As an efficient and concise programming language, Golang has excellent performance in file processing. Among them, file monitoring is a very common and useful function, which can help us monitor changes in the file system in real time, so as to make corresponding processing in a timely manner. This article will delve into how Golang implements the file monitoring function and provide specific code examples to help readers better understand and apply this function.
Why do you need the file monitoring function?
In the modern software development process, file operation is a very important link. Especially in some scenarios where file status needs to be updated in real time, such as log monitoring, file synchronization, etc., the file monitoring function is particularly important. Through file monitoring, we can obtain the changes in files in real time, so as to make corresponding processing in a timely manner and improve the real-time and stability of the system.
How does Golang implement file monitoring function?
In Golang, the file monitoring function can be implemented by using the fsnotify
package. fsnotify
is a cross-platform file monitoring library that can monitor file creation, modification, deletion and other events in the file system. Next, we will use a simple example to demonstrate how to use fsnotify
to implement the file monitoring function in Golang.
First, we need to install the fsnotify
package:
go get github.com/fsnotify/fsnotify
Next, we can write a simple file monitoring program:
package main import ( "fmt" "github.com/fsnotify/fsnotify" ) func main() { watcher, err := fsnotify.NewWatcher() if err != nil { fmt.Println("Error:", err) return } defer watcher.Close() err = watcher.Add("./testFolder/") // 监控目标文件夹 if err != nil { fmt.Println("Error:", err) return } fmt.Println("Watching for file changes...") for { select { case event, ok := <-watcher.Events: if !ok { return } fmt.Println("Event:", event) if event.Op&fsnotify.Write == fsnotify.Write { fmt.Println("File modified:", event.Name) } case err, ok := <-watcher.Errors: if !ok { return } fmt.Println("Error:", err) } } }
In this example , we first created a Watcher
object to monitor file changes in the specified directory. Then it continuously monitors file change events through a for
loop, and handles them accordingly according to the type of event. Here, we only processed the file write event. When a file is modified, the program will output the corresponding information.
Summary
Through the above example, we learned how to use the fsnotify
package to implement the file monitoring function in Golang. The file monitoring function has a wide range of application scenarios in actual development. By monitoring file changes, you can respond to system changes in a timely manner and improve the real-time and stability of the system. Readers can further expand this example to implement more complex file monitoring functions based on actual needs. I hope this article can be helpful to readers, thank you for reading!
The above is the detailed content of In-depth exploration: How Golang implements file monitoring function. For more information, please follow other related articles on the PHP Chinese website!
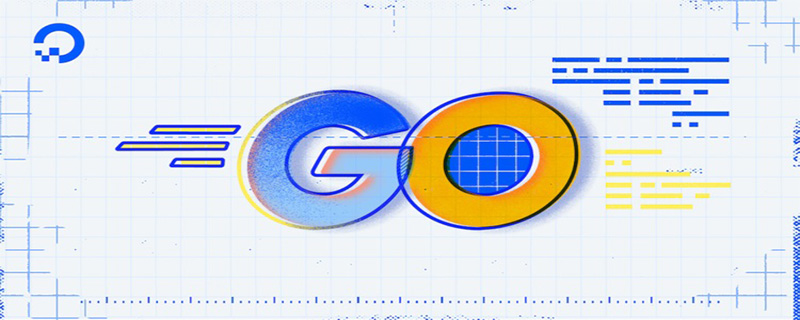
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
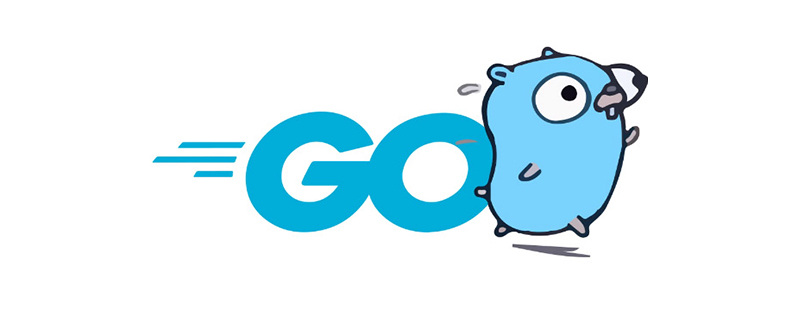
本篇文章带大家了解一下golang 的几种常用的基本数据类型,如整型,浮点型,字符,字符串,布尔型等,并介绍了一些常用的类型转换操作。
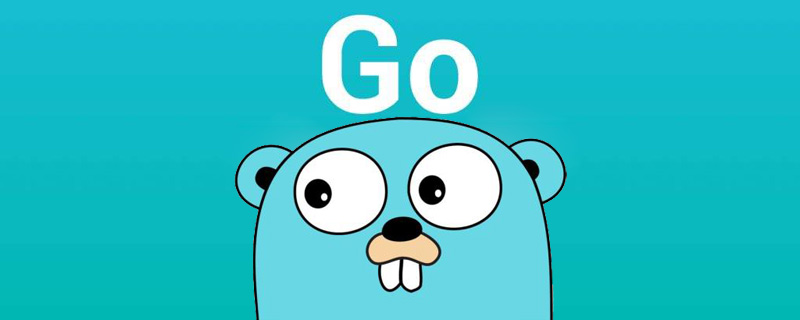
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
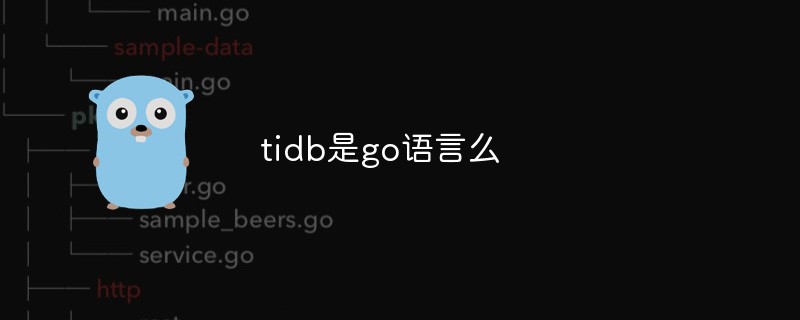
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
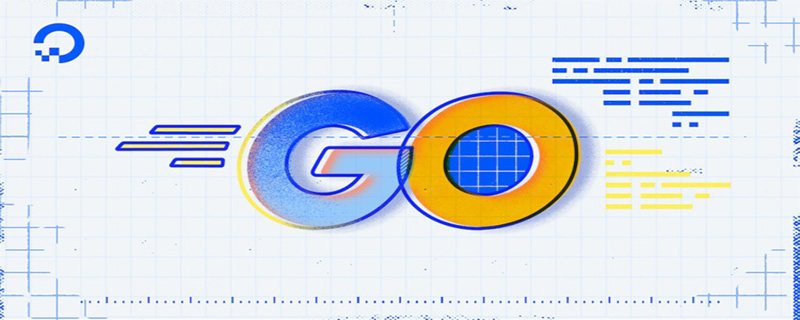
在写 Go 的过程中经常对比这两种语言的特性,踩了不少坑,也发现了不少有意思的地方,下面本篇就来聊聊 Go 自带的 HttpClient 的超时机制,希望对大家有所帮助。
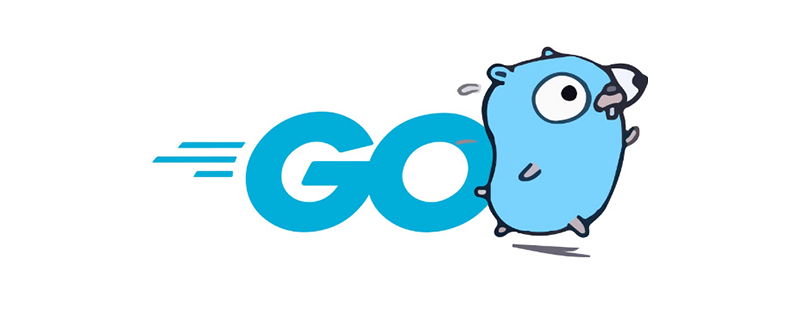
go语言需要编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言,也就说Go语言程序在运行之前需要通过编译器生成二进制机器码(二进制的可执行文件),随后二进制文件才能在目标机器上运行。
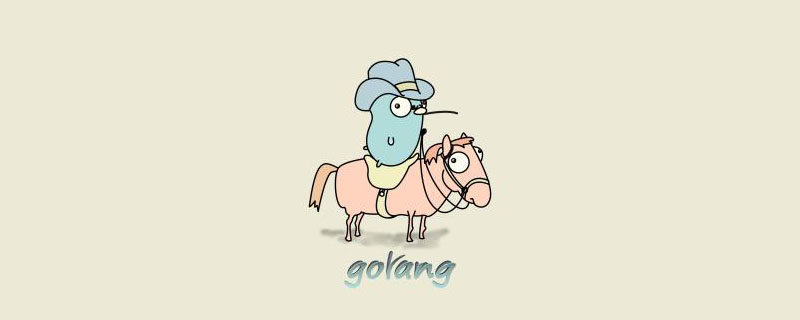
删除map元素的两种方法:1、使用delete()函数从map中删除指定键值对,语法“delete(map, 键名)”;2、重新创建一个新的map对象,可以清空map中的所有元素,语法“var mapname map[keytype]valuetype”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor

Atom editor mac version download
The most popular open source editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
