How to call the quick sort function to sort data in Java requires specific code examples
Quick sort is a commonly used sorting algorithm when processing large-scale data Has higher efficiency. Calling the quick sort function in Java to sort data can be implemented recursively. The following will introduce you in detail how to perform quick sorting in Java and provide specific code examples.
First of all, we need to understand the principle of quick sort. The basic idea of quick sort is to select a benchmark element and divide the sequence to be sorted into two parts through one sorting pass. The elements in one part are smaller than the benchmark element, and the elements in the other part are larger than the benchmark element. The two parts are then sorted recursively until the entire sequence is sorted.
The following is a quick sort code example in Java:
public class QuickSort { public void quickSort(int[] arr, int low, int high) { if (low < high) { int pi = partition(arr, low, high); quickSort(arr, low, pi - 1); quickSort(arr, pi + 1, high); } } public int partition(int[] arr, int low, int high) { int pivot = arr[high]; int i = (low - 1); for (int j = low; j < high; j++) { if (arr[j] < pivot) { i++; int temp = arr[i]; arr[i] = arr[j]; arr[j] = temp; } } int temp = arr[i + 1]; arr[i + 1] = arr[high]; arr[high] = temp; return i + 1; } public static void main(String[] args) { int[] arr = {10, 7, 8, 9, 1, 5}; int n = arr.length; QuickSort sorter = new QuickSort(); sorter.quickSort(arr, 0, n - 1); System.out.println("排好序的数组:"); for (int i : arr) { System.out.print(i + " "); } } }
In the above example, we first define a QuickSort class and declare the quickSort and partition methods in it. The quick sort method quickSort uses a recursive method to divide the array into two parts by calling the partition method, and then continue to call the quickSort method recursively on the two parts until the entire array is sorted. The partition method is used to determine the base element and place elements smaller than the base element to the left of the base element and elements larger than the base element to the right of the base element.
In the main method, we create an array arr containing some unsorted elements and pass it to the quickSort method for sorting. Finally, we use a loop to print out the sorted array.
Through the above code example, we can call the quick sort function in Java to sort data. You can modify and adjust this example to implement more complex sorting functionality based on your actual needs. Hope this article helps you!
The above is the detailed content of Write quick sort algorithm in Java to sort data. For more information, please follow other related articles on the PHP Chinese website!
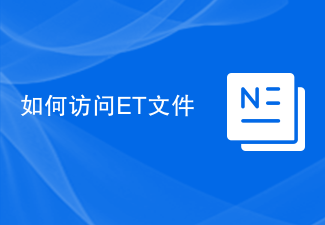
ET文件是一种非常常见的文件格式,它通常是由WPS软件中的表格编辑器生成的。在接触ET文件之前,我们可以先了解一下什么是ET文件,然后讨论如何打开和编辑它们。ET文件是WPS表格软件的文件格式,类似于MicrosoftExcel中的XLS或XLSX文件。WPS表格是一款功能强大的电子表格软件,提供了类似Excel的功能,可以进行数据处理、数据分析和图表创建
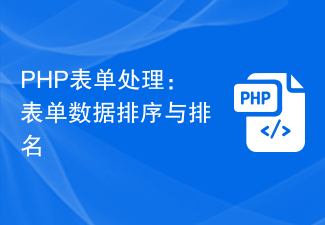
PHP表单处理:表单数据排序与排名在Web开发中,表单是一种常见的用户输入方式。当我们收集到来自用户的表单数据后,通常需要对这些数据进行处理和分析。本文将介绍如何使用PHP对表单数据进行排序与排名,以便更好地展示和分析用户提交的数据。一、表单数据排序当我们收集到用户提交的表单数据后,可能会发现这些数据的顺序不一定符合我们的要求。而对于需要按照特定规则展示或分
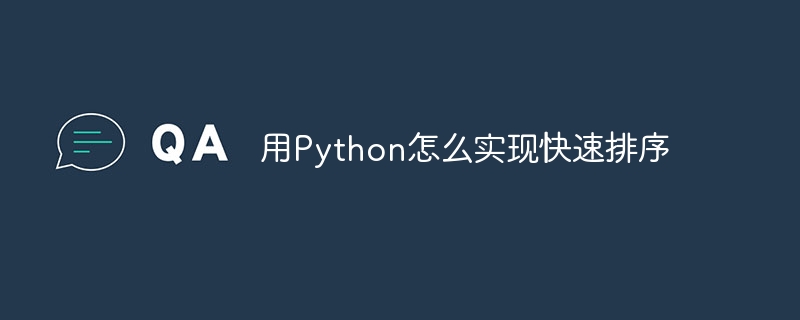
用Python实现快速排序的方法:1、定义一个名为quick_sort的函数,使用递归的方法来实现快速排序;2、检查数组的长度,如果长度小于等于1,则直接返回数组,否则,选择数组中的第一个元素作为枢纽元素(pivot),然后将数组分成比枢纽元素小和比枢纽元素大的两个子数组;3、将这两个子数组和枢纽元素连接起来,形成排序好的数组即可。
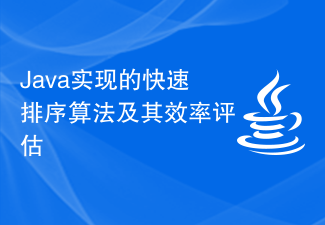
快速排序的Java实现及其性能分析快速排序(QuickSort)是一种很常用且高效的排序算法,它是一种分治法(DivideandConquer)的思想。该算法通过将一个数组分成两个子数组,然后对这两个子数组分别进行排序,最终将整个数组变为有序序列。在处理大规模数据时,快速排序表现出了非常出色的性能。快速排序的实现采用递归的方式,基本思路如下:选择一个基
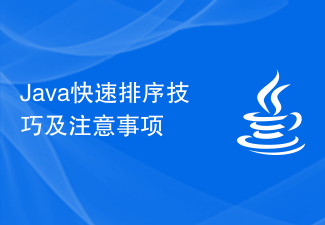
掌握Java快速排序的关键技巧和注意事项快速排序(QuickSort)是一种常用的排序算法,其核心思想是通过选择一个基准元素,将待排序序列分割成独立的两部分,其中一部分的所有元素均小于基准元素,另一部分的所有元素均大于基准元素,然后对这两部分分别进行递归排序,最终得到有序序列。虽然快速排序在平均情况下的时间复杂度为O(nlogn),但在最坏情况下会退化为O
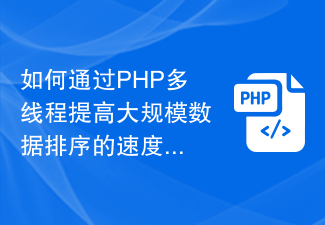
如何通过PHP多线程提高大规模数据排序的速度随着互联网的高速发展和大数据的普及,对于处理海量数据的需求也越来越大。其中,对于数据排序这一常见问题,如何提高处理速度成为了一个亟待解决的问题。在PHP领域,多线程技术被认为是一种有效的解决方案。本文将介绍如何通过PHP多线程提高大规模数据排序的速度。一、多线程的原理多线程是指同时存在多个线程,多个线程可同时执行不
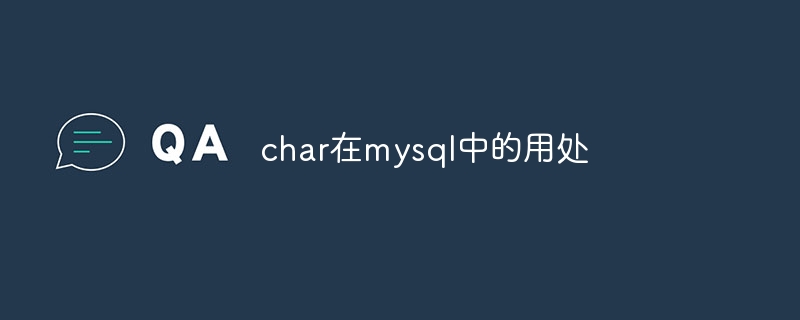
CHAR 数据类型在 MySQL 中用于存储固定长度的文本数据,可确保数据一致性、提高查询性能。该类型规定了数据长度,介于 0 到 255 个字符之间,长度在创建表时指定,并且对于同一列的所有行保持不变。对于可变长度的数据,建议使用 VARCHAR 类型。
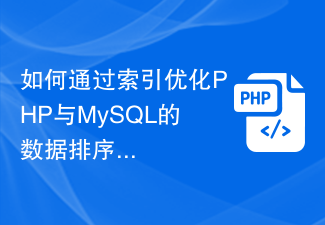
如何通过索引优化PHP与MySQL的数据排序和数据分组的效率?在开发Web应用过程中,经常需要对数据进行排序和分组操作。而对于PHP与MySQL之间的数据排序和数据分组操作,我们可以通过索引来优化其效率。索引是一种数据结构,用于提高数据的检索速度。它可以加快数据的排序、分组以及查找操作。下面我们将介绍如何通过索引来优化PHP与MySQL的数据排序和数据分组的


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
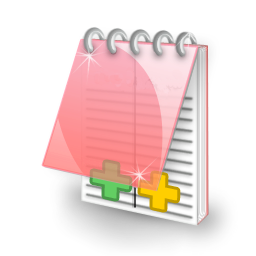
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
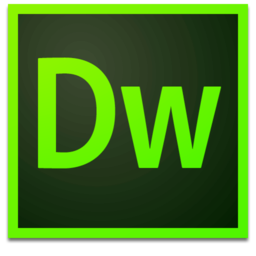
Dreamweaver Mac version
Visual web development tools
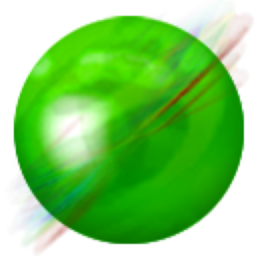
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
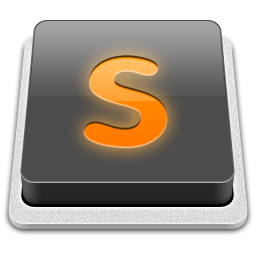
SublimeText3 Mac version
God-level code editing software (SublimeText3)

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
