Basic concepts and definitions of Golang arrays
In Golang, an array (Array) is a data structure of fixed length and the same type, used to store the same type sequence of elements. Arrays are value types, and elements are accessed by index, starting from 0. In Golang, the length of an array is part of the array type, which means that the length is part of the array type definition, so arrays of different lengths are actually arrays of different types.
Define array
In Golang, defining an array can be in the following format:
var variableName [length]dataType
Among them, variableName
represents the variable name of the array, length
represents the length of the array, dataType
represents the data type stored in the array.
The following is an example:
var arr [5]int
This line of code declares an array of length 5 that stores integer types. You can assign values to the array in the following ways:
arr = [5]int{1, 2, 3, 4, 5}
You can also not specify the length, and automatically infer the array length directly based on the length of the array content:
arr := [...]int{1, 2, 3, 4, 5}
Access the array elements
You can pass Indexing accesses the elements of the array, and the index starts from 0, as shown below:
fmt.Println(arr[0]) // 输出:1 fmt.Println(arr[2]) // 输出:3
Multidimensional array
Golang also supports multidimensional arrays, and can define two-dimensional, three-dimensional or even higher-dimensional arrays. The following is an example of a two-dimensional array:
var matrix [2][3]int matrix = [2][3]int{{1, 2, 3}, {4, 5, 6}}
A two-dimensional array with 2 rows and 3 columns is defined here and the values are initialized.
Traversal of arrays
You can use the for
loop to traverse the array, for example:
for i := 0; i < len(arr); i++ { fmt.Println(arr[i]) }
You can also use the range
keyword To traverse the array:
for _, value := range arr { fmt.Println(value) }
Notes
- In Golang, arrays are value types, and the entire array will be copied when assigned directly or passed as a parameter.
- The array length is part of the array type, so arrays of different lengths are arrays of different types.
- Using slices allows you to operate arrays more flexibly, because a slice is a reference to an array.
Through the brief introduction of this article, readers should have a preliminary understanding of the basic concepts and definitions of Golang arrays. In practical applications, rational use of arrays can process data more efficiently and improve code readability and performance.
References:
- https://golang.org/doc/effective_go.html
- https://tour.golang.org/arrays
I hope this article is helpful to you, thank you for reading!
The above is the detailed content of Basic concepts and definitions of Golang arrays. For more information, please follow other related articles on the PHP Chinese website!
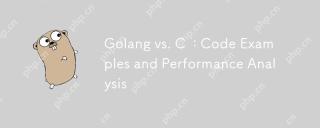
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
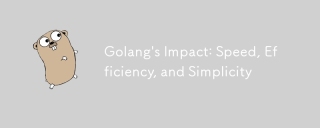
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
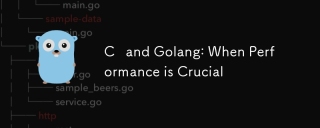
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
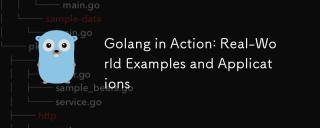
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
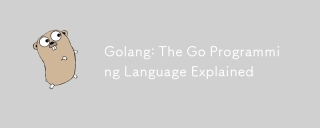
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
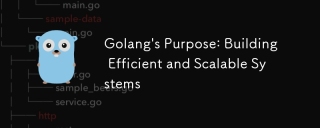
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
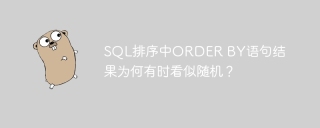
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
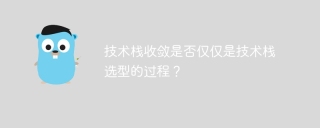
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Chinese version
Chinese version, very easy to use
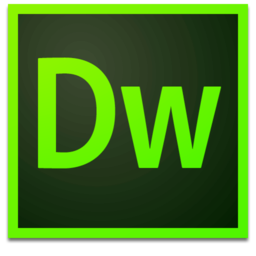
Dreamweaver Mac version
Visual web development tools