There are many ways to implement concurrency thousands of requests in python. The following are some commonly used methods:
- Using Multi-threading: You can use the
threading
module to create and manage multiple threads to send requests concurrently. Each thread can be responsible for sending a request. You can use Thread Pool to manage and control the number of threads.
import threading import requests def send_request(url): response = requests.get(url) print(response.text) urls = [...]# 存储要发送请求的URL列表 threads = [] for url in urls: thread = threading.Thread(target=send_request, args=(url,)) thread.start() threads.append(thread) for thread in threads: thread.join()
- Use coroutines: You can use the
async<strong class="keylink">io</strong>
module and the<strong class="keylink">ai</strong>o<strong class="keylink">Http</strong>
library to achieve concurrency ask. A coroutine is a lightweight thread that can achieve concurrency in a single thread. By using theasync
andawait
keywords, you can create asynchronous functions to execute requests concurrently.
import asyncio import aiohttp async def send_request(url): async with aiohttp.ClientSession() as session: async with session.get(url) as response: data = await response.text() print(data) urls = [...]# 存储要发送请求的URL列表 loop = asyncio.get_event_loop() tasks = [send_request(url) for url in urls] loop.run_until_complete(asyncio.wait(tasks)) loop.close()
- Use concurrency library: You can use some third-party concurrency libraries, such as
grequests
orgevent
, to implement concurrent requests. These libraries can execute multiple requests concurrently in a single thread.
Example using grequests
library:
import grequests urls = [...]# 存储要发送请求的URL列表 requests = [grequests.get(url) for url in urls] responses = grequests.map(requests) for response in responses: print(response.text)
Example using gevent
library:
import gevent import requests def send_request(url): response = requests.get(url) print(response.text) urls = [...]# 存储要发送请求的URL列表 greenlets = [gevent.spawn(send_request, url) for url in urls] gevent.joinall(greenlets)
No matter which method you choose, be careful to control the number of concurrent requests to avoid excessive resource consumption or server overloading.
The above is the detailed content of How to concurrently send thousands of requests in python. For more information, please follow other related articles on the PHP Chinese website!
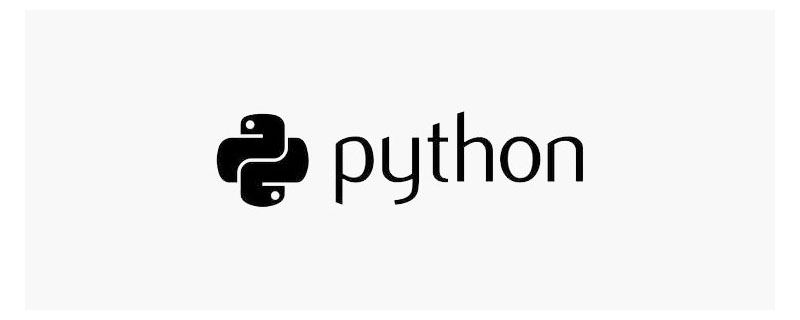
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于Seaborn的相关问题,包括了数据可视化处理的散点图、折线图、条形图等等内容,下面一起来看一下,希望对大家有帮助。
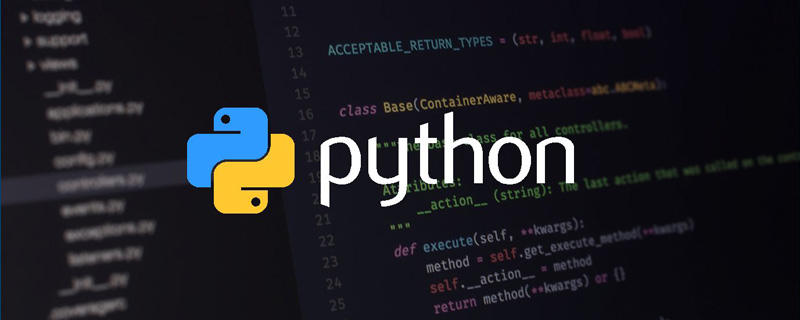
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于进程池与进程锁的相关问题,包括进程池的创建模块,进程池函数等等内容,下面一起来看一下,希望对大家有帮助。
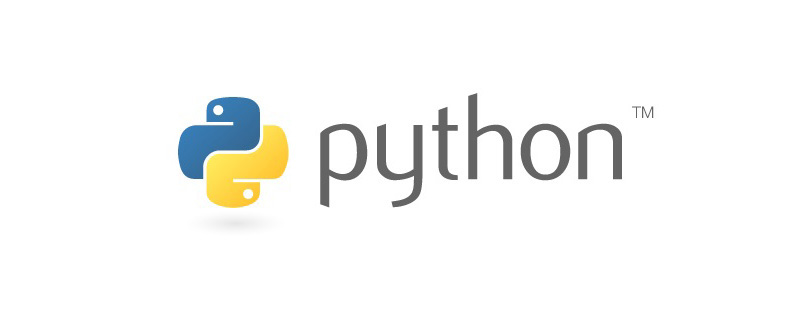
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于简历筛选的相关问题,包括了定义 ReadDoc 类用以读取 word 文件以及定义 search_word 函数用以筛选的相关内容,下面一起来看一下,希望对大家有帮助。
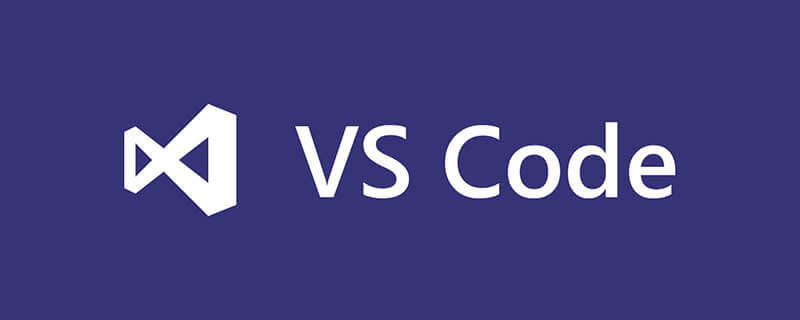
VS Code的确是一款非常热门、有强大用户基础的一款开发工具。本文给大家介绍一下10款高效、好用的插件,能够让原本单薄的VS Code如虎添翼,开发效率顿时提升到一个新的阶段。
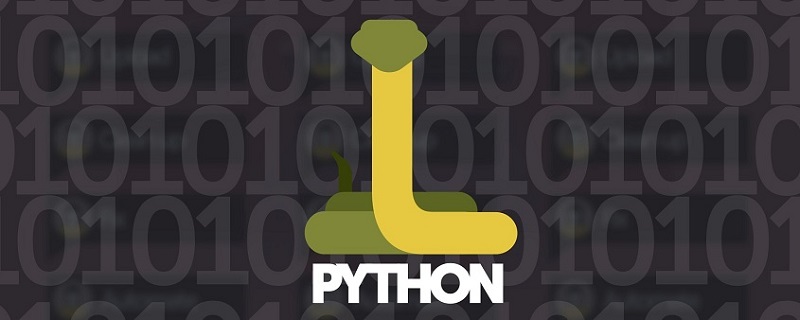
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于数据类型之字符串、数字的相关问题,下面一起来看一下,希望对大家有帮助。
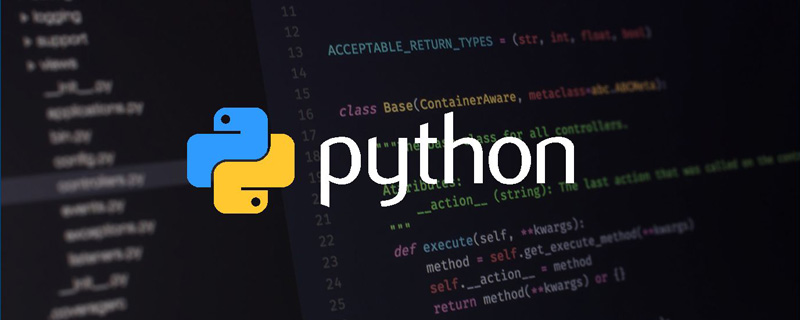
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于numpy模块的相关问题,Numpy是Numerical Python extensions的缩写,字面意思是Python数值计算扩展,下面一起来看一下,希望对大家有帮助。
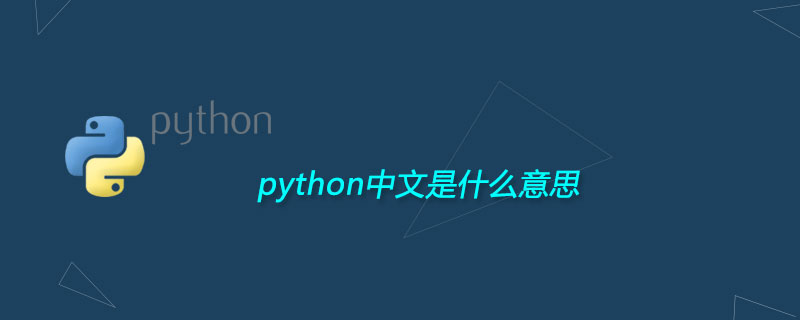
pythn的中文意思是巨蟒、蟒蛇。1989年圣诞节期间,Guido van Rossum在家闲的没事干,为了跟朋友庆祝圣诞节,决定发明一种全新的脚本语言。他很喜欢一个肥皂剧叫Monty Python,所以便把这门语言叫做python。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
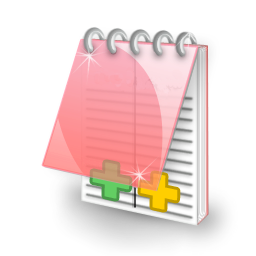
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
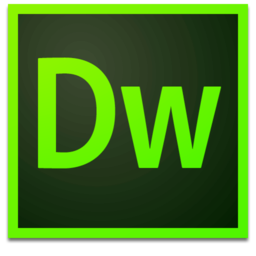
Dreamweaver Mac version
Visual web development tools

Atom editor mac version download
The most popular open source editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
