Laravel Redis Tutorial: Quickly master usage, specific code examples are required
In modern web development, caching is one of the important means to improve website performance. As a high-performance in-memory database, Redis is widely used in various web applications. In this tutorial, we will introduce how to use Redis in the Laravel framework to improve performance and expand functionality.
1. Install Redis
Before starting, first make sure that the Redis service has been installed in your development environment. You can check whether Redis has been installed by running the following command:
$ redis-cli -v
If it has been installed, the version information of Redis will be displayed. If it is not installed, you can install Redis through the following command:
$ sudo apt-get install redis-server
After the installation is completed, start the Redis service:
$ redis-server
2. Configure the Laravel project
Use in the Laravel project Redis is very simple. We only need to configure the Redis connection information in the .env
file. Open the .env
file and add the following configuration:
REDIS_HOST=127.0.0.1 REDIS_PORT=6379 REDIS_PASSWORD=null
The address, port and password of the Redis service are configured here. Modify them according to your actual configuration.
3. Use Redis cache
- Cache data
In Laravel, we can use the Cache
facade to operate the cache. The following are some operation examples:
use IlluminateSupportFacadesCache; // 缓存数据,有效期为10分钟 Cache::put('key', 'value', 10); // 获取缓存数据 $value = Cache::get('key'); // 检查缓存是否存在 if (Cache::has('key')) { // 缓存存在 } else { // 缓存不存在 } // 删除缓存 Cache::forget('key');
- Using Redis as a cache driver
In the config/cache.php
configuration file, we can set Redis As a cache driver:
'default' => env('CACHE_DRIVER', 'redis'),
In this way, we can use Redis as the default cache driver.
4. Using Redis Queue
In addition to serving as a cache, Redis can also be used to process queue tasks. In Laravel, we can set the queue driver to Redis and use the power of Redis to process queue tasks. The following are some usage examples:
- Queue task enqueue:
use IlluminateSupportFacadesQueue; Queue::push('AppJobsProcessPost', ['post_id' => 1]);
- Queue task dequeue:
$ php artisan queue:work
The above is Some basic usage examples. In this way, we can use Redis to process a large number of tasks asynchronously and improve the performance and response speed of the system.
5. Other Redis functions
In addition to caching and queues, Redis also has many other functions, such as publishing and subscription, transaction management, etc. In Laravel, we can also use these functions of Redis to implement more business logic requirements. Here are some examples:
- Publish Subscription:
use IlluminateSupportFacadesRedis; Redis::publish('channel', 'message');
- Transaction Management:
use IlluminateSupportFacadesRedis; Redis::transaction(function ($redis) { $redis->set('key1', 'value1'); $redis->set('key2', 'value2'); });
Through the above examples, we can see It is very simple to use Redis to implement various functions in Laravel, and it can be completed with only a few lines of code. The high performance and flexibility of Redis provide more possibilities for our applications and help us improve user experience and system performance.
Summary: Redis is widely used in Laravel. Whether it is used as a cache, queue or other functions, Redis can provide us with effective solutions. I hope that through this tutorial, you can quickly master the usage of Redis in Laravel and improve your skills and level in web development.
The above is the detailed content of Laravel Redis Tutorial: Quickly Master Usage. For more information, please follow other related articles on the PHP Chinese website!

Laravel is suitable for building web applications quickly, while Python is suitable for a wider range of application scenarios. 1.Laravel provides EloquentORM, Blade template engine and Artisan tools to simplify web development. 2. Python is known for its dynamic types, rich standard library and third-party ecosystem, and is suitable for Web development, data science and other fields.

Laravel and Python each have their own advantages: Laravel is suitable for quickly building feature-rich web applications, and Python performs well in the fields of data science and general programming. 1.Laravel provides EloquentORM and Blade template engines, suitable for building modern web applications. 2. Python has a rich standard library and third-party library, and Django and Flask frameworks meet different development needs.

Laravel is worth choosing because it can make the code structure clear and the development process more artistic. 1) Laravel is based on PHP, follows the MVC architecture, and simplifies web development. 2) Its core functions such as EloquentORM, Artisan tools and Blade templates enhance the elegance and robustness of development. 3) Through routing, controllers, models and views, developers can efficiently build applications. 4) Advanced functions such as queue and event monitoring further improve application performance.

Laravel is not only a back-end framework, but also a complete web development solution. It provides powerful back-end functions, such as routing, database operations, user authentication, etc., and supports front-end development, improving the development efficiency of the entire web application.

Laravel is suitable for web development, Python is suitable for data science and rapid prototyping. 1.Laravel is based on PHP and provides elegant syntax and rich functions, such as EloquentORM. 2. Python is known for its simplicity, widely used in Web development and data science, and has a rich library ecosystem.

Laravelcanbeeffectivelyusedinreal-worldapplicationsforbuildingscalablewebsolutions.1)ItsimplifiesCRUDoperationsinRESTfulAPIsusingEloquentORM.2)Laravel'secosystem,includingtoolslikeNova,enhancesdevelopment.3)Itaddressesperformancewithcachingsystems,en

Laravel's core functions in back-end development include routing system, EloquentORM, migration function, cache system and queue system. 1. The routing system simplifies URL mapping and improves code organization and maintenance. 2.EloquentORM provides object-oriented data operations to improve development efficiency. 3. The migration function manages the database structure through version control to ensure consistency. 4. The cache system reduces database queries and improves response speed. 5. The queue system effectively processes large-scale data, avoid blocking user requests, and improve overall performance.

Laravel performs strongly in back-end development, simplifying database operations through EloquentORM, controllers and service classes handle business logic, and providing queues, events and other functions. 1) EloquentORM maps database tables through the model to simplify query. 2) Business logic is processed in controllers and service classes to improve modularity and maintainability. 3) Other functions such as queue systems help to handle complex needs.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Zend Studio 13.0.1
Powerful PHP integrated development environment
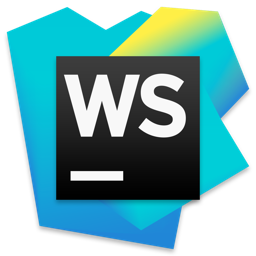
WebStorm Mac version
Useful JavaScript development tools
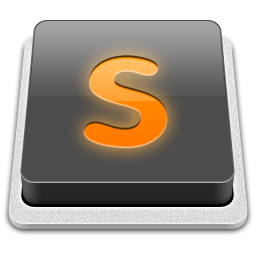
SublimeText3 Mac version
God-level code editing software (SublimeText3)