


Getting Started with Java Developers: Mastering the Secrets of Interfaces and Abstract Classes Java is a programming language widely used in the field of software development. For beginners, mastering interfaces and abstract classes is very important basic knowledge. Interfaces and abstract classes are important concepts used to achieve polymorphism in Java. They can help developers better organize the code structure and improve the maintainability and scalability of the code. This article will delve into the definition, characteristics, and usage of interfaces and abstract classes to help Java developers better understand and apply these two concepts.
Interfaces and abstract classes are crucial concepts in the Java programming language. They enhance the reusability, scalability and maintainability of the code. This article will introduce the concepts of interfaces and abstract classes in a simple and easy-to-understand manner, supplemented by demonstration code to help Java beginners master their mysteries.
interface
Interface is an abstract type in Java that defines method signatures. It has no implementation and only defines the method name and parameter list. The interface acts as a contract for classes that wish to implement these methods.
Demo code:
public interface Animal { void makeSound(); void sleep(); }
advantage:
- Promote decoupling: Interfaces separate classes from implementation details, enhancing code reusability.
- Implement polymorphism: Different classes can implement the same interface, allowing dynamic binding and polymorphic behavior.
- Mandatory contract: The interface forces the implementation class to provide specified methods to ensure consistency and reliability.
defect:
- Cannot have implementation: The interface does not contain method implementation and can only define method signatures.
Abstract class
Abstract classes are classes that cannot be instantiated. It provides a public interface and implementation details for subclasses. Abstract classes can contain abstract methods (without implementation) and ordinary methods (with implementation).
Demo code:
public abstract class Shape { private String name; public abstract double getArea(); public final String getName() { return name; } }
advantage:
- Promote inheritance: Abstract classes provide a common foundation for subclasses, allowing code reuse and extension.
- Define partial implementation: Abstract classes can contain common methods, provide partial implementation, and reduce repeated code in subclasses.
- Forcing subclasses to implement: Abstract methods force subclasses to provide implementation to ensure consistency and reliability.
defect:
- Cannot be instantiated: The abstract class itself cannot be created as an object.
- Restrict flexibility: Abstract classes solidify part of the implementation and may limit the flexibility of subclasses.
Selection of interfaces and abstract classes
When choosing to use an interface or an abstract class, you need to consider the following factors:
- Reusability: If you need to use the same method signature in different classes, use interfaces.
- Inheritance: If implementation details need to be shared, use abstract classes.
- Flexibility: If greater flexibility is required, use an interface as it does not impose any implementation.
Collaborative work
Interfaces and abstract classes can work together to provide more powerful functionality. For example, an abstract class can implement an interface, providing partial implementation, while subclasses can further implement the remaining methods.
Example:
public abstract class Animal implements AnimalInterface { @Override public void makeSound() { // 提供默认实现 } // 子类必须实现 sleep() 方法 } public class Dog extends Animal { @Override public void sleep() { // 提供具体实现 } }
Summarize
Interfaces and abstract classes are indispensable tools in Java programming. Understanding how they work is critical to building reusable, scalable, and maintainable code. By combining the advantages of interfaces and abstract classes, Java developers can create more powerful applications.
The above is the detailed content of Getting Started with Java Developers: Mastering the Secrets of Interfaces and Abstract Classes. For more information, please follow other related articles on the PHP Chinese website!
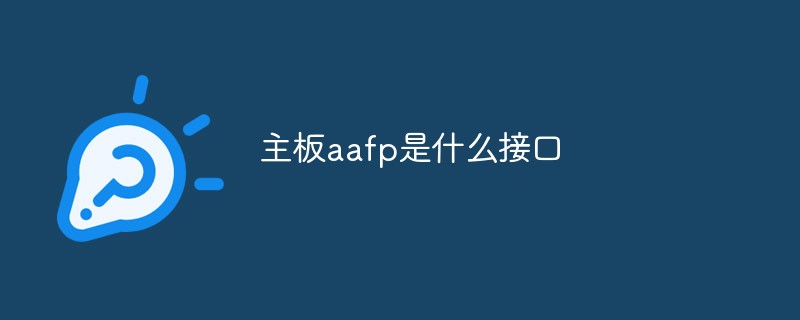
主板上的aafp是音频接口;该接口的功能是启用前面板的“3.5mm”插孔,起到传输音频的作用,aafp跳线基本上由两个部分组成,一部分是固定在主板、硬盘等设备上的,由两根或两根以上金属跳针组成,另一部分是跳线帽,是一个可以活动的组件,外层是绝缘塑料,内层是导电材料,可以插在跳线针上。
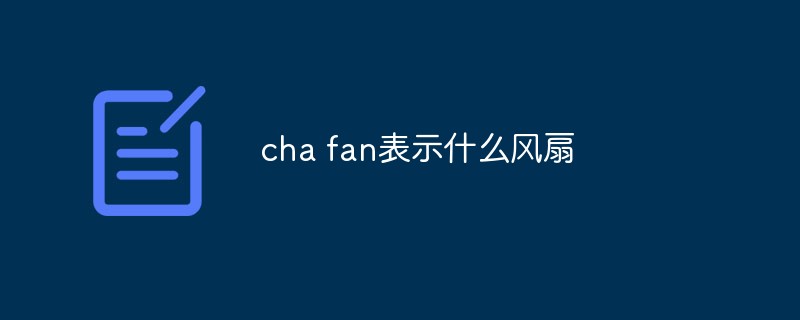
“cha fan”表示的是机箱风扇;“cha”是“chassis”的缩写,是机箱的意思,“cha fan”接口是主板上的风扇供电接口,用于连接主板与机箱风扇,可以配合温度传感器反馈的信息进行智能的转速调节、控制噪音。
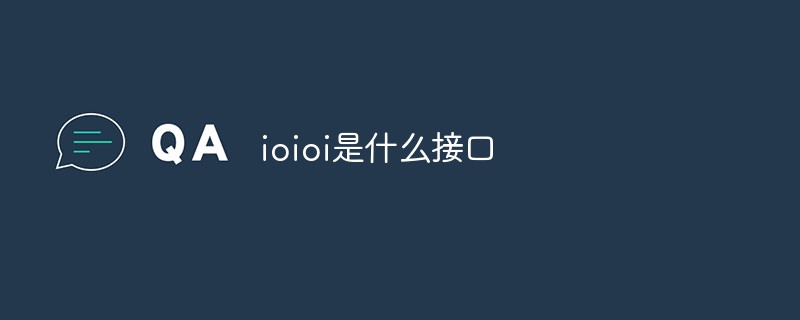
ioioi是指COM接口,即串行通讯端口,简称串口,是采用串行通信方式的扩展接口。COM接口是指数据一位一位地顺序传送;其特点是通信线路简单,只要一对传输线就可以实现双向通信(可以直接利用电话线作为传输线),从而大大降低了成本,特别适用于远距离通信,但传送速度较慢。
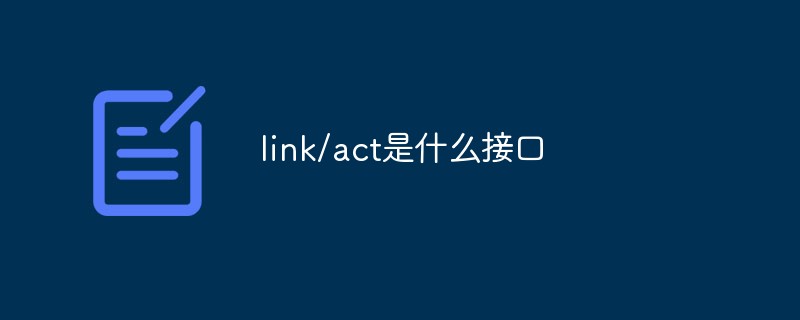
link/act是物理数据接口;交换机上的link/act指示灯表示线路是否连接或者活动的状态;通常Link/ACT指示灯用来观察线路是否激活或者通畅;一般情况下,若是线路畅通,则指示灯长亮,若是有数据传送时,则指示灯闪烁。
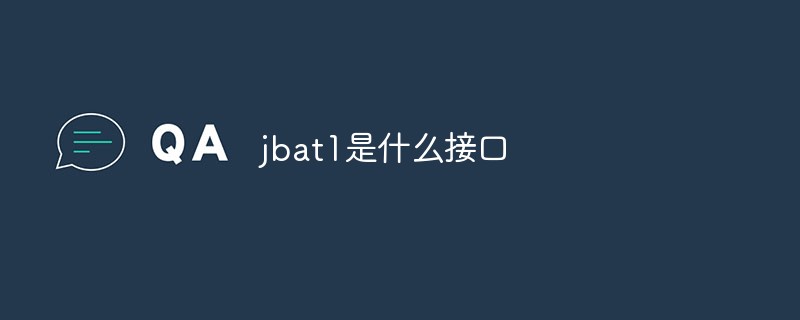
jbat1是主板电2113池放电跳线接口,对于现在市面上常见的主板来说,它们都设计有CMOS的放电跳线,让用户在操作时更加便捷,它也因此成为了CMOS最常见的放电方法。
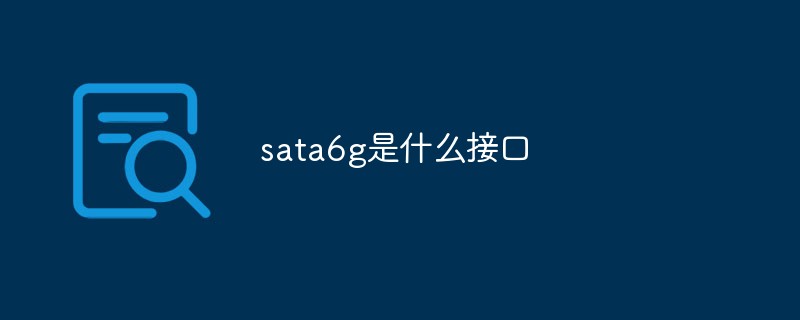
sata6g是数据传输速度为“6G/s”的sata接口;sata即“Serial ATA”,也就是串行ATA,是主板接口的名称,现在的硬盘和光驱都使用sata接口与主板相连,这个接口的规格目前已经发展到第三代sata3接口。
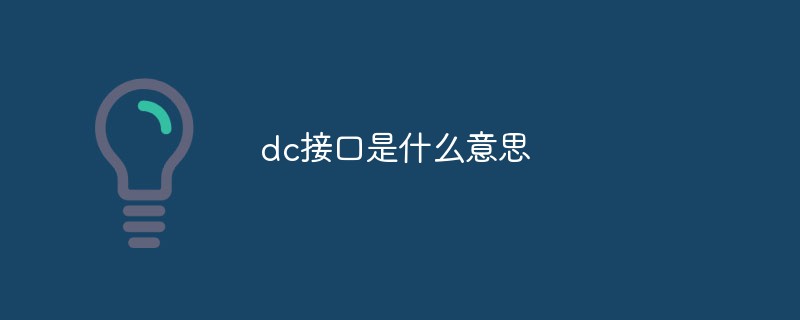
dc接口是一种为转变输入电压后有效输出固定电压接口的意思;dc接口是由横向插口、纵向插口、绝缘基座、叉形接触弹片、定向键槽组成,两只叉型接触弹片定位在基座中心部位,成纵横向排列互不相连,应用于手机、MP3、数码相机、便携式媒体播放器等产品中。
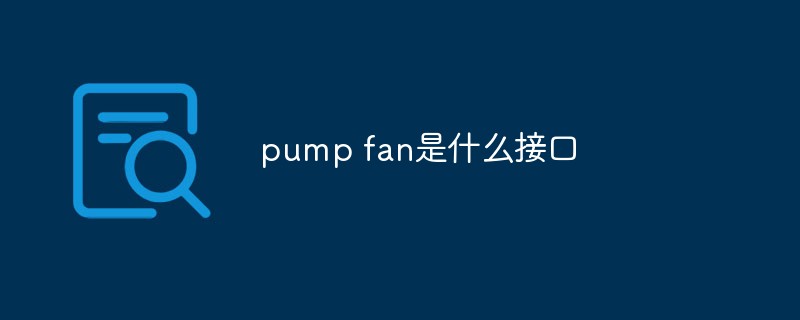
pump fan是散热风扇接口。主板上的风扇接口有cpu fun、sys fun、pump fun,对于一般普通用户来说区别不大,一般接哪个都行,而pump fun上的电流更大一点,用于接功率大一点的水冷风扇头。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Linux new version
SublimeText3 Linux latest version

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
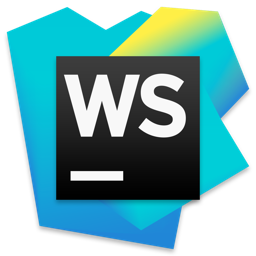
WebStorm Mac version
Useful JavaScript development tools
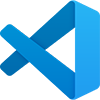
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
