Oracle stored procedure batch update implementation method
Title: Oracle stored procedure batch update implementation method
In Oracle database, using stored procedures to batch update data is a common operation. Batch updates can improve the efficiency of data processing, reduce frequent access to the database, and also reduce the complexity of the code. This article will introduce how to use stored procedures to update data in batches in Oracle database, and give specific code examples.
First, we need to create a stored procedure that will implement the function of updating data in batches. The following is a simple stored procedure example. Suppose we have a table named "employee" and need to update employees' salaries in batches based on employee IDs:
CREATE OR REPLACE PROCEDURE batch_update_salary IS CURSOR c_employee IS SELECT employee_id, new_salary FROM employee WHERE salary < 5000; TYPE t_employee_id IS TABLE OF employee.employee_id%TYPE INDEX BY PLS_INTEGER; TYPE t_salary IS TABLE OF employee.salary%TYPE INDEX BY PLS_INTEGER; l_employee_ids t_employee_id; l_salaries t_salary; BEGIN -- 填充要更新的员工ID和新工资到两个数组中 FOR rec IN c_employee LOOP l_employee_ids(l_employee_ids.COUNT + 1) := rec.employee_id; l_salaries(l_salaries.COUNT + 1) := rec.new_salary; END LOOP; -- 批量更新员工工资 FOR i IN 1..l_employee_ids.COUNT LOOP UPDATE employee SET salary = l_salaries(i) WHERE employee_id = l_employee_ids(i); END LOOP; COMMIT; DBMS_OUTPUT.PUT_LINE('批量更新完成'); END; /
In this stored procedure, first we define a cursor "c_employee" to select the employee ID and new salary whose salary is less than 5000. Then we defined two PL/SQL table types "t_employee_id" and "t_salary" to store arrays of employee IDs and new salaries.
Then, in the main part of the stored procedure, we use the cursor to traverse the query results and fill in the employee ID and new salary into the array one by one. After that, a loop is traversed through the array to update the employee's salary to the new salary one by one.
Finally, we use the COMMIT statement to commit the transaction and output a prompt message through DBMS_OUTPUT.
To call this stored procedure, you can use the following SQL statement:
EXECUTE batch_update_salary;
Through the above code example, we show how to use stored procedures in Oracle database to implement the function of batch update data. When a large amount of data needs to be processed and batch updates are required, using stored procedures can improve efficiency and reduce code complexity. It is one of the important technologies in database development.
The above is the detailed content of Oracle stored procedure batch update implementation method. For more information, please follow other related articles on the PHP Chinese website!
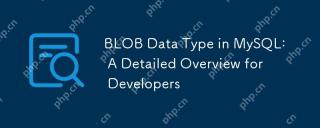
BlobdatatypesinmysqlareusedforvoringLargebinarydatalikeImagesoraudio.1) Useblobtypes (tinyblobtolongblob) Basedondatasizeneeds. 2) Storeblobsin Perplate Petooptimize Performance.3) ConsidersxterNal Storage Forel Blob Romana DatabasesizerIndimprovebackupupe
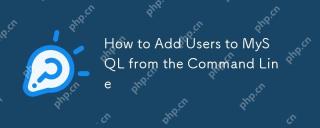
ToadduserstoMySQLfromthecommandline,loginasroot,thenuseCREATEUSER'username'@'host'IDENTIFIEDBY'password';tocreateanewuser.GrantpermissionswithGRANTALLPRIVILEGESONdatabase.*TO'username'@'host';anduseFLUSHPRIVILEGES;toapplychanges.Alwaysusestrongpasswo
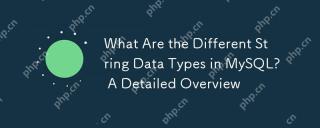
MySQLofferseightstringdatatypes:CHAR,VARCHAR,BINARY,VARBINARY,BLOB,TEXT,ENUM,andSET.1)CHARisfixed-length,idealforconsistentdatalikecountrycodes.2)VARCHARisvariable-length,efficientforvaryingdatalikenames.3)BINARYandVARBINARYstorebinarydata,similartoC
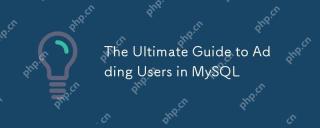
ToaddauserinMySQL,usetheCREATEUSERstatement.1)UseCREATEUSER'newuser'@'localhost'IDENTIFIEDBY'password';tocreateauser.2)Enforcestrongpasswordpolicieswithvalidate_passwordpluginsettings.3)GrantspecificprivilegesusingGRANTstatement.4)Forremoteaccess,use
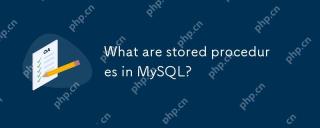
Stored procedures are precompiled SQL statements in MySQL for improving performance and simplifying complex operations. 1. Improve performance: After the first compilation, subsequent calls do not need to be recompiled. 2. Improve security: Restrict data table access through permission control. 3. Simplify complex operations: combine multiple SQL statements to simplify application layer logic.
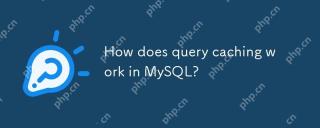
The working principle of MySQL query cache is to store the results of SELECT query, and when the same query is executed again, the cached results are directly returned. 1) Query cache improves database reading performance and finds cached results through hash values. 2) Simple configuration, set query_cache_type and query_cache_size in MySQL configuration file. 3) Use the SQL_NO_CACHE keyword to disable the cache of specific queries. 4) In high-frequency update environments, query cache may cause performance bottlenecks and needs to be optimized for use through monitoring and adjustment of parameters.
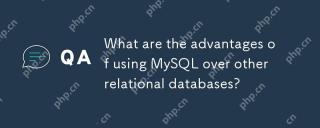
The reasons why MySQL is widely used in various projects include: 1. High performance and scalability, supporting multiple storage engines; 2. Easy to use and maintain, simple configuration and rich tools; 3. Rich ecosystem, attracting a large number of community and third-party tool support; 4. Cross-platform support, suitable for multiple operating systems.
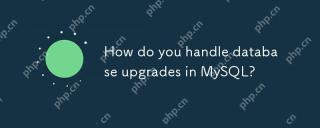
The steps for upgrading MySQL database include: 1. Backup the database, 2. Stop the current MySQL service, 3. Install the new version of MySQL, 4. Start the new version of MySQL service, 5. Recover the database. Compatibility issues are required during the upgrade process, and advanced tools such as PerconaToolkit can be used for testing and optimization.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
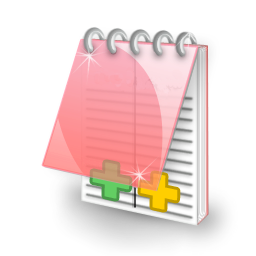
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Zend Studio 13.0.1
Powerful PHP integrated development environment
