Discuss the concept and application of macros in Golang
In Golang, macro (Macro) is an advanced programming technology that can help programmers simplify the code structure and improve the maintainability of the code. Macros are a source code level text replacement mechanism that replaces macro code snippets with actual code snippets during compilation. In this article, we will explore the concept and application of macros in Golang and provide specific code examples.
1. The concept of macros
In Golang, macros are not a natively supported feature, because the original design intention of Golang is to keep the language simple and clear. However, through code generation technology and the support of some third-party libraries, we can achieve macro-like functions. Macros allow programmers to define some code templates and then replace code snippets where needed, thereby simplifying the code writing process.
2. Application of macros
Below we will use a specific example to show how to implement the function of macros in Golang. Suppose we need to define a simple macro that generates and initializes slice variables of a specified type.
package main import "fmt" // 定义一个宏,用于生成指定类型的切片变量并初始化 func SliceMacro(t int, values ...int) []int { return append([]int{}, values...) } func main() { // 使用宏定义一个切片变量并初始化 slice := SliceMacro(1, 2, 3, 4, 5) // 打印切片内容 fmt.Println(slice) }
In the above example, we define a macro function named SliceMacro, which accepts a type parameter t and variable parameter values, and then returns and initializes a slice variable of the specified type. In the main function, we call the SliceMacro macro, generate an integer slice containing 1, 2, 3, 4, and 5, and print it out.
3. Precautions for macros
In the process of using macros, you need to pay attention to the following points:
- Macros will perform text replacement during the compilation process. Therefore, when using macros, make sure that the generated code conforms to the syntax specifications.
- Macros will increase the complexity and difficulty of understanding the code, so you must weigh the pros and cons when choosing to use macros.
- When using macros, avoid defining overly complex code templates to avoid reducing code readability and maintainability.
4. Summary
In this article, we explored the concept and application of macros in Golang and provided a simple code example. Through the use of macros, we can simplify the code writing process and improve the maintainability of the code. However, care needs to be taken when using macros to avoid overusing and overcomplicating the structure of your code. I hope this article can help readers better understand and apply macro techniques in Golang.
The above is the detailed content of Discuss the concept and application of macros in Golang. For more information, please follow other related articles on the PHP Chinese website!
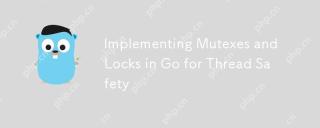
In Go, using mutexes and locks is the key to ensuring thread safety. 1) Use sync.Mutex for mutually exclusive access, 2) Use sync.RWMutex for read and write operations, 3) Use atomic operations for performance optimization. Mastering these tools and their usage skills is essential to writing efficient and reliable concurrent programs.
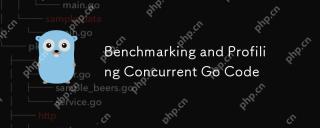
How to optimize the performance of concurrent Go code? Use Go's built-in tools such as getest, gobench, and pprof for benchmarking and performance analysis. 1) Use the testing package to write benchmarks to evaluate the execution speed of concurrent functions. 2) Use the pprof tool to perform performance analysis and identify bottlenecks in the program. 3) Adjust the garbage collection settings to reduce its impact on performance. 4) Optimize channel operation and limit the number of goroutines to improve efficiency. Through continuous benchmarking and performance analysis, the performance of concurrent Go code can be effectively improved.
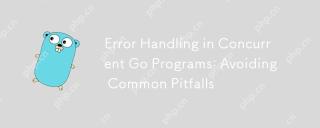
The common pitfalls of error handling in concurrent Go programs include: 1. Ensure error propagation, 2. Processing timeout, 3. Aggregation errors, 4. Use context management, 5. Error wrapping, 6. Logging, 7. Testing. These strategies help to effectively handle errors in concurrent environments.
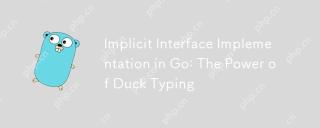
ImplicitinterfaceimplementationinGoembodiesducktypingbyallowingtypestosatisfyinterfaceswithoutexplicitdeclaration.1)Itpromotesflexibilityandmodularitybyfocusingonbehavior.2)Challengesincludeupdatingmethodsignaturesandtrackingimplementations.3)Toolsli
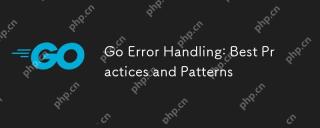
In Go programming, ways to effectively manage errors include: 1) using error values instead of exceptions, 2) using error wrapping techniques, 3) defining custom error types, 4) reusing error values for performance, 5) using panic and recovery with caution, 6) ensuring that error messages are clear and consistent, 7) recording error handling strategies, 8) treating errors as first-class citizens, 9) using error channels to handle asynchronous errors. These practices and patterns help write more robust, maintainable and efficient code.
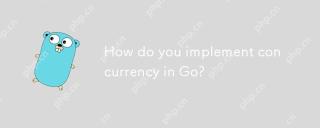
Implementing concurrency in Go can be achieved by using goroutines and channels. 1) Use goroutines to perform tasks in parallel, such as enjoying music and observing friends at the same time in the example. 2) Securely transfer data between goroutines through channels, such as producer and consumer models. 3) Avoid excessive use of goroutines and deadlocks, and design the system reasonably to optimize concurrent programs.
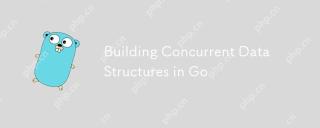
Gooffersmultipleapproachesforbuildingconcurrentdatastructures,includingmutexes,channels,andatomicoperations.1)Mutexesprovidesimplethreadsafetybutcancauseperformancebottlenecks.2)Channelsofferscalabilitybutmayblockiffullorempty.3)Atomicoperationsareef
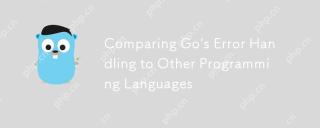
Go'serrorhandlingisexplicit,treatingerrorsasreturnedvaluesratherthanexceptions,unlikePythonandJava.1)Go'sapproachensureserrorawarenessbutcanleadtoverbosecode.2)PythonandJavauseexceptionsforcleanercodebutmaymisserrors.3)Go'smethodpromotesrobustnessand


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
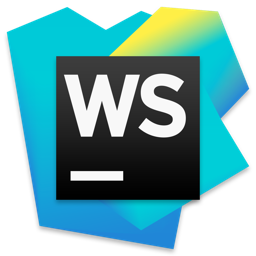
WebStorm Mac version
Useful JavaScript development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Zend Studio 13.0.1
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
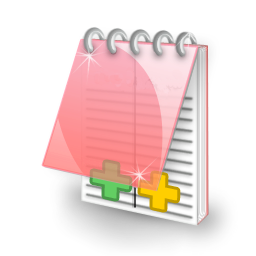
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
