


In-depth analysis of JVM principles: exploring the parsing and execution of Java bytecode
In-depth analysis of JVM principles: Exploring the parsing and execution of Java bytecode
Introduction:
JVM (Java Virtual Machine) is the core engine for Java program execution , responsible for parsing and executing Java bytecode. A deep understanding of JVM principles is crucial for developers. It not only helps us optimize code performance, but also helps us solve some common problems. This article will provide an in-depth analysis of how the JVM works and illustrate it with specific code examples.
1. Composition of JVM
JVM consists of three core components: ClassLoader, Runtime Data Area and Execution Engine.
1.1 Class loader
The class loader is responsible for loading compiled Java bytecode into the JVM. JVM provides three built-in class loaders: Bootstrap ClassLoader, Extension ClassLoader and Application ClassLoader. Different class loaders are responsible for loading different classes.
1.2 Runtime data area
The runtime data area includes method area (Method Area), heap (Heap), virtual machine stack (VM Stack), native method stack (Native Method Stack) and program counter (Program Counter) etc. Each thread has its own virtual machine stack and program counter, while the method area and heap are shared by all threads.
1.3 Execution engine
The execution engine is responsible for interpreting and executing Java bytecode. JVM supports two execution engines: interpreter (Interpreter) and just-in-time compiler (Just-In-Time Compiler, JIT). The interpreter interprets and executes the bytecode one by one, while the just-in-time compiler converts the bytecode into native machine code and executes it.
2. Parsing and Execution of Bytecode
Java bytecode is a platform-independent intermediate code. Java programs will be compiled into bytecode during the compilation process. Bytecode consists of a sequence of instructions that the JVM parses and executes to execute a Java program.
2.1 Bytecode parsing
Bytecode parsing is the first stage of JVM. It is responsible for reading bytecode files into memory and parsing them into a format that the JVM can understand. The specific process is as follows:
import java.io.FileInputStream; import java.io.IOException; public class BytecodeParser { public static void main(String[] args) { try { FileInputStream fis = new FileInputStream("HelloWorld.class"); byte[] bytecode = new byte[fis.available()]; fis.read(bytecode); fis.close(); // 解析字节码逻辑 // ... } catch (IOException e) { e.printStackTrace(); } } }
In the above code example, we read the bytecode file into memory through FileInputStream and save it in the bytecode array. Next, we can parse the bytecode instructions one by one by parsing the bytecode array and perform the corresponding operations.
2.2 Execution of bytecode
Execution of bytecode is the second stage of the JVM, which is responsible for converting the parsed bytecode instructions into machine code and executing it. The execution engine of the JVM uses different strategies to execute bytecode according to different situations. It can be interpreted or compiled. The following is a simple example:
public class HelloWorld { public static void main(String[] args) { System.out.println("Hello, World!"); } }
In the above code, the JVM parses the bytecode instructions of the line System.out.println("Hello, World!")
into the corresponding machine code and execute the machine code to print out "Hello, World!".
3. Application of JVM principles
In-depth understanding of JVM principles is crucial for developers. It can help us optimize code performance, solve memory leak problems, etc. The following are several common application scenarios:
3.1 Performance Optimization
By in-depth understanding of JVM principles, we can know which codes will cause performance problems and improve the performance of the program by optimizing the code. For example, we can avoid frequent creation and destruction of objects and reduce the burden of GC by reusing objects.
3.2 Memory Optimization
An in-depth understanding of JVM principles can help us discover problems such as memory leaks and memory overflows, and take appropriate measures for tuning. For example, we can solve the memory leak problem by analyzing GC logs to find unreasonable object references.
3.3 Exception Handling
Various exceptions may occur during the running of the JVM. An in-depth understanding of JVM principles can help us better capture and handle these exceptions. For example, by analyzing the exception stack information, we can find the source of the exception and handle it accordingly.
Conclusion:
JVM is the core engine for Java program execution. An in-depth understanding of JVM principles is crucial for developers. This article explains the parsing and execution process of Java bytecode through an in-depth analysis of JVM principles and specific code examples. An in-depth understanding of JVM principles can help us optimize code performance, solve common problems, and improve the quality and performance of Java programs.
The above is the detailed content of In-depth analysis of JVM principles: exploring the parsing and execution of Java bytecode. For more information, please follow other related articles on the PHP Chinese website!
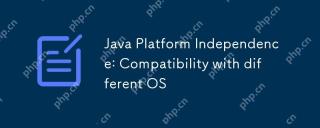
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunondifferentoperatingsystemswithoutmodification.TheJVMcompilesJavacodeintoplatform-independentbytecode,whichittheninterpretsandexecutesonthespecificOS,abstractingawayOS
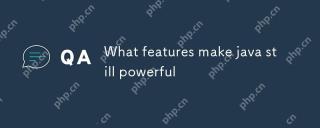
Javaispowerfulduetoitsplatformindependence,object-orientednature,richstandardlibrary,performancecapabilities,andstrongsecurityfeatures.1)PlatformindependenceallowsapplicationstorunonanydevicesupportingJava.2)Object-orientedprogrammingpromotesmodulara
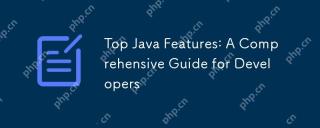
The top Java functions include: 1) object-oriented programming, supporting polymorphism, improving code flexibility and maintainability; 2) exception handling mechanism, improving code robustness through try-catch-finally blocks; 3) garbage collection, simplifying memory management; 4) generics, enhancing type safety; 5) ambda expressions and functional programming to make the code more concise and expressive; 6) rich standard libraries, providing optimized data structures and algorithms.
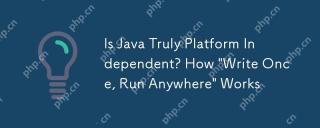
JavaisnotentirelyplatformindependentduetoJVMvariationsandnativecodeintegration,butitlargelyupholdsitsWORApromise.1)JavacompilestobytecoderunbytheJVM,allowingcross-platformexecution.2)However,eachplatformrequiresaspecificJVM,anddifferencesinJVMimpleme
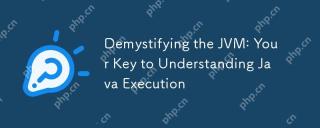
TheJavaVirtualMachine(JVM)isanabstractcomputingmachinecrucialforJavaexecutionasitrunsJavabytecode,enablingthe"writeonce,runanywhere"capability.TheJVM'skeycomponentsinclude:1)ClassLoader,whichloads,links,andinitializesclasses;2)RuntimeDataAr
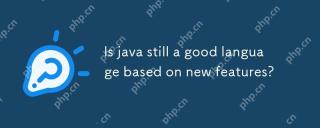
Javaremainsagoodlanguageduetoitscontinuousevolutionandrobustecosystem.1)Lambdaexpressionsenhancecodereadabilityandenablefunctionalprogramming.2)Streamsallowforefficientdataprocessing,particularlywithlargedatasets.3)ThemodularsystemintroducedinJava9im
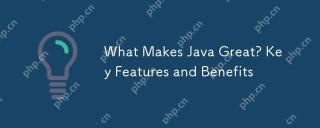
Javaisgreatduetoitsplatformindependence,robustOOPsupport,extensivelibraries,andstrongcommunity.1)PlatformindependenceviaJVMallowscodetorunonvariousplatforms.2)OOPfeatureslikeencapsulation,inheritance,andpolymorphismenablemodularandscalablecode.3)Rich
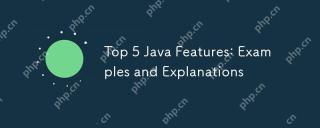
The five major features of Java are polymorphism, Lambda expressions, StreamsAPI, generics and exception handling. 1. Polymorphism allows objects of different classes to be used as objects of common base classes. 2. Lambda expressions make the code more concise, especially suitable for handling collections and streams. 3.StreamsAPI efficiently processes large data sets and supports declarative operations. 4. Generics provide type safety and reusability, and type errors are caught during compilation. 5. Exception handling helps handle errors elegantly and write reliable software.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 English version
Recommended: Win version, supports code prompts!
