


PHP is a server-side scripting language widely used in web development. Due to its flexibility and ease of use, it is widely used in the development of various websites and web applications. . In actual development work, we often encounter situations where we need to query multi-level classified data in the database and perform loop processing. This article will introduce how to use loops to query subcategories and optimize performance, and provide specific PHP code examples.
1. Data table structure design
Before you start writing PHP code, you first need to design the structure of the database table. Normally, multi-level classification data can be stored using the following table structure:
CREATE TABLE categories ( id INT PRIMARY KEY, name VARCHAR(50) NOT NULL, parent_id INT, INDEX parent_id_index (parent_id), FOREIGN KEY (parent_id) REFERENCES categories(id) ON DELETE CASCADE );
The above table structure contains the id, name and id of the parent category. The parent and child are established through parent_id and the id in the categories table. relation.
2. Use recursive query for subcategories
In PHP, you can query all subcategories recursively. The following is a simple example:
function getSubcategories($parent_id, $depth) { $categories = []; $sql = "SELECT * FROM categories WHERE parent_id = $parent_id"; $result = mysqli_query($conn, $sql); while ($row = mysqli_fetch_assoc($result)) { $categories[] = $row; if ($depth > 0) { $subcategories = getSubcategories($row['id'], $depth - 1); $categories = array_merge($categories, $subcategories); } } return $categories; } // 调用函数查询所有子分类 $subcategories = getSubcategories($parent_id, $depth);
In the above code, the getSubcategories function receives the id and depth parameters of the parent category, recursively queries all subcategories, and stores them in the $categories array. In each recursive call, it is judged whether the depth parameter is greater than 0, and if so, continue to query the subcategory downwards. Finally, an array containing all subcategories is returned.
3. Optimize performance
When processing multi-level classification data, if the classification level is deep, using recursive queries may cause performance problems. In order to optimize performance, you can consider using a circular query plus caching method. The following is an example of using loop query and caching:
function getSubcategoriesWithCache($parent_id) { $categories = []; $cache = []; $queue = [$parent_id]; $depth = 0; while (!empty($queue)) { $current_id = array_shift($queue); if (!isset($cache[$current_id])) { $sql = "SELECT * FROM categories WHERE parent_id = $current_id"; $result = mysqli_query($conn, $sql); while ($row = mysqli_fetch_assoc($result)) { $categories[] = $row; $cache[$row['id']] = true; $queue[] = $row['id']; } } if ($depth > 0) { $depth--; } else { break; } } return $categories; } // 调用函数查询所有子分类 $subcategories = getSubcategoriesWithCache($parent_id);
In the above code, the getSubcategoriesWithCache function uses loop query and combines the caching mechanism to reduce the number of database queries. Use an array $cache to record the categories that have been queried to avoid repeated queries. A queue $queue is used to store the category ID to be queried, and the subcategories are queried layer by layer by looping through the queue.
Conclusion
Through the method introduced in this article, multi-level classification data can be efficiently processed in PHP and performance optimized. Using recursive query can easily and quickly obtain all subcategories, while using circular query plus caching can reduce the number of database accesses and improve query efficiency. In actual projects, depending on the amount of classified data and the depth of the hierarchy, choose an appropriate method to process multi-level classified data to improve system performance and user experience.
The above is the detailed content of How to use loops to query subcategories and optimize performance in PHP. For more information, please follow other related articles on the PHP Chinese website!
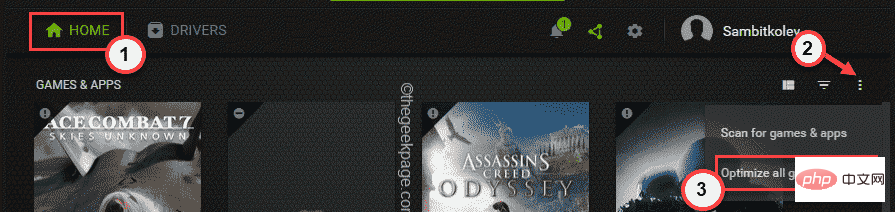
GeforceExperience不仅为您下载最新版本的游戏驱动程序,它还提供更多!最酷的事情之一是它可以根据您的系统规格优化您安装的所有游戏,为您提供最佳的游戏体验。但是一些游戏玩家报告了一个问题,即GeForceExperience没有优化他们系统上的游戏。只需执行这些简单的步骤即可在您的系统上解决此问题。修复1–为所有游戏使用最佳设置您可以设置为所有游戏使用最佳设置。1.在您的系统上打开GeForceExperience应用程序。2.GeForceExperience面
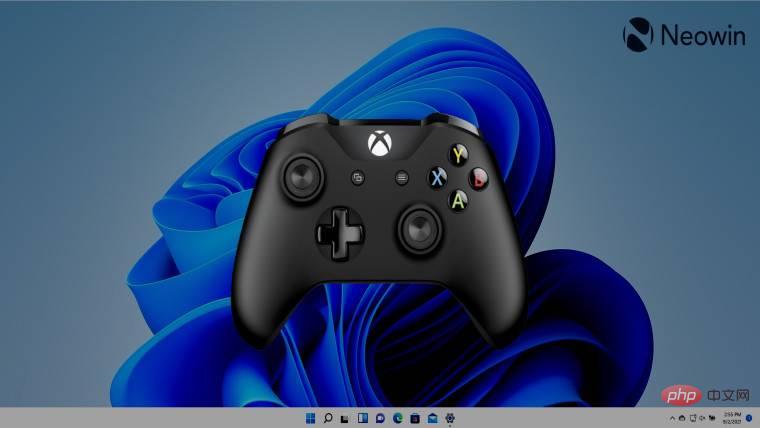
如果您在Windows机器上玩旧版游戏,您会很高兴知道Microsoft为它们计划了某些优化,特别是如果您在窗口模式下运行它们。该公司宣布,最近开发频道版本的内部人员现在可以利用这些功能。本质上,许多旧游戏使用“legacy-blt”演示模型在您的显示器上渲染帧。尽管DirectX12(DX12)已经利用了一种称为“翻转模型”的新演示模式,但Microsoft现在也正在向DX10和DX11游戏推出这一增强功能。迁移将改善延迟,还将为自动HDR和可变刷新率(VRR)等进一步增强打
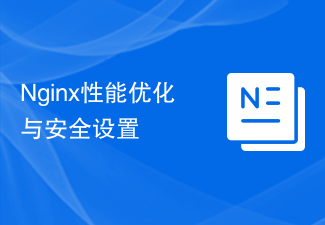
Nginx是一种常用的Web服务器,代理服务器和负载均衡器,性能优越,安全可靠,可以用于高负载的Web应用程序。在本文中,我们将探讨Nginx的性能优化和安全设置。一、性能优化调整worker_processes参数worker_processes是Nginx的一个重要参数。它指定了可以使用的worker进程数。这个值需要根据服务器硬件、网络带宽、负载类型等
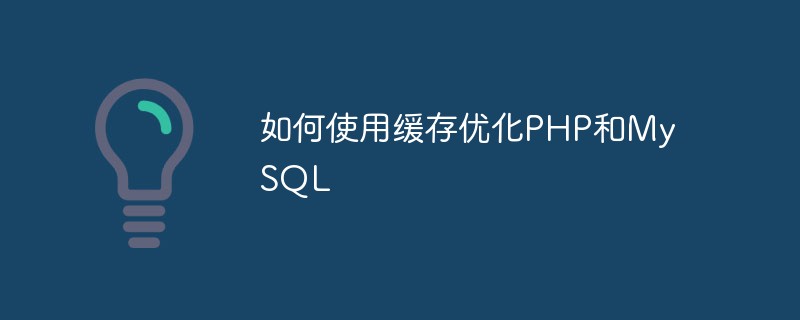
随着互联网的不断发展和应用的扩展,越来越多的网站和应用需要处理海量的数据和实现高流量的访问。在这种背景下,对于PHP和MySQL这样的常用技术,缓存优化成为了非常必要的优化手段。本文将在介绍缓存的概念及作用的基础上,从两个方面的PHP和MySQL进行缓存优化的实现,希望能够为广大开发者提供一些帮助。一、缓存的概念及作用缓存是指将计算结果或读取数据的结果缓存到
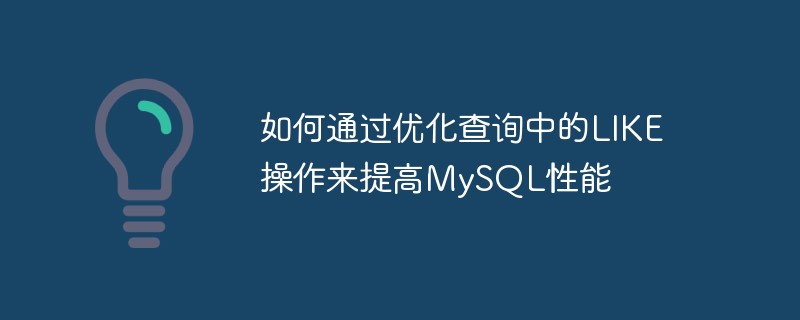
MySQL是目前最流行的关系型数据库之一,但是在处理大量数据时,MySQL的性能可能会受到影响。其中,一种常见的性能瓶颈是查询中的LIKE操作。在MySQL中,LIKE操作是用来模糊匹配字符串的,它可以在查询数据表时用来查找包含指定字符或者模式的数据记录。但是,在大型数据表中,如果使用LIKE操作,它会对数据库的性能造成影响。为了解决这个问题,我们可
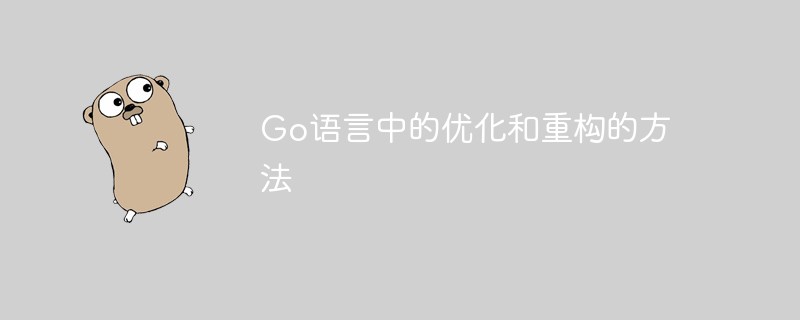
Go语言是一门相对年轻的编程语言,虽然从语言本身的设计来看,其已经考虑到了很多优化点,使得其具备高效的性能和良好的可维护性,但是这并不代表着我们在开发Go应用时不需要优化和重构,特别是在长期的代码积累过程中,原来的代码架构可能已经开始失去优势,需要通过优化和重构来提高系统的性能和可维护性。本文将分享一些在Go语言中优化和重构的方法,希望能够对Go开发者有所帮
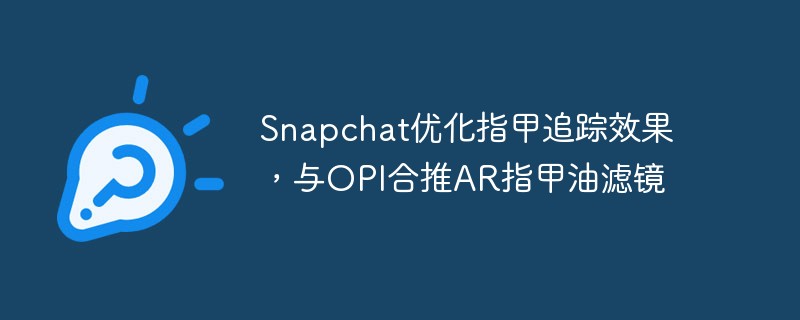
5月26日消息,SnapchatAR试穿滤镜技术升级,并与OPI品牌合作,推出指甲油AR试用滤镜。据悉,为了优化AR滤镜对手指甲的追踪定位,Snap在LensStudio中推出手部和指甲分割功能,允许开发者将AR图像叠加在指甲这种细节部分。据青亭网了解,指甲分割功能在识别到人手后,会给手部和指甲分别设置掩膜,用于渲染2D纹理。此外,还会识别用户个人指甲的底色,来模拟指甲油真实上手的效果。从演示效果来看,新的AR指甲油滤镜可以很好的模拟浅蓝磨砂质地。实际上,此前Snapchat曾推出AR指甲油试用
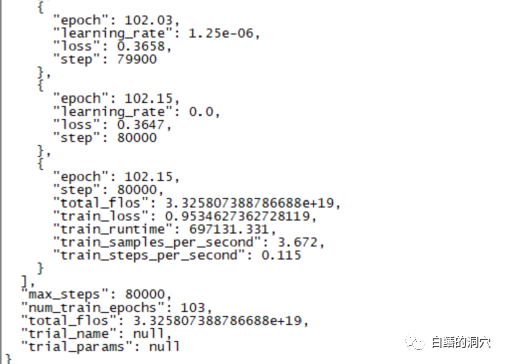
昨天一个跑了220个小时的微调训练完成了,主要任务是想在CHATGLM-6B上微调出一个能够较为精确的诊断数据库错误信息的对话模型来。不过这个等了将近十天的训练最后的结果令人失望,比起我之前做的一个样本覆盖更小的训练来,差的还是挺大的。这样的结果还是有点令人失望的,这个模型基本上是没有实用价值的。看样子需要重新调整参数与训练集,再做一次训练。大语言模型的训练是一场军备竞赛,没有好的装备是玩不起来的。看样子我们也必须要升级一下实验室的装备了,否则没有几个十天可以浪费。从最近的几次失败的微调训练来看


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

Atom editor mac version download
The most popular open source editor
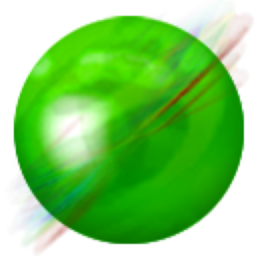
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Zend Studio 13.0.1
Powerful PHP integrated development environment
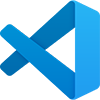
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft