Sharing of PHP search function optimization tips
PHP search function has always been a very important part of website development, because users often use the search box to find the information they need. However, many websites have problems such as low efficiency and inaccurate search results when implementing search functions. In order to help you optimize PHP search function, this article will share some tips and provide specific code examples.
1. Use full-text search engines
Traditional SQL databases are less efficient when processing large amounts of text content, so it is recommended to use full-text search engines, such as Elasticsearch, Solr, etc. These search engines provide faster and more accurate search results, while supporting full-text search, word segmentation and other functions. The following is a sample code that uses Elasticsearch to implement search functions:
require 'vendor/autoload.php'; use ElasticsearchClientBuilder; $client = ClientBuilder::create()->build(); $params = [ 'index' => 'your_index_name', 'type' => '_doc', 'body' => [ 'query' => [ 'match' => [ 'content' => 'search_keyword' ] ] ] ]; $response = $client->search($params); print_r($response);
2. Use indexes to speed up searches
Adding indexes to search fields can greatly improve search efficiency, especially for large data sets. In MySQL, you can add indexes through the following code:
CREATE INDEX index_name ON table_name (column_name);
3. Search results highlighted
In order to improve the user experience, you can highlight search keywords in the search results to allow users to Find the information you need more easily. The following is a simple highlighting example:
function highlight_keywords($text, $keywords) { foreach ($keywords as $keyword) { $text = str_ireplace($keyword, "<span style='background-color: yellow;'>$keyword</span>", $text); } return $text; }
4. Search results sorting
Search results can be sorted according to factors such as relevance, time, etc., so that users can see the most relevant content . The following is an example of sorting by relevance:
SELECT * FROM table_name WHERE MATCH(column_name) AGAINST('search_keyword' IN NATURAL LANGUAGE MODE) ORDER BY MATCH(column_name) AGAINST('search_keyword' IN NATURAL LANGUAGE MODE) DESC;
5. Asynchronous loading of search results
In order to improve the search experience, you can use AJAX technology to implement asynchronous loading of search results to avoid page refresh. The following is a simple asynchronous search example:
$('#search_box').keyup(function() { var keyword = $(this).val(); $.ajax({ url: 'search.php', method: 'POST', data: {keyword: keyword}, success: function(response) { $('#search_results').html(response); } }); });
Through the above tips and code examples, we can optimize the PHP search function, improve search efficiency and accuracy, and improve user experience. Hope these contents are helpful to you.
The above is the detailed content of Sharing of PHP search function optimization tips. For more information, please follow other related articles on the PHP Chinese website!
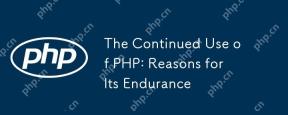
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
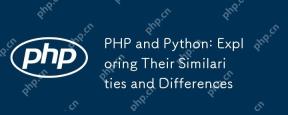
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
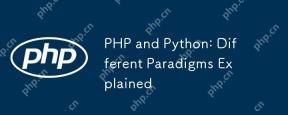
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
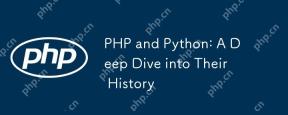
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
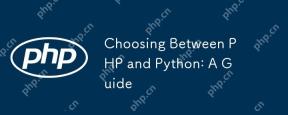
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
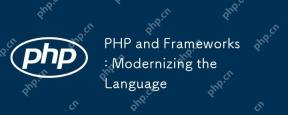
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
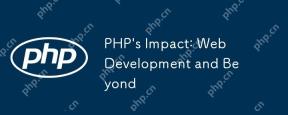
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
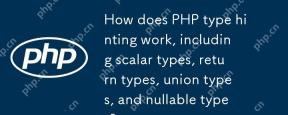
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
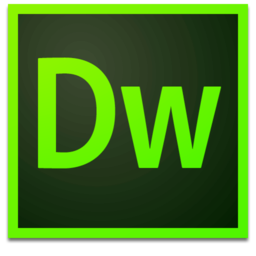
Dreamweaver Mac version
Visual web development tools
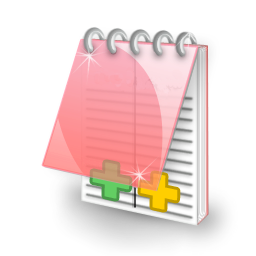
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.