php editor Zimo takes you to reveal the secret weapon behind Java JAX-RS. Java JAX-RS is a Java API for building RESTful Web services. It provides a powerful set of tools and frameworks that allow developers to quickly and efficiently build and deploy Web services. In this article, we will delve into the core concepts, functional features and practical applications of Java JAX-RS to help you better understand and utilize this powerful technology. Let's uncover the secrets behind Java JAX-RS and explore its endless possibilities!
JAX-RS, part of the Java EE platform, provides a rich set of capabilities and features, making it an ideal choice for developing RESTful api. In addition to ease of use, high performance, and scalability, JAX-RS has the following secret sauce:
1. Annotation-driven development
JAX-RS adopts an annotation-driven development model, allowing you to use Java annotations to declare WEB resources, Http methods and parameters. This declarative approach simplifies API development, reduces boilerplate code, and improves readability and maintainability.
The following code demonstrates a RESTful service using JAX-RS annotations:
@Path("/todos") public class TodoResource { @GET public List<Todo> getTodos() { // 获取所有待办事项 } @POST public Todo createTodo(Todo todo) { // 创建一个新待办事项 } }
2. RESTful service documentation
JAX-RS integrates frameworks such as swagger and OpenAPI, allowing you to use annotations to generate documentation for RESTful APIs. These documents provide developers and external systems with a detailed description of the API, including endpoints, request and response formats.
The following code demonstrates using Swagger annotations to generate documentation for a RESTful service:
@Api(value = "Todo API", description = "RESTful API for managing todos") @Path("/todos") public class TodoResource { // ... }
3. Message body processing
JAX-RS supports a variety of message body handlers, allowing you to easily handle requests and responses in JSON, XML, and other formats. These handlers can automatically parse and serialize the message body, simplifying API development.
The following code demonstrates using the Jackson jsON handler to handle JSON requests:
@POST @Consumes("application/json") public void createTodo(@RequestBody Todo todo) { // 使用 Jackson 解析 JSON 请求并创建 Todo 对象 }
4. Client API
JAX-RS provides a client API that allows you to easily create clients for RESTful services. The client API provides all the functionality needed to interact with the server, including HTTP requests, response handling, and exception handling.
The following code demonstrates using the client API to call a RESTful service:
Client client = ClientBuilder.newClient(); WebTarget target = client.target("http://localhost:8080/todos"); Response response = target.request().get(); List<Todo> todos = response.readEntity(new GenericType<List<Todo>>() {});
5. Scalability and flexibility
JAX-RS's modular design and extensible architecture allows you to customize and extend the API as needed. You can enhance the capabilities of JAX-RS by creating custom providers, interceptors, and filters to meet specific needs.
The following code demonstrates how to create a custom provider to handle custom media types:
@Provider @Consumes("application/custom-media-type") public class CustomMediaTypeProvider implements MessageBodyReader<CustomObject> { // ... }
in conclusion
JAX-RS is a powerful Java library for developing RESTful web services. By leveraging its secret sauce, including annotation-driven development, RESTful service documentation, message body handling, client APIs, and extensibility, you can build efficient, maintainable, and feature-rich RESTful APIs. By embracing the power of JAX-RS, you can create seamless web experiences for your applications and users.
The above is the detailed content of Uncovering the secret weapon behind Java JAX-RS. For more information, please follow other related articles on the PHP Chinese website!
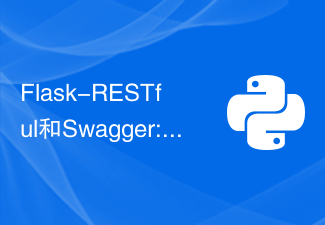
Flask-RESTful和Swagger:Pythonweb应用程序中构建RESTfulAPI的最佳实践(第二部分)在上一篇文章中,我们探讨了如何使用Flask-RESTful和Swagger来构建RESTfulAPI的最佳实践。我们介绍了Flask-RESTful框架的基础知识,并展示了如何使用Swagger来构建RESTfulAPI的文档。本
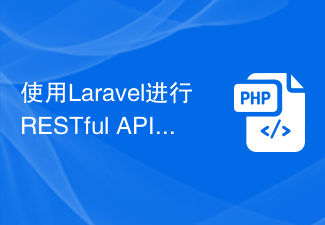
使用Laravel进行RESTfulAPI开发:构建现代化的Web服务随着互联网的快速发展,Web服务的需求日益增加。而RESTfulAPI作为一种现代化的Web服务架构方式,具备轻量、灵活、易扩展的特点,因此在Web开发中得到了广泛应用。在本文中,我们将介绍如何使用Laravel框架来构建一个现代化的RESTfulAPI。Laravel是PHP语言中
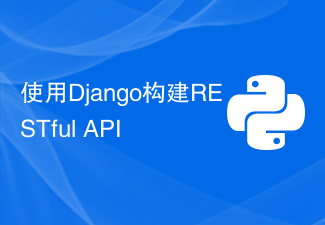
Django是一个Web框架,可以轻松地构建RESTfulAPI。RESTfulAPI是一种基于Web的架构,可以通过HTTP协议访问。在这篇文章中,我们将介绍如何使用Django来构建RESTfulAPI,包括如何使用DjangoREST框架来简化开发过程。安装Django首先,我们需要在本地安装Django。可以使用pip来安装Django,具体
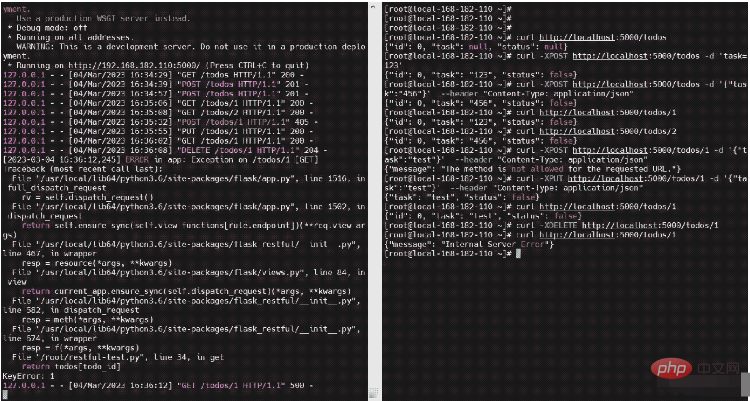
一、RESTful概述REST(RepresentationalStateTransfer)风格是一种面向资源的Web应用程序设计风格,它遵循一些设计原则,使得Web应用程序具有良好的可读性、可扩展性和可维护性。下面我们来详细解释一下RESTful风格的各个方面:资源标识符:在RESTful风格中,每个资源都有一个唯一的标识符,通常是一个URL(UniformResourceLocator)。URL用于标识资源的位置,使得客户端可以使用HTTP协议进行访问。例如,一个简单的URL可以是:http
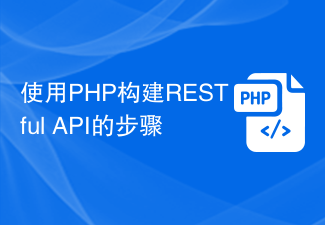
随着互联网的发展和普及,Web应用程序和移动应用程序越来越普遍。这些应用程序需要与后端服务器进行通信,并获取或提交数据。在过去,常规的通信方式是使用SOAP(简单对象访问协议)或XML-RPC(XML远程过程调用)。然而,随着时间的推移,这些协议被认为过于笨重和复杂。现代应用程序需要更加轻巧和直接的API来进行通信。RESTfulAPI(表现层状态转化AP
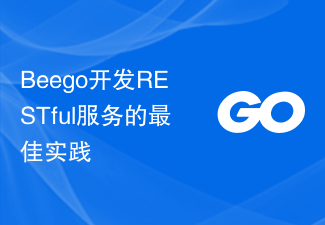
在当下信息技术不断创新的环境下,RESTful架构风靡于各种常用的WebAPI应用之中,成为了新兴的服务开发趋势。而Beego框架作为Golang中一款高性能、易扩展的Web框架,出于其高效、易用、灵活等优点,被广泛应用于RESTful服务的开发中。下文将从Beego开发RESTful服务的最佳实践的角度,为广大的开发者提供一些参考。一、路由设计在REST
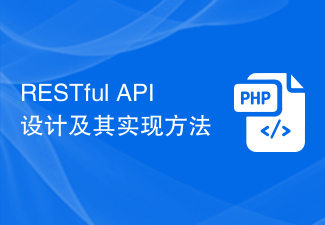
RESTfulAPI是目前Web架构中较为常用的一种API设计风格,它的设计理念是基于HTTP协议的标准方法来完成Web资源的表示与交互。在实现过程中,RESTfulAPI遵循一系列规则和约束,包括可缓存、服务器-客户端分离、无状态性等,这些规则保证了API的可维护性、扩展性、安全性以及易用性。接下来,本文将详细介绍RESTfulAPI的设计及其实现方
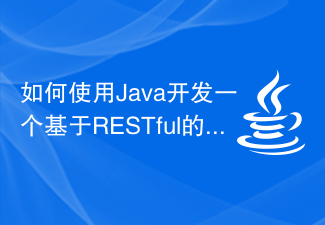
如何使用Java开发一个基于RESTful的APIRESTful是一种基于HTTP协议的架构风格,通过使用HTTP协议的GET、POST、PUT、DELETE等方法来实现对资源的操作。在Java开发中,可以使用一些框架来简化RESTfulAPI的开发过程,如SpringMVC、Jersey等。本文将向您详细介绍如何使用Java开发一个基于RESTful的


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
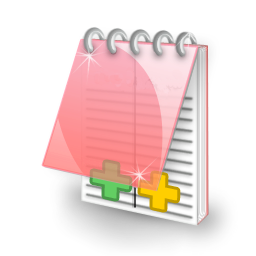
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Linux new version
SublimeText3 Linux latest version

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
