File editing tool written in Go language
File modification program written in Golang
In recent years, Golang has been favored by developers as an efficient and concise programming language. Its powerful concurrency capabilities and easy-to-learn characteristics make it one of the first choices of many developers. In daily development, reading, modifying and saving files are often involved. This article will introduce how to use Golang to write a file modification program by reading the file content, modifying it and saving the new file.
Step 1: Import the necessary packages
Before writing the file modification program, you first need to import the standard library that handles file operations in Golang:
package main import ( "fmt" "io/ioutil" "os" )
Step 2: Define the file Modify function
Next, we define a function to read the file content and perform corresponding modification operations. In this example, we will read a text file, convert the uppercase characters in it to lowercase characters, and save it as a new file.
func modifyFile(inputFile, outputFile string) error { // 读取原文件内容 data, err := ioutil.ReadFile(inputFile) if err != nil { return err } // 将大写字母转换为小写字母 for i, b := range data { if 'A' <= b && b <= 'Z' { data[i] += 'a' - 'A' } } // 将修改后的内容写入新文件 err = ioutil.WriteFile(outputFile, data, 0644) if err != nil { return err } return nil }
Step 3: Main function call
Finally, we write the main function, call the file modification function defined above, and pass in the paths of the input file and output file:
func main() { inputFile := "input.txt" outputFile := "output.txt" err := modifyFile(inputFile, outputFile) if err != nil { fmt.Println("文件修改失败:", err) return } fmt.Println("文件修改成功!") }
Complete code:
package main import ( "fmt" "io/ioutil" ) func modifyFile(inputFile, outputFile string) error { data, err := ioutil.ReadFile(inputFile) if err != nil { return err } for i, b := range data { if 'A' <= b && b <= 'Z' { data[i] += 'a' - 'A' } } err = ioutil.WriteFile(outputFile, data, 0644) if err != nil { return err } return nil } func main() { inputFile := "input.txt" outputFile := "output.txt" err := modifyFile(inputFile, outputFile) if err != nil { fmt.Println("文件修改失败:", err) return } fmt.Println("文件修改成功!") }
With the above code, we can implement a simple file modification program to convert uppercase letters in the file to lowercase letters and save it as a new file. This example is just a simple demonstration. In actual applications, more complex file content modification operations can be performed according to requirements. I hope this article will be helpful to you and allow you to better master Golang programming.
The above is the detailed content of File editing tool written in Go language. For more information, please follow other related articles on the PHP Chinese website!
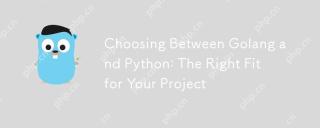
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
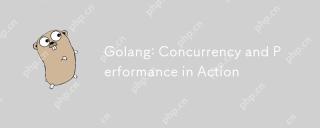
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
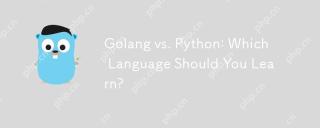
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
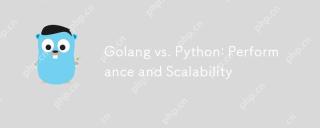
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
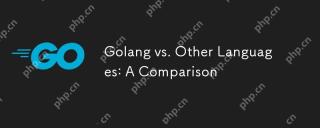
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
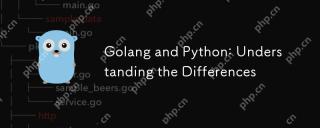
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
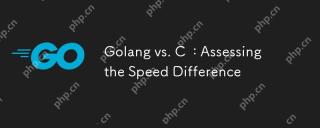
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
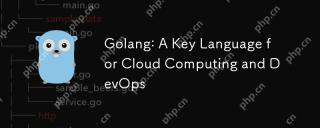
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
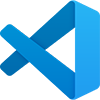
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
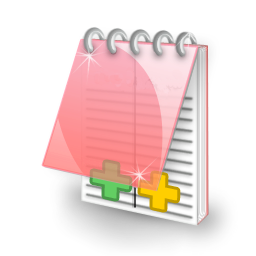
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use