


Thoroughly understand Python classes and objects and become a qualified Python developer
1. Classes and Objects
In python, a class is a blueprint for creating objects. Classes contain the data structures and behaviors of objects. Objects are instances of classes. The data structures in a class are called properties, and the behaviors in a class are called methods.
2. Define class
Use the class
keyword to define a class. The definition of a class includes the name of the class and the body of the class. The body of a class contains the properties and methods of the class.
For example, the following code defines a class named Person
:
class Person: def __init__(self, name, age): self.name = name self.age = age def greet(self): print("Hello, my name is", self.name)
In this class, the __init__
method is a special function that is called when creating an instance of the class. The __init__
method accepts two parameters: self
(representing an instance of the class) and name
and age
(representing the properties of the object).
greet
The method is a normal method that can be called by instances of the class. The greet
method accepts one parameter: self
(representing an instance of the class).
3. Create object
You can use the class
keyword to create an instance of a class. An instance of a class is a concrete object of the class.
For example, the following code creates two instances of the Person
class:
person1 = Person("John", 25) person2 = Person("Mary", 30)
person1
and person2
are two instances of the Person
class.
4. Access properties and methods
You can use the .
operator to access the properties and methods of a class.
For example, the following code accesses the name
attribute of the person1
object:
print(person1.name)
Output:
John
The following code calls the greet
method of the person1
object:
person1.greet()
Output:
Hello, my name is John
5. Class inheritance
Python Supports class inheritance. Class inheritance allows one class to inherit the properties and methods of another class.
For example, the following code defines a class named Student
, which inherits the Person
class:
class Student(Person): def __init__(self, name, age, major): super().__init__(name, age) self.major = major def study(self): print("I am studying", self.major)
In this class, the __init__
method calls super().__init__
to inherit the attributes and methods of the Person
class. study
Method is a normal method that can be called by instances of a class. The study
method accepts one parameter: self
(representing an instance of the class).
6. Class polymorphism
Python supports class polymorphism. Class polymorphism allows the same method to have different behaviors for different classes.
For example, the following code defines a function named greet_person
that accepts an instance of the Person
class as a parameter:
def greet_person(person): person.greet()
This function can be called on instances of the Person
class and its derived classes.
For example, the following code calls the greet_person
function to greet person1
and person2
:
greet_person(person1) greet_person(person2)
Output:
Hello, my name is John Hello, my name is Mary
The above is the detailed content of Thoroughly understand Python classes and objects and become a qualified Python developer. For more information, please follow other related articles on the PHP Chinese website!
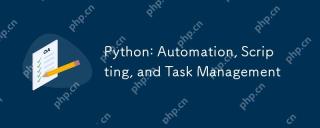
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
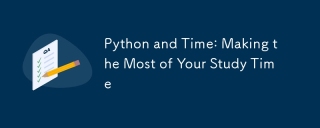
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
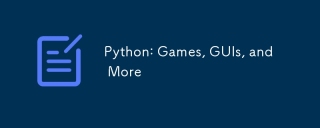
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
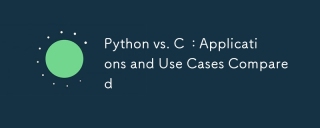
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
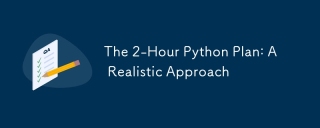
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
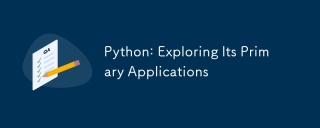
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
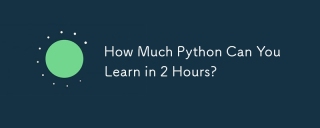
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
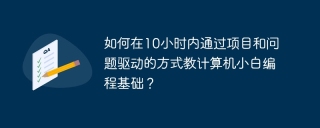
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
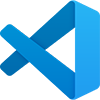
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.