AngularJS 1.2 makes it easier to create page-to-page transitions in a single-page application by introducing pure CSS class-based transitions and animations. Using just an ng-view, let's take a look at a scalable approach that introduces numerous different transitions and how each page can be transitioned in and out.
Demo: http://embed.plnkr.co/PqhvmW/preview
First, tag:
<div class="page-container"> <div ng-view class="page-view" ng-class="pageAnimationClass"> </div> </div>
'go' method
In a single-page application, we still want to enable navigation via URLs and ensure that the browser's back and next buttons work as expected. So once we set up our routes, templates, and controllers (optional parsing) in $routeProvider, we can use a relative path in an ng-click to switch pages directly:
<a ng-click="/page2">Go to page 2</a>
That also works, but we need to hardcode a class switch in ng-view. Instead, let's create a 'go' method on $rootScope that lets us specify a path and a toggle like this:
<a ng-click="go('/page2', 'slideLeft')">Go to page 2</a>
This is our $rootScope 'go' method:
$rootScope.go = function (path, pageAnimationClass) { if (typeof(pageAnimationClass) === 'undefined') { // Use a default, your choice $rootScope.pageAnimationClass = 'crossFade'; } else { // Use the specified animation $rootScope.pageAnimationClass = pageAnimationClass; } if (path === 'back') { // Allow a 'back' keyword to go to previous page $window.history.back(); } else { // Go to the specified path $location.path(path); } };
Now, any toggle class you specified as the second parameter will be added to ng-view and the go method will change the page path with the specified first parameter.
Switch class
The next thing to do is to create any number of toggle classes and use the hooks provided by the ngAnimate module , for example:
/* slideLeft */ .slideLeft { transition-timing-function: ease; transition-duration: 250ms; } .slideLeft.ng-enter { transition-property: none; transform: translate3d(100%,0,0); } .slideLeft.ng-enter.ng-enter-active { transition-property: all; transform: translate3d(0,0,0); } .slideLeft.ng-leave { transition-property: all; transform: translate3d(0,0,0); } .slideLeft.ng-leave.ng-leave-active { transition-property: all; transform: translate3d(-100%,0,0); }
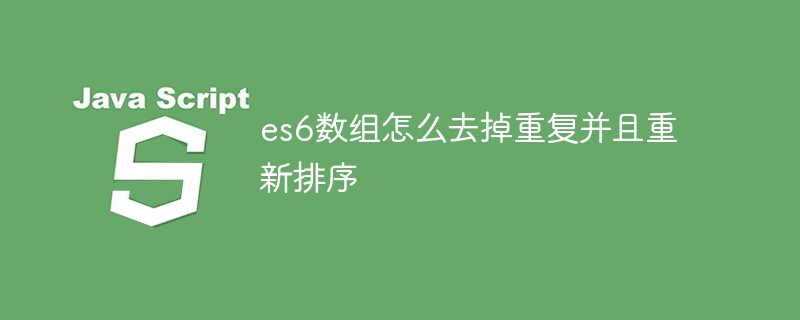
去掉重复并排序的方法:1、使用“Array.from(new Set(arr))”或者“[…new Set(arr)]”语句,去掉数组中的重复元素,返回去重后的新数组;2、利用sort()对去重数组进行排序,语法“去重数组.sort()”。
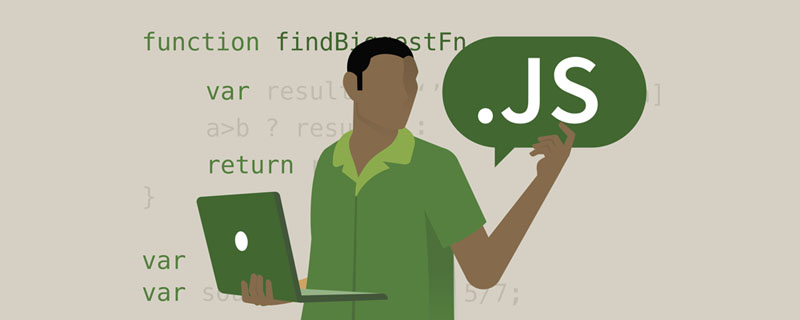
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于Symbol类型、隐藏属性及全局注册表的相关问题,包括了Symbol类型的描述、Symbol不会隐式转字符串等问题,下面一起来看一下,希望对大家有帮助。
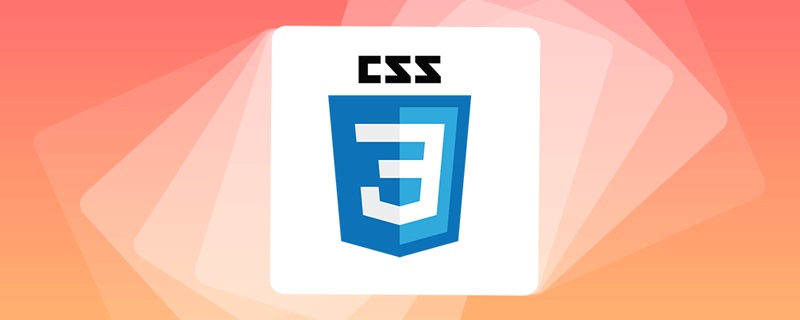
怎么制作文字轮播与图片轮播?大家第一想到的是不是利用js,其实利用纯CSS也能实现文字轮播与图片轮播,下面来看看实现方法,希望对大家有所帮助!
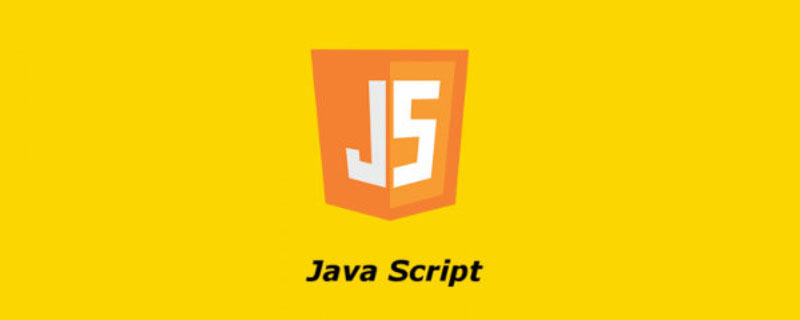
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于对象的构造函数和new操作符,构造函数是所有对象的成员方法中,最早被调用的那个,下面一起来看一下吧,希望对大家有帮助。
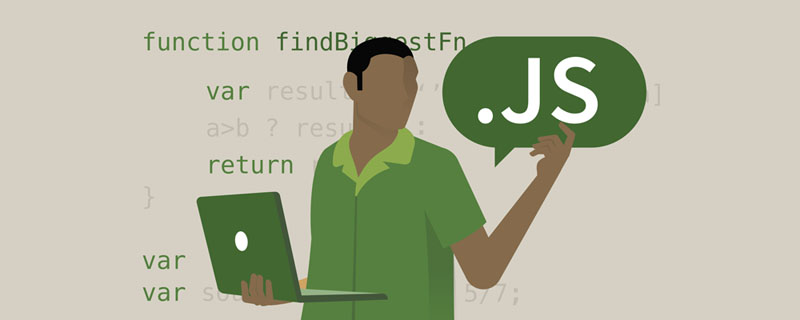
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于面向对象的相关问题,包括了属性描述符、数据描述符、存取描述符等等内容,下面一起来看一下,希望对大家有帮助。
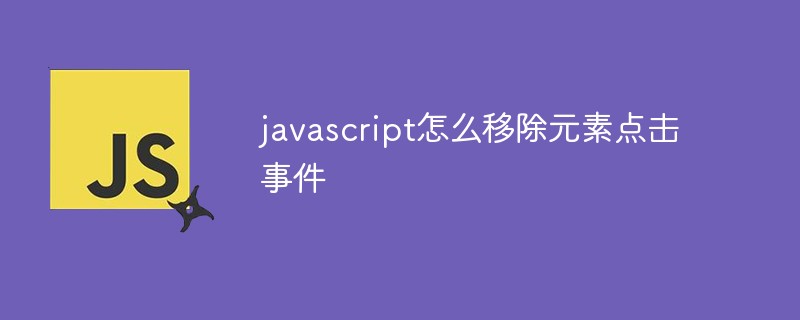
方法:1、利用“点击元素对象.unbind("click");”方法,该方法可以移除被选元素的事件处理程序;2、利用“点击元素对象.off("click");”方法,该方法可以移除通过on()方法添加的事件处理程序。
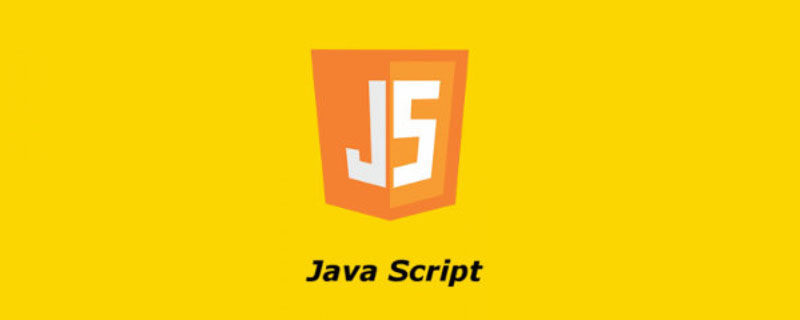
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于BOM操作的相关问题,包括了window对象的常见事件、JavaScript执行机制等等相关内容,下面一起来看一下,希望对大家有帮助。
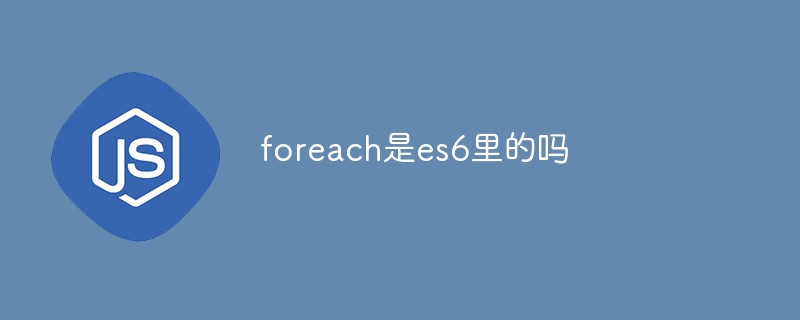
foreach不是es6的方法。foreach是es3中一个遍历数组的方法,可以调用数组的每个元素,并将元素传给回调函数进行处理,语法“array.forEach(function(当前元素,索引,数组){...})”;该方法不处理空数组。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
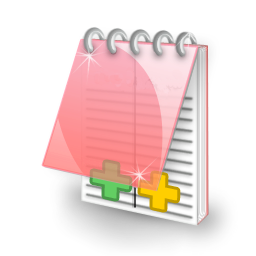
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Chinese version
Chinese version, very easy to use

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
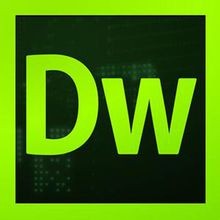
Dreamweaver CS6
Visual web development tools
