


How to create electronic business cards using JavaScript's AngularJS library_AngularJS
Introduction
In this example, I referenced some JavaScript libraries including AngularJS to implement a very simple business card generator. Although the AngularJS library does not do much in this small application compared to other JavaScript libraries, this small but powerful AngularJS is the entire inspiration for this application.
Background
In this application, we need to do some simple work. First, we need to design the business card using CSS. Then, we need to allow users to input and edit data in real time, where AngularJS is indispensable. Then, we need to convert the HTML div container of the business card into a canvas and download it as a PNG image. It's that simple!
Use of code
Here, let me explain the following code block.
<!DOCTYPE html> <html> <head> <title>vCard Creator demo</title> <link rel="stylesheet" type="text/css" href="main.css"> </head> <body> <div id="wrapper" ng-app> <h1 id="Real-time-vCard-Creator">Real time vCard Creator</h1> <div id="editor"> <input placeholder="Company name" ng-model="cname"/> <input placeholder="Company tag line" ng-model="tagline"/> <input placeholder="Your full name" ng-model="name"/> <input placeholder="Your designation" ng-model="desig"/> <input placeholder="Phone number" ng-model="phone"/> <input placeholder="Email address" ng-model="email"/> <input placeholder="Website url" ng-model="url"/> <button id="saveBut">Download vCard as PNG</button> </div> <div id="card"> <header> <h4 id="cname">{{cname}}</h4> <p>{{tagline}}</p> </header> <div id="theBody"> <div id="theLeft"> <h2 id="name">{{name}}</h2> <h5 id="desig">{{desig}}</h5> </div> <div id="theRight"> <p>{{phone}}</p> <p>{{email}}</p> <p>{{url}}</p> </div> </div> </div> </div> <script type="text/javascript" src="angular.min.js"></script> <script type="text/javascript" src="jquery.min.js"></script> <script type="text/javascript" src="html2canvas.js"></script> <script type="text/javascript" src="canvas2image.js"></script> <script type="text/javascript" src="base64.com"></script> </body> </html>
This is the HTML structure of the application. This structure consists of two parts. One is the div with the id of editor, and the other is the div with the id of card. The former is used to allow users to enter information, and the latter is used to display information on business cards. These two divs are wrapped by a div with the ID of wrapper. In this div with the ID of wrapper, we will add the ng-app attribute, because it is in this div container that we will use angular. We can add ng-app to the HTML tag, so that we can use angular anywhere on the web page. Next, we create some input boxes to receive user input. Make sure that each input box has an attribute like ng-model, which is used to pass the corresponding value in the input box. We put the ng-model attribute here mainly because we want to update the value in the div with the id of card in real time. Now, inside the div with the id card, make sure we've placed some weird-looking double brackets, and inside the double brackets we've put the value from ng-model. Basically, after we enter content in the input box, the value in the double brackets changes immediately. So the editing of business cards ends here. Our goal is that when a user clicks the download button, the current business card will be converted into an image and downloaded to the user's computer.
#editor{ width:350px; background: silver; margin:0 auto; margin-top:20px; padding-top:10px; padding-bottom:10px; } input{ width:90%; margin-left:5px; } button{ margin-left:5px; } #card{ width:350px; height:200px; background:whitesmoke; box-shadow: 0 0 2px #323232; margin:0 auto; margin-top:20px; } header{ background:#323232; padding:5px; } header h4{ color:white; line-height:0; font-family:helvetica; margin:7px; margin-top:20px; text-shadow: 1px 1px black; text-transform:uppercase; } header p{ line-height:0; color:silver; font-size:10px; margin:11px; margin-bottom:20px; } #theBody{ background:blue; width:100%; height:auto; } #theLeft{ width:50%; float:left; text-align:right; } #theLeft h2{ margin-right:10px; margin-top:40px; font-family:helvetica; margin-bottom:0; color:#323232; } #theLeft h5{ margin-right:10px; font-family:helvetica; margin-top:5px; line-height:0; font-weight: bold; color:#323232; } #theRight{ width:50%; float:right; padding-top:42px; } #theRight p{ line-height:0; font-size:12px; color:#323232; font-family:Calibri; margin-left:15px; }
This is the CSS style for the app. Here we mock up a business card design and create an editing panel that allows users to enter information.
<script> $(function() { $("#saveBut").click(function() { html2canvas($("#card"), { onrendered: function(canvas) { theCanvas = canvas; Canvas2Image.saveAsPNG(canvas); } }); }); }); </script>
Finally, insert this script before the body closing tag of the HTML page. This script contains the event response corresponding to the download button. In other words, the function of the html2canvas function is to convert the div with the id of card into an HTML canvas, and after rendering the canvas, save it in the form of a PNG file. canvas canvas. After adding this script, all that needs to be done is done.
Notes
This canvas2image.js script code does not use the extension .png at the end of the generated file name by default. So if you cannot open the image, please rename the file name and add the .png extension to the end of the file name.
Online debugging jsFiddle
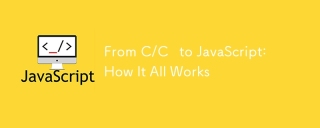
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
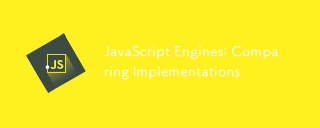
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
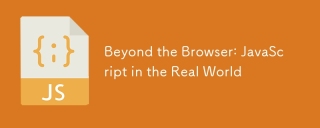
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
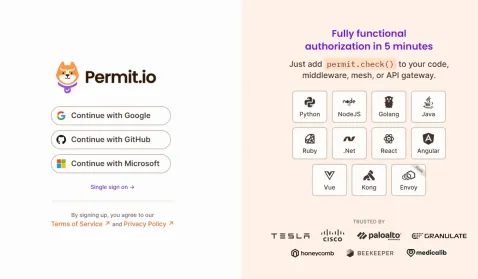
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
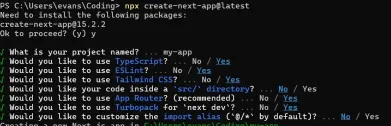
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
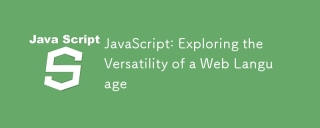
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
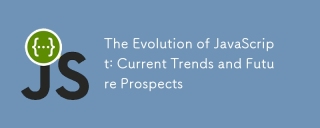
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
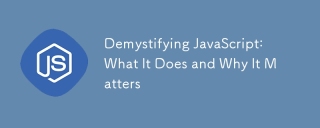
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
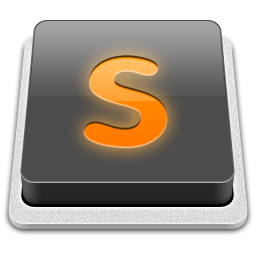
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
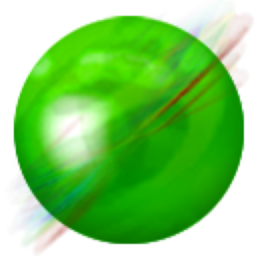
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment