


Best Practices for Python Blockchain Development: Avoid Common Mistakes to Ensure Project Success
Blockchain technology has become one of the hottest technologies in the world today, and python as a powerful Programming languages also play an important role in blockchain development. However, there are also some common errors in Python blockchain development, which may cause project to fail. To avoid these mistakes and ensure project success, this article will introduce some best practices for Python blockchain development.
1. Choose a suitable development environment
Blockchain development requires the use of a variety of tools and software, and these tools and software need to be compatible with the operating environment. Choosing a suitable development environment can improve development efficiency and avoid potential problems. Commonly used Python blockchain development environments include:
- Anaconda: Anaconda is a free, open source data science platform that provides Python and many popular scientific computing libraries. It is a popular choice for Python blockchain development .
- PyCharm: PyCharm is a professional python development environment that provides many functions, including code editing, debugging, and unit testing etc., very suitable for Python blockchain development.
- Visual Studio Code: Visual Studio Code is a free, open source code editor that provides many features, including syntax highlighting, auto-completion, debugging, etc. , is also a popular choice for Python blockchain development.
2. Reasonably design the code structure
Rational design usually includes modularizing the code according to function, with each module responsible for a function to enhance the readability and maintainability of the code. In addition, using appropriate data structures and algorithms can improve the performance and efficiency of your code.
# 导入必要的库 import hashlib import JSON # 定义一个函数来计算哈希值 def calculate_hash(data): """ 计算数据的哈希值 :param data: 要计算哈希值的数据 :return: 数据的哈希值 """ # 创建一个SHA256哈希对象 hasher = hashlib.sha256() # 将数据转换为字节数组 data_bytes = data.encode("utf-8") # 将数据字节数组更新到哈希对象中 hasher.update(data_bytes) # 获取hash值 hash_value = hasher.hexdigest() # 返回hash值 return hash_value # 定义一个函数来创建区块 def create_block(index, timestamp, data, previous_hash): """ 创建一个区块 :param index: 区块的索引 :param timestamp: 区块的时间戳 :param data: 区块的数据 :param previous_hash: 上一个区块的哈希值 :return: 一个区块 """ # 创建一个区块 block = { "index": index, "timestamp": timestamp, "data": data, "previous_hash": previous_hash } # 计算区块的哈希值 block["hash"] = calculate_hash(json.dumps(block)) # 返回区块 return block # 创建创世区块 genesis_block = create_block(0, "2023-03-08 12:00:00", "创世区块", "0") # 创建第二个区块 second_block = create_block(1, "2023-03-08 12:01:00", "第二个区块", genesis_block["hash"]) # 创建第三个区块 third_block = create_block(2, "2023-03-08 12:02:00", "第三个区块", second_block["hash"]) # 打印区块链 blockchain = [genesis_block, second_block, third_block] print(json.dumps(blockchain, indent=4))
3. Use the right libraries and tools
There are many libraries and tools in Python that can help us develop blockchain applications, such as:
- web3.py: WEB3.py is a library for interacting with the Ethereum blockchain, which provides a number of features including Send transactions, query blockchain data, etc.
- eth-abi: eth-abi is a library for encoding and decoding Ethereum smart contract function parameters and return values.
- eth-account: eth-account is a library for creating and managing Ethereum accounts.
Choosing the right libraries and tools can simplify the blockchain development process and improve development efficiency.
4. Ensure code security
Blockchain applications handle large amounts of funds and sensitive data, so it is very important to ensure that the code is safe. Here are some ways to keep your code safe:
-
Use safe data types and libraries: Python provides some safe data types and libraries that can help us write safe code. For example, we can use the
secrets
module to generate secure random numbers, use thehashlib
module to calculate hash values, etc. - Verify input data: When processing user-entered data, we need to perform verification to prevent malicious attacks. For example, we can use regular expressions to verify email addresses, use digital signatures to verify transactions, etc.
- Use safe coding practices: When writing code, we need to follow some safe coding practices, for example, avoid using hard-coded passwords, avoid using dangerous functions, etc.
5. Conduct adequate testing
Testing is an important means to ensure code quality and reliability. In blockchain development, we need to conduct the following types of testing:
- Unit testing: Unit testing is a test for a single function or class, which can help us find logical errors in the code.
- Integration testing: Integration testing is a test that combines multiple functions or classes. It can help us find integration errors in the code.
- System testing: System testing is a test of the entire blockchain application, which can help us find system errors in the code.
By conducting adequate testing, we can ensure the quality and reliability of the code and avoid problems in the production environment.
6. Regularly update and maintain the code
Blockchain technology and the market are constantly evolving, therefore, we need to regularly update and maintain the code to ensure that the code adapts to the latest technology and market needs. Here are some ways to update and maintain your code:
- Pay attention to the latest developments in blockchain technology and market: We need to pay attention to the latest developments in blockchain technology and market in order to keep abreast of new technologies and market needs.
- Update the code regularly: We need to update the code regularly to ensure that the code is consistent with the latest technology and market needs.
- Create a code maintenance plan: We need to create a code maintenance plan so that the code can be maintained regularly.
By regularly updating and maintaining the code, we can ensure the quality and reliability of the code and avoid code problems in the production environment.
The above is the detailed content of Best Practices for Python Blockchain Development: Avoid Common Mistakes to Ensure Project Success. For more information, please follow other related articles on the PHP Chinese website!
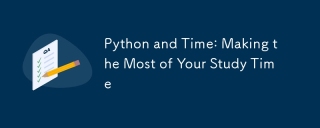
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
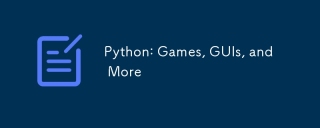
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
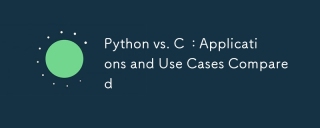
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
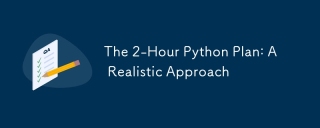
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
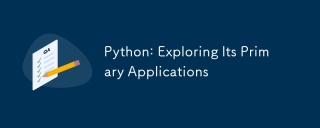
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
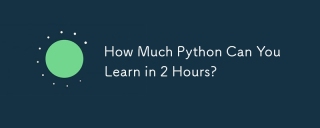
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
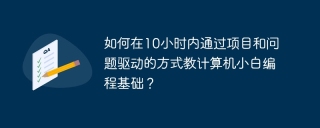
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
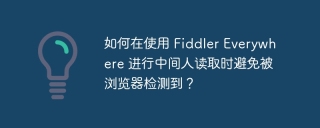
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 English version
Recommended: Win version, supports code prompts!
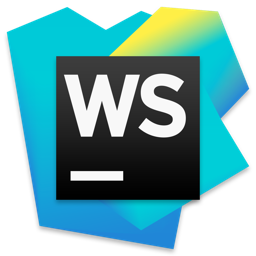
WebStorm Mac version
Useful JavaScript development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Zend Studio 13.0.1
Powerful PHP integrated development environment