


Golang practice: common problems and solutions for file modification operations
With the continuous development and widespread application of Go language (Golang), processing file operations is also one of the common needs in development. However, when performing file modification operations, you sometimes encounter various problems, such as file read and write permissions, file non-existence, file locks, etc. This article will focus on these common problems and provide solutions and related Golang code examples.
1. File read and write permission issues
When performing file modification operations, we often encounter the problem of insufficient file read and write permissions. At this time, we need to ensure that the program has appropriate read and write permissions on the file.
Solution:
We can use the os.OpenFile
function to open the file in write mode and set the appropriate permissions when opening the file.
package main import ( "os" ) func main() { filepath := "example.txt" f, err := os.OpenFile(filepath, os.O_WRONLY|os.O_CREATE, 0666) if err != nil { panic(err) } defer f.Close() // 文件写入操作 }
2. The file does not exist
When trying to modify a file that does not exist, an error will occur in the program. How to correctly handle the situation where the file does not exist?
Solution:
We can use the os.Stat
function to determine whether the file exists, and if it does not exist, we can create the file.
package main import ( "os" ) func main() { filepath := "example.txt" if _, err := os.Stat(filepath); os.IsNotExist(err) { file, err := os.Create(filepath) if err != nil { panic(err) } defer file.Close() } // 文件写入操作 }
3. File lock problem
When multiple coroutines or processes modify the same file at the same time, file lock problems may occur, which may lead to data inconsistency or program crash. How to use file locks correctly in Golang?
Solution:
We can use the syscall.Flock
function to lock the file to ensure that only one process can access the file when performing file modification operations.
package main import ( "os" "syscall" ) func main() { filepath := "example.txt" file, err := os.OpenFile(filepath, os.O_WRONLY|os.O_CREATE, 0666) if err != nil { panic(err) } defer file.Close() if err := syscall.Flock(int(file.Fd()), syscall.LOCK_EX); err != nil { panic(err) } defer syscall.Flock(int(file.Fd()), syscall.LOCK_UN) // 文件写入操作 }
The above is an introduction and code examples about common problems and solutions in Golang file modification operations. When dealing with file operations, we should always pay attention to issues such as file permissions, file existence, and file locks to ensure that the program can correctly perform file modification operations. Hope this article is helpful to you.
The above is the detailed content of Golang practice: common problems and solutions for file modification operations. For more information, please follow other related articles on the PHP Chinese website!
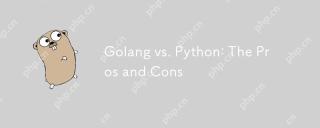
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
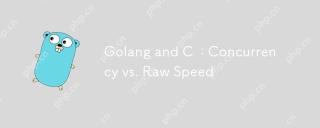
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
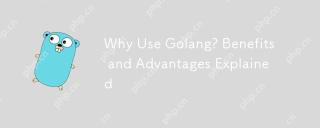
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
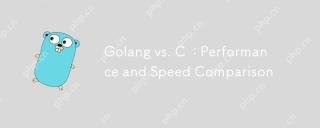
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
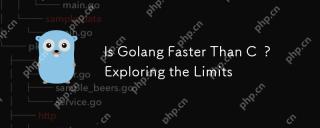
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
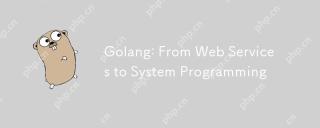
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
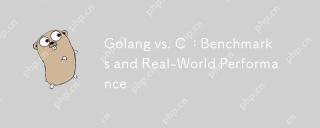
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
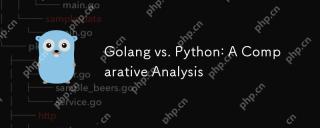
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
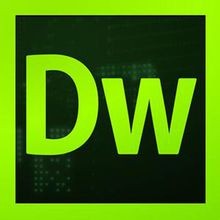
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Zend Studio 13.0.1
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool