiple parameters, separated by commas, the return value list is after the parameter list, indicating the return value of the function. If the function does not return a value, the return value list can be omitted.
2. Function call
After defining the function, you can call it through the function name. The sample code is as follows:
package main import "fmt" // 定义一个简单的函数,实现两数相加 func add(x, y int) int { return x + y } func main() { sum := add(3, 5) fmt.Println("3 + 5 =", sum) }
Run the above code, the output result is:
3 + 5 = 8
3. Multiple return values of functions
Go language supports functions returning multiple values. The sample code is as follows:
package main import "fmt" // 定义一个函数,返回两个值 func divmod(a, b int) (int, int) { return a / b, a % b } func main() { quotient, remainder := divmod(10, 3) fmt.Printf("10 ÷ 3 = %d, 余 %d ", quotient, remainder) }
Run the above code, the output result is:
10 ÷ 3 = 3, 余 1
4. Anonymous function
Anonymous function is a function without a function name. It can be directly assigned to a variable or passed as a parameter. The sample code is as follows:
package main import "fmt" func main() { add := func(x, y int) int { return x + y } sum := add(4, 6) fmt.Println("4 + 6 =", sum) }
Run the above code and output The result is:
4 + 6 = 10
5. Closure
A closure is an entity composed of a function and its related reference environment. Closures can capture variables in external functions and operate on them. The sample code is as follows:
package main import "fmt" func outer() func() { count := 0 return func() { count++ fmt.Println("count:", count) } } func main() { f := outer() f() // count: 1 f() // count: 2 }
Run the above code, the output result is:
count: 1 count: 2
Through the above introduction, we understand Go Basic concepts and common usage of language functions, including function definition, function calling, multiple return values, anonymous functions and closures. Functions are an important part of the Go language, and mastering the use of functions will help improve the readability and reusability of the code. I hope this article will be helpful to you in learning Go language functions.
The above is the detailed content of Introduction to the basic concepts and usage of Go language functions. For more information, please follow other related articles on the PHP Chinese website!
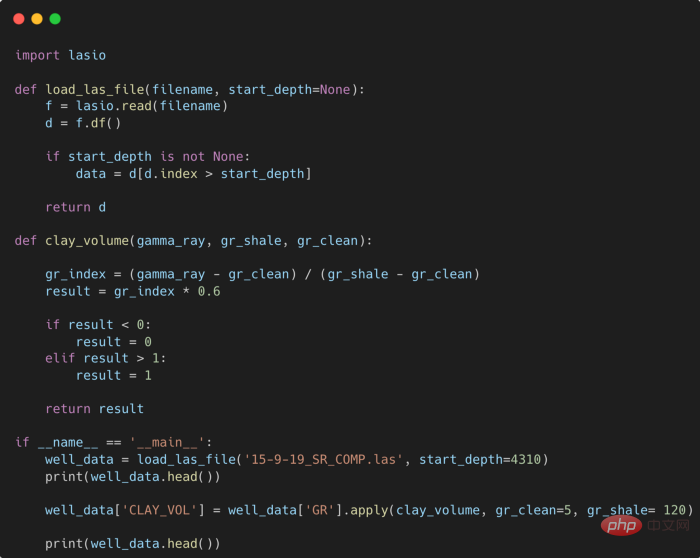
Python 中有许多方法可以帮助我们理解代码的内部工作原理,良好的编程习惯,可以使我们的工作事半功倍!例如,我们最终可能会得到看起来很像下图中的代码。虽然不是最糟糕的,但是,我们需要扩展一些事情,例如:load_las_file 函数中的 f 和 d 代表什么?为什么我们要在 clay 函数中检查结果?这些函数需要什么类型?Floats? DataFrames?在本文中,我们将着重讨论如何通过文档、提示输入和正确的变量名称来提高应用程序/脚本的可读性的五个基本技巧。1. Comments我们可
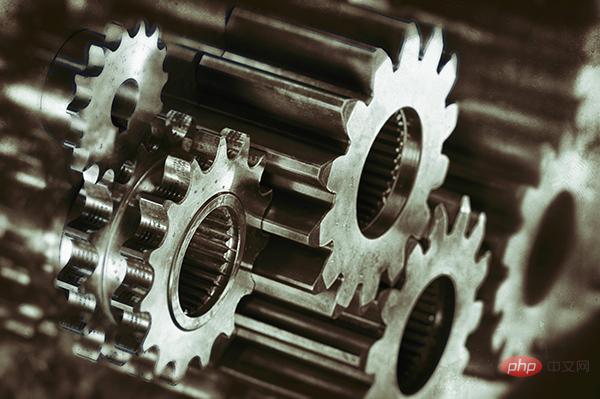
连续分级概率评分(Continuous Ranked Probability Score, CRPS)或“连续概率排位分数”是一个函数或统计量,可以将分布预测与真实值进行比较。机器学习工作流程的一个重要部分是模型评估。这个过程本身可以被认为是常识:将数据分成训练集和测试集,在训练集上训练模型,并使用评分函数评估其在测试集上的性能。评分函数(或度量)是将真实值及其预测映射到一个单一且可比较的值 [1]。例如,对于连续预测可以使用 RMSE、MAE、MAPE 或 R 平方等评分函数。如果预测不是逐点
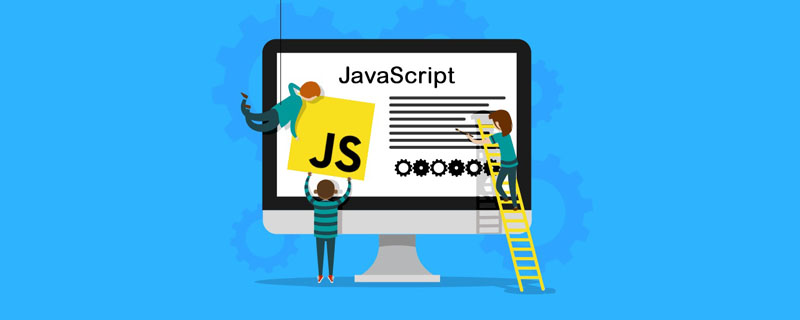
js是弱类型语言,不能像C#那样使用param关键字来声明形参是一个可变参数。那么js中,如何实现这种可变参数呢?下面本篇文章就来聊聊JavaScript函数可变参数的实现方法,希望对大家有所帮助!
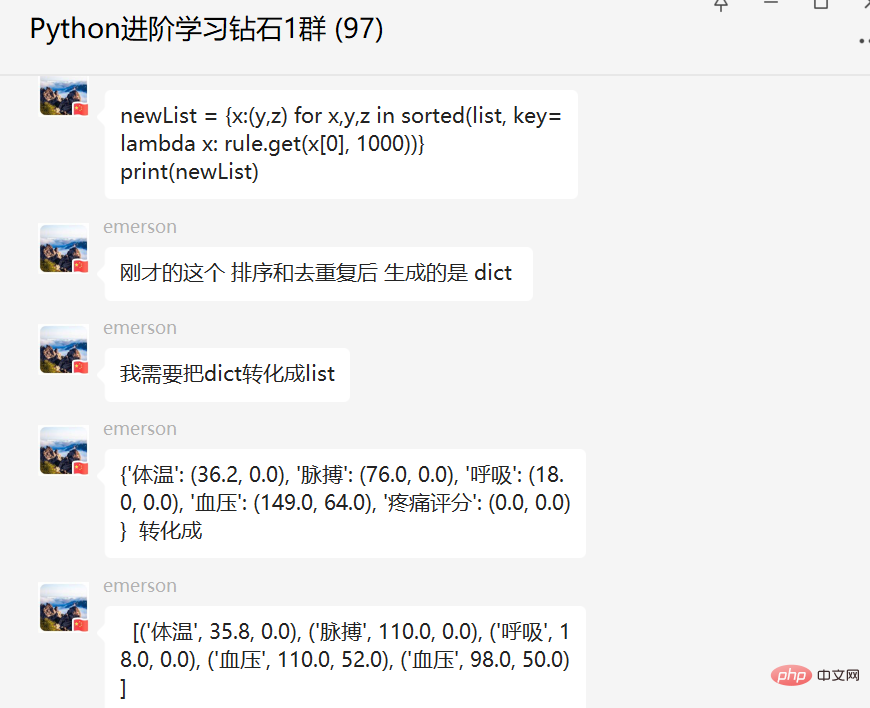
一、前言前几天在Python钻石交流群有个叫【emerson】的粉丝问了一个Python排序的问题,这里拿出来给大家分享下,一起学习下。其实这里【瑜亮老师】、【布达佩斯的永恒】等人讲了很多,只不过对于基础不太好的小伙伴们来说,还是有点难的。不过在实际应用中内置函数sorted()用的还是蛮多的,这里也单独拿出来讲一下,希望下次再有小伙伴遇到的时候,可以不慌。二、基础用法内置函数sorted()可以用来做排序,基础的用法很简单,看个例子,如下所示。lst=[3,28,18,29,2,5,88
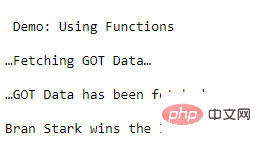
Python 中的 main 函数充当程序的执行点,在 Python 编程中定义 main 函数是启动程序执行的必要条件,不过它仅在程序直接运行时才执行,而在作为模块导入时不会执行。要了解有关 Python main 函数的更多信息,我们将从如下几点逐步学习:什么是 Python 函数Python 中 main 函数的功能是什么一个基本的 Python main() 是怎样的Python 执行模式Let’s get started什么是 Python 函数相信很多小伙伴对函数都不陌生了,函数是可

好嘞,今天我们继续剖析下Python里的类。[[441842]]先前我们定义类的时候,使用到了构造函数,在Python里的构造函数书写比较特殊,他是一个特殊的函数__init__,其实在类里,除了构造函数还有很多其他格式为__XXX__的函数,另外也有一些__xx__的属性。下面我们一一说下:构造函数Python里所有类的构造函数都是__init__,其中根据我们的需求,构造函数又分为有参构造函数和无惨构造函数。如果当前没有定义构造函数,那么系统会自动生成一个无参空的构造函数。例如:在有继承关系
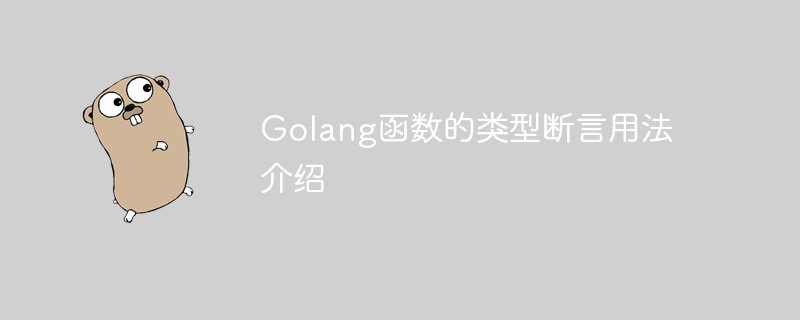
Golang的函数类型断言是一个非常重要的特性,它可以让我们在函数中精细地控制变量的类型,从而更加方便地进行数据处理和转换。本文将介绍Golang函数的类型断言用法,希望能够对大家的学习有所帮助。一、什么是Golang函数的类型断言?Golang函数的类型断言可以理解为函数参数中所声明变量的类型具有多态性,这使得一个函数在不同的参数传递下可以灵活
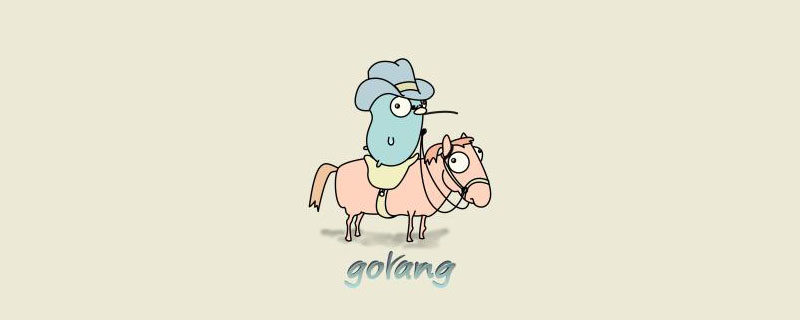
形参变量在未出现函数调用时并不占用内存,只在调用时才占用,调用结束后将释放内存。形参全称“形式参数”,是函数定义时使用的参数;但函数定义时参数是没有任实际何数据的,因而在函数被调用前没有为形参分配内存,其作用是说明自变量的类型和形态以及在过程中的作用。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
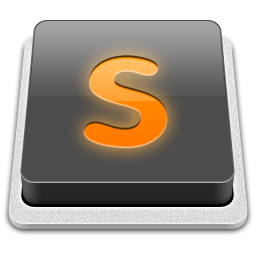
SublimeText3 Mac version
God-level code editing software (SublimeText3)
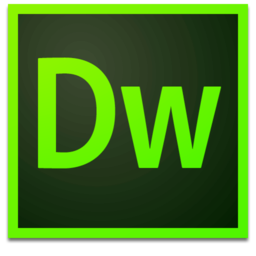
Dreamweaver Mac version
Visual web development tools

SublimeText3 Chinese version
Chinese version, very easy to use
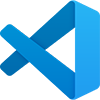
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Linux new version
SublimeText3 Linux latest version
