Security and efficiency analysis of cryptographic algorithms in Golang
In today’s digital society, data security issues have always attracted much attention. With the widespread popularity of Internet applications, the security and efficiency of cryptographic algorithms have become one of the important topics that software developers must study in depth. This article will focus on the security and efficiency of cryptographic algorithms in Golang, and show the implementation process through specific code examples, hoping to help readers gain a deeper understanding of this issue.
1. Selection of cryptographic algorithms
When selecting a cryptographic algorithm, we need to consider three aspects: security, efficiency and maintainability of the algorithm. Golang provides a series of cryptographic algorithms, such as AES, DES, RSA, etc. AES is a symmetric encryption algorithm that is widely used at present, and RSA is an asymmetric encryption algorithm. Different scenarios may require different cryptographic algorithms, and the appropriate algorithm needs to be selected based on comprehensive considerations based on the specific situation.
2. Example of cryptographic algorithm in Golang
The following is an example using the AES symmetric encryption algorithm, demonstrating how to encrypt and decrypt strings:
package main import ( "crypto/aes" "crypto/cipher" "crypto/rand" "encoding/hex" "fmt" "io" ) func encrypt(text, key []byte) string { block, err := aes.NewCipher(key) if err != nil { panic(err) } ciphertext := make([]byte, aes.BlockSize+len(text)) iv := ciphertext[:aes.BlockSize] if _, err := io.ReadFull(rand.Reader, iv); err != nil { panic(err) } stream := cipher.NewCFBEncrypter(block, iv) stream.XORKeyStream(ciphertext[aes.BlockSize:], text) return hex.EncodeToString(ciphertext) } func decrypt(text string, key []byte) string { ciphertext, _ := hex.DecodeString(text) block, err := aes.NewCipher(key) if err != nil { panic(err) } if len(ciphertext) < aes.BlockSize { panic("ciphertext too short") } iv := ciphertext[:aes.BlockSize] ciphertext = ciphertext[aes.BlockSize:] stream := cipher.NewCFBDecrypter(block, iv) stream.XORKeyStream(ciphertext, ciphertext) return string(ciphertext) } func main() { text := "Hello, World!" key := []byte("supersecretkey") encrypted := encrypt([]byte(text), key) fmt.Println("Encrypted:", encrypted) decrypted := decrypt(encrypted, key) fmt.Println("Decrypted:", decrypted) }
The above code In the example, two functions, encrypt and decrypt, are first defined to encrypt and decrypt strings respectively. In the main function, we define a string text to be encrypted and a key, then encrypt the text through the encrypt function, and then decrypt the encrypted string through the decrypt function and output the result.
3. Security and Efficiency Analysis
In practical applications, the security of data encryption is crucial. AES is a widely recognized encryption algorithm that is highly secure and efficient. By using keys of appropriate length, AES can provide adequate security while also performing well in terms of performance. However, it should be noted that the management and storage of keys are also key links in ensuring encryption security.
In addition, pay attention to avoid using known weak passwords and encryption algorithms, and regularly update keys and other measures to improve data security. For some scenarios with extremely high security requirements, it may be necessary to combine multiple encryption algorithms to improve security.
In short, the cryptographic algorithms in Golang provide a wealth of choices. Developers need to choose the appropriate algorithm according to actual needs and strictly follow security best practices to ensure a balance between data security and efficiency. I hope this article will be helpful to readers in cryptographic algorithms.
The above is the detailed content of Security and efficiency analysis of cryptographic algorithms in Golang. For more information, please follow other related articles on the PHP Chinese website!
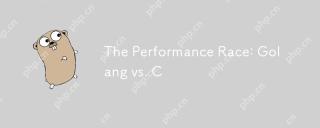
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
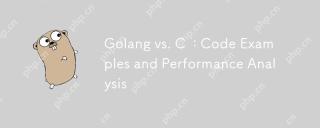
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
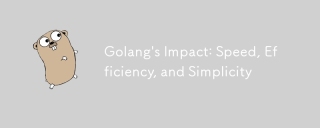
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
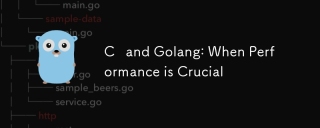
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
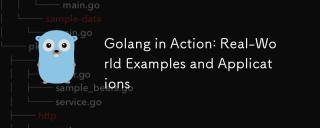
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
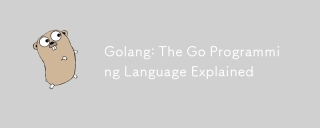
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
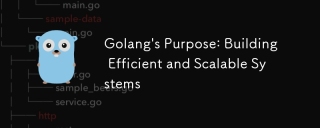
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
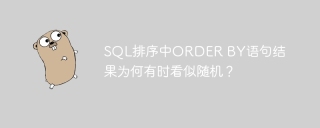
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
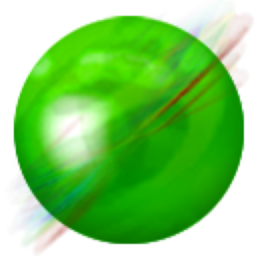
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
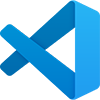
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft