Must-know jQuery event knowledge
Must master jQuery event knowledge, need specific code examples
In front-end development, jQuery is a widely used JavaScript library, which simplifies DOM operations, Event processing, animation effects and other tasks. Among them, event processing is a crucial part of web page interaction. Mastering the knowledge of jQuery events can help developers better achieve various interactive effects and user experience. This article will introduce some necessary knowledge of jQuery events and provide specific code examples.
- Click event
Click event is one of the most common events, which can trigger corresponding actions when the user clicks on a page element. The following is a simple click event example:
<!DOCTYPE html> <html> <head> <title>点击事件示例</title> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> </head> <body> <button id="myButton">点击我</button> <script> $(document).ready(function(){ $("#myButton").click(function(){ alert("你点击了按钮"); }); }); </script> </body> </html>
In the above code, when the user clicks the button, a prompt box will pop up showing "You clicked the button".
- Hover event
Hover event is an event triggered when the user hovers the mouse over a page element. It is usually used to implement some special effects. The following is an example of a hover event:
<!DOCTYPE html> <html> <head> <title>悬停事件示例</title> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> </head> <body> <div id="myDiv" style="width: 100px; height: 100px; background-color: red;"></div> <script> $(document).ready(function(){ $("#myDiv").hover(function(){ $(this).css("background-color", "blue"); }, function(){ $(this).css("background-color", "red"); }); }); </script> </body> </html>
In the above code, when the user hovers the mouse over the red square, the background color of the square will change to blue; when the mouse moves out, the color will change. Return to red.
- Double-click event
Double-click event is an event triggered when the user clicks on a page element twice in succession. The following is an example of a double-click event:
<!DOCTYPE html> <html> <head> <title>双击事件示例</title> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> </head> <body> <h2 id="双击这里">双击这里</h2> <script> $(document).ready(function(){ $("#myHeader").dblclick(function(){ $(this).css("color", "green"); }); }); </script> </body> </html>
In the above code, when the user double-clicks the title, the text color of the title will change to green.
Through the above examples, we can see how to use jQuery to handle various events. Of course, jQuery also provides more event handling methods, such as keyboard events, form events, scroll events, etc. Mastering the knowledge of these events allows developers to handle user interactions more flexibly and improve the interactive experience of web pages.
In short, proficiency in jQuery event knowledge is a basic requirement in front-end development. Through continuous practice and accumulation of experience, you can write more colorful interactive effects and provide users with a better browsing experience. I hope the above content can help readers better understand and use jQuery event processing.
The above is the detailed content of Must-know jQuery event knowledge. For more information, please follow other related articles on the PHP Chinese website!
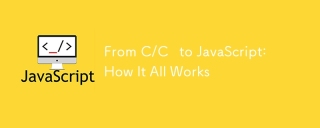
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
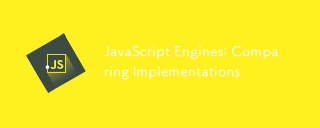
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
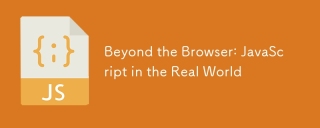
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
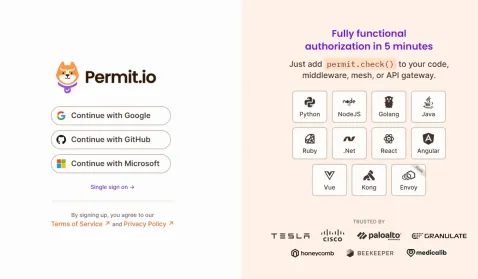
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
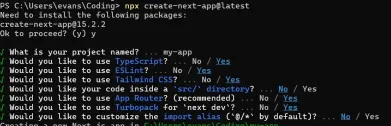
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
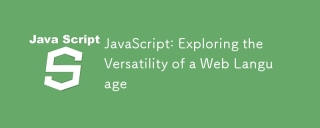
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
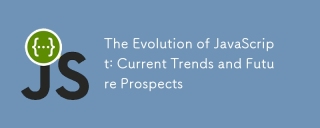
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
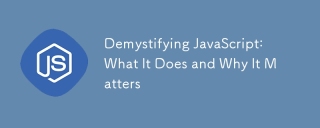
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
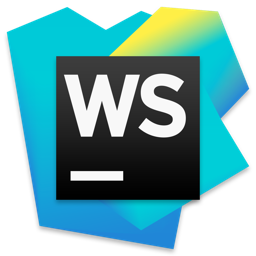
WebStorm Mac version
Useful JavaScript development tools

Notepad++7.3.1
Easy-to-use and free code editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.