php editor Baicao has carefully written a Java Q&A article for you about using AAD and AWS Cognito to protect Spring Boot REST API. In this article, we will explore how to leverage these two authentication services to protect different endpoints and ensure that your API is safe and secure. Follow our guide and learn how to implement authentication and authorization in your Spring Boot project to make your REST API more powerful and reliable.
Question content
Hopefully someone can help me here as I can't find any resources on this topic anywhere.
I have a spring boot restapi, and the current configuration has two routes: 1. Unauthorized 2. Authorized through the bearer of aad/entra
My configuration method is currently set up as follows:
@override protected void configure(httpsecurity http) throws exception { super.configure(http); http.csrf().disable(); http.authorizerequests(requests -> requests .antmatchers(httpmethod.options, "/**/**").permitall() .antmatchers("/api/protected/**").fullyauthenticated() .anyrequest().permitall() ); }
It is wrapped in a class that extends aadresourceserverwebsecurityconfigureradapter
.
By configuring our api in this way, we are able to secure our routes as follows:
@preauthorize("hasauthority('approle_appname.rolename')") @getmapping(value = "/some-method", produces = mediatype.application_json_value) public responseentity<list<string>> getstrings() { return responseentity.ok(...); }
Our api should now be extended to allow new types of users to use the authorization endpoint. These users are managed by aws cognito. How do I set up my websecurityconfigureradapter
to allow some paths to be unauthorized, some paths to be protected via aad, and some paths to be protected via aws cognito?
The main problem I seem to be having is aadresourceserverwebsecurityconfigureradapter
configuring jwt validation in such a way that it only works with bearers provided by microsoft.
Ideally I would like something like this:
@configuration @enablewebsecurity @enableglobalmethodsecurity(prepostenabled = true) public class securityconfig extends websecurityconfigureradapter { @configuration @order(1) public static class azureadsecurityconfig extends aadresourceserverwebsecurityconfigureradapter { @override protected void configure(httpsecurity http) throws exception { http.authorizerequests(requests -> requests .antmatchers("/api/aad/**").fullyauthenticated() ); http.oauth2resourceserver().jwt([utilize aad jwt validation]); } } @configuration @order(2) public static class awscognitosecurityconfig extends websecurityconfigureradapter { @override protected void configure(httpsecurity http) throws exception { http.authorizerequests(requests -> requests .antmatchers("/api/cognito/**").fullyauthenticated() ); http.oauth2resourceserver().jwt([utilize aws cognito jwt validation]); } } @configuration @order(3) public static class defaultsecurityconfig extends websecurityconfigureradapter { @override protected void configure(httpsecurity http) throws exception { http.csrf().disable(); http.authorizerequests(requests -> requests .antmatchers(httpmethod.options, "/**/**").permitall() .anyrequest().permitall() ); } } }
Another issue I found is that aadresourceserverwebsecurityconfigureradapter
automatically sets all possible prefixes for the jwtclaimnames "roles" and "scp" to "scope_" and "approle_". Ideally I would like them to be different for aad and aws cognito so that I prefix "aad_scope_", "aad_approle_" and "cognito_group_".
I found some information explaining how to implement multi-tenant jwt authentication for spring boot, but they all only use sql database to implement password/user based authentication.
Is there a way to basically have to re-implement all the aad logic so that I can mix in the validation of the jwt given by aws cognito, or is there a way to make the decision based on routing?
I already know that you can use the oauth2resourceserver()
function on httpsecurity
to configure jwt usage, but I only found information on how to implement that functionality for a single tenant.
If anyone has successfully implemented this specific or similar case, or can push me in the right direction, I would be very grateful. Or maybe my idea is completely wrong, so please tell me.
Using Working Solutions Update (January 25, 2024)
Thanks to @ch4mp for the answer, I have succeeded. >Working Answers
My implementation is now highly simplified and looks like this:
application.yml
com: c4-soft: springaddons: oidc: ops: - iss: https://cognito-idp.<region>.amazonaws.com/<cognito-pool> authorities: - path: $.cognito:groups prefix: cognito_group_ - iss: https://sts.windows.net/<entra objectid>/ authorities: - path: $.roles.* prefix: aad_approle_ - path: $.scp prefix: aad_scope_ aud: <enterprise application id> resource-server: permit-all: - /api/route/noauth
Security Configuration
package some.package; import org.springframework.context.annotation.configuration; import org.springframework.security.config.annotation.method.configuration.enablemethodsecurity; import org.springframework.security.config.annotation.web.configuration.enablewebsecurity; @enablewebsecurity @enablemethodsecurity @configuration public class securityconfig { }
My controller now looks like this:
package some.package; import org.springframework.http.responseentity; import org.springframework.security.access.prepost.preauthorize; import org.springframework.security.core.context.securitycontextholder; import org.springframework.security.oauth2.jwt.jwt; import org.springframework.web.bind.annotation.getmapping; import org.springframework.web.bind.annotation.requestmapping; import org.springframework.web.bind.annotation.restcontroller; @restcontroller @requestmapping("/api/route") public class jwttestcontroller { @getmapping("/aadauth") @preauthorize("hasauthority('aad_approle_grantedapprole.xxx')") public responseentity<string> aadauthrole() { jwt jwt = (jwt) securitycontextholder.getcontext().getauthentication().getprincipal(); return responseentity.ok(jwt.getclaims().tostring()); } @getmapping("/aadauth") @preauthorize("hasauthority('aad_scope_grantedscope.xxx')") public responseentity<string> aadauthscope() { jwt jwt = (jwt) securitycontextholder.getcontext().getauthentication().getprincipal(); return responseentity.ok(jwt.getclaims().tostring()); } @preauthorize("hasauthority('cognito_group_somegroup')") @getmapping("/cognitoauth") public responseentity<string> cognitoauth() { jwt jwt = (jwt) securitycontextholder.getcontext().getauthentication().getprincipal(); return responseentity.ok(jwt.getclaims().tostring()); } @getmapping("/noauth") public responseentity<string> noauth() { return responseentity.ok("hello world!"); } }
Build.gradle
implementation 'org.springframework.boot:spring-boot-starter-oauth2-resource-server' implementation 'com.c4-soft.springaddons:spring-addons-starter-oidc:7.3.5'
This is not the official launcher of spring, but the oss implementation: https://www.php.cn/link/49844ba129a1cbc3d964703fcdb756ba
I'll update again if I run into any other issues, but for now it's working.
Solution
I'm going to expose a solution here using my starter since it's easier.
If you prefer to build a secure configuration using only the "official" spring boot launcher, you must provide your own authenticationmanagerresolver<httpservletrequest></httpservletrequest>
using the iss
declaration, per authentication manager Each server has its own authentication converter and its own permissions converter to handle the origin claims and prefixes you want. Browse my tutorials or official documentation for examples and implementation tips. This other answer may also help (the permission mapping requirements are completely different, but the Authentication Manager resolver is similar).
Use boot 3.2.2
and spring-addons
<?xml version="1.0" encoding="utf-8"?> <project xmlns="http://maven.apache.org/pom/4.0.0" xmlns:xsi="http://www.w3.org/2001/xmlschema-instance" xsi:schemalocation="http://maven.apache.org/pom/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelversion>4.0.0</modelversion> <parent> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-parent</artifactid> <version>3.2.2</version> <relativepath/> <!-- lookup parent from repository --> </parent> <groupid>com.c4-soft.demo</groupid> <artifactid>multi-tenant-resource-server</artifactid> <version>0.0.1-snapshot</version> <properties> <java.version>21</java.version> <spring-addons.version>7.3.5</spring-addons.version> </properties> <dependencies> <dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-oauth2-resource-server</artifactid> </dependency> <dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-web</artifactid> </dependency> <dependency> <groupid>com.c4-soft.springaddons</groupid> <artifactid>spring-addons-starter-oidc</artifactid> <version>${spring-addons.version}</version> </dependency> <dependency> <groupid>com.c4-soft.springaddons</groupid> <artifactid>spring-addons-starter-oidc-test</artifactid> <version>${spring-addons.version}</version> <scope>test</scope> </dependency> </dependencies> <build> <plugins> <plugin> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-maven-plugin</artifactid> </plugin> </plugins> </build> </project>
@configuration @enablemethodsecurity public class securityconf { }
Edit the following application.yaml
to place your own publisher:
com: c4-soft: springaddons: oidc: ops: - iss: https://cognito-idp.us-west-2.amazonaws.com/us-west-2_rzhmglwjl authorities: - path: $.cognito:groups prefix: cognito_group_ - iss: https://sts.windows.net/0a962d63-6b23-4416-81a6-29f88c553998/ authorities: - path: $.approles.*.displayname prefix: aad_approle_ - path: $.scope prefix: aad_scope_ resourceserver: # spring-addons whitelist is for permitall() (rather than isauthenticated()) # which is probably much safer permit-all: - /actuator/health/readiness - /actuator/health/liveness - /v3/api-docs/** - /api/public/**
The value of path
above is the json path. You can use tools such as jsonpath.com to test path expressions against your own token payload (extracted using tools such as jwt.io).
Yes, it's that simple. No, I didn't omit any yaml properties or java configuration (if you don't believe me, just test it in a new project).
示例控制器
@restcontroller public class greetcontroller { @getmapping("/greet") @preauthorize("isauthenticated()") public string getgreet(authentication auth) { return "hello %s! you are granted with %s.".formatted(auth.getname(), auth.getauthorities()); } @getmapping(value = "/strings") @preauthorize("hasanyauthority('aad_approle_admin', 'cognito_group_admin')") public list<string> getstrings() { return list.of("protected", "strings"); } }
示例测试
@webmvctest(controllers = greetcontroller.class) @autoconfigureaddonswebmvcresourceserversecurity @import(securityconf.class) class greetcontrollertest { @autowired mockmvcsupport api; @test @withanonymoususer void givenuserisanonymous_whengetgreet_thenunauthorized() throws unsupportedencodingexception, exception { api.get("/greet").andexpect(status().isunauthorized()); } @test @withjwt("aad_admin.json") void givenuserisaadadmin_whengetgreet_thenok() throws unsupportedencodingexception, exception { final var actual = api.get("/greet").andexpect(status().isok()).andreturn().getresponse().getcontentasstring(); assertequals( "hello aad-admin! you are granted with [aad_approle_msiam_access, aad_approle_admin, aad_scope_openid, aad_scope_profile, aad_scope_machin:truc].", actual); } @test @withjwt("cognito_admin.json") void givenuseriscognitoadmin_whengetgreet_thenok() throws unsupportedencodingexception, exception { final var actual = api.get("/greet").andexpect(status().isok()).andreturn().getresponse().getcontentasstring(); assertequals("hello amazon-cognito-admin! you are granted with [cognito_group_admin, cognito_group_machin:truc].", actual); } @test @withjwt("aad_machin-truc.json") void givenuserisaadmachintruc_whengetgreet_thenok() throws unsupportedencodingexception, exception { final var actual = api.get("/greet").andexpect(status().isok()).andreturn().getresponse().getcontentasstring(); assertequals("hello aad-user! you are granted with [aad_approle_msiam_access, aad_scope_openid, aad_scope_profile, aad_scope_machin:truc].", actual); } @test @withjwt("cognito_machin-truc.json") void givenuseriscognitomachintruc_whengetgreet_thenok() throws unsupportedencodingexception, exception { final var actual = api.get("/greet").andexpect(status().isok()).andreturn().getresponse().getcontentasstring(); assertequals("hello amazon-cognito-user! you are granted with [cognito_group_machin:truc].", actual); } @test @withanonymoususer void givenuserisanonymous_whengetstrings_thenunauthorized() throws unsupportedencodingexception, exception { api.get("/strings").andexpect(status().isunauthorized()); } @test @withjwt("aad_admin.json") void givenuserisaadadmin_whengetstrings_thenok() throws unsupportedencodingexception, exception { final var actual = api.get("/strings").andexpect(status().isok()).andreturn().getresponse().getcontentasstring(); assertequals("[\"protected\",\"strings\"]", actual); } @test @withjwt("cognito_admin.json") void givenuseriscognitoadmin_whengetstrings_thenok() throws unsupportedencodingexception, exception { final var actual = api.get("/strings").andexpect(status().isok()).andreturn().getresponse().getcontentasstring(); assertequals("[\"protected\",\"strings\"]", actual); } @test @withjwt("aad_machin-truc.json") void givenuserisaadmachintruc_whengetstrings_thenforbidden() throws unsupportedencodingexception, exception { api.get("/strings").andexpect(status().isforbidden()); } @test @withjwt("cognito_machin-truc.json") void givenuseriscognitomachintruc_whengetstrings_thenforbidden() throws unsupportedencodingexception, exception { api.get("/strings").andexpect(status().isforbidden()); } }
使用此测试资源:
aad_admin.json
{ "sub": "aad-admin", "iss": "https://sts.windows.net/0a962d63-6b23-4416-81a6-29f88c553998/", "approles": [ { "allowedmembertypes": [ "user" ], "description": "msiam_access", "displayname": "msiam_access", "id": "ef7437e6-4f94-4a0a-a110-a439eb2aa8f7", "isenabled": true, "origin": "application", "value": null }, { "allowedmembertypes": [ "user" ], "description": "administrators only", "displayname": "admin", "id": "4f8f8640-f081-492d-97a0-caf24e9bc134", "isenabled": true, "origin": "serviceprincipal", "value": "administrator" } ], "scope": "openid profile machin:truc" }
aad_machin-truc.json
{ "sub": "aad-user", "iss": "https://sts.windows.net/0a962d63-6b23-4416-81a6-29f88c553998/", "approles": [ { "allowedmembertypes": [ "user" ], "description": "msiam_access", "displayname": "msiam_access", "id": "ef7437e6-4f94-4a0a-a110-a439eb2aa8f7", "isenabled": true, "origin": "application", "value": null } ], "scope": "openid profile machin:truc" }
cognito_admin.json
{ "sub": "amazon-cognito-admin", "iss": "https://cognito-idp.us-west-2.amazonaws.com/us-west-2_rzhmglwjl", "cognito:groups": ["admin", "machin:truc"], "scope": "openid profile cog:scope" }
cognito_machin-truc.json
{ "sub": "amazon-cognito-user", "iss": "https://cognito-idp.us-west-2.amazonaws.com/us-west-2_RzhmgLwjl", "cognito:groups": ["machin:truc"], "scope": "openid profile cog:scope" }
The above is the detailed content of Securing Spring Boot REST API for different endpoints using AAD and AWS Cognito. For more information, please follow other related articles on the PHP Chinese website!
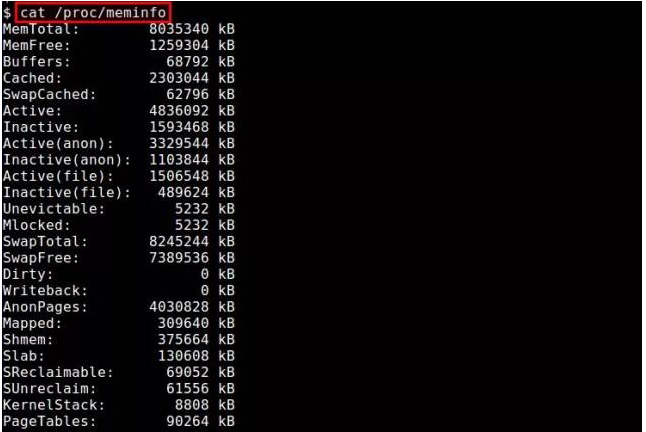
Q:我有一个问题,我想要监视Linux系统的内存使用情况。在Linux下有哪些可用的视图或命令行工具可以使用呢?A:在Linux系统中,有多种方法可以监视内存使用情况。下面是一些通过视图工具或命令行来查看内存使用情况的方法。/proc/meminfo:最简单的方法是查看/proc/meminfo文件。这个虚拟文件会动态更新,并提供了关于内存使用情况的详细信息。它列出了各种内存指标,可以满足你对内存使用情况的大部分需求。另外,你还可以通过/proc//statm和/proc//status来查看进
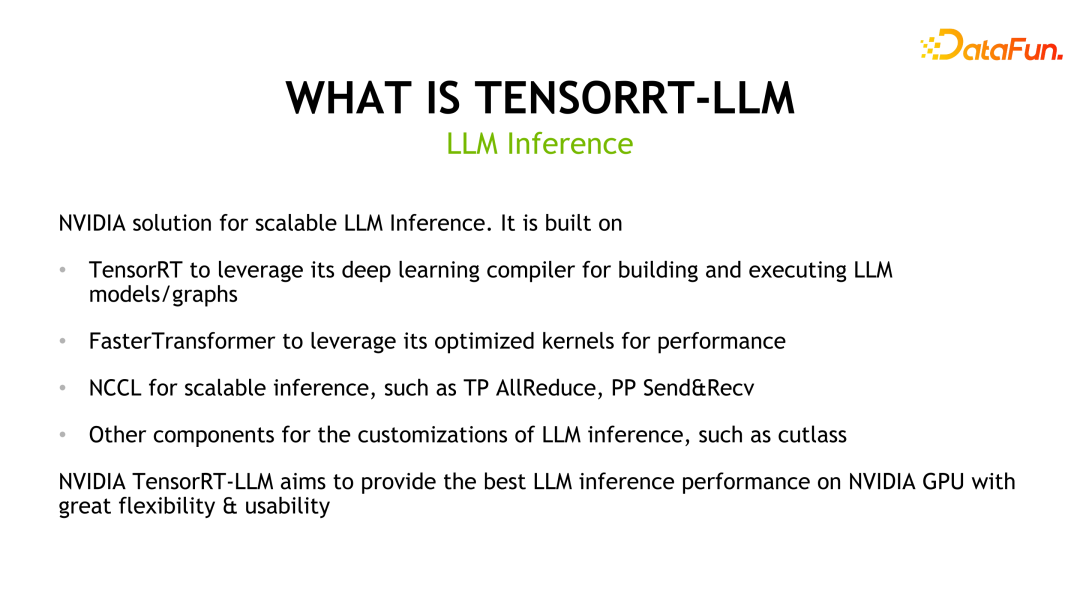
一、TensorRT-LLM的产品定位TensorRT-LLM是NVIDIA为大型语言模型(LLM)开发的可扩展推理方案。它基于TensorRT深度学习编译框架构建、编译和执行计算图,并借鉴了FastTransformer中高效的Kernels实现。此外,它还利用NCCL实现设备间的通信。开发者可以根据技术发展和需求差异,定制算子以满足特定需求,例如基于cutlass开发定制的GEMM。TensorRT-LLM是NVIDIA官方推理方案,致力于提供高性能并不断完善其实用性。TensorRT-LL
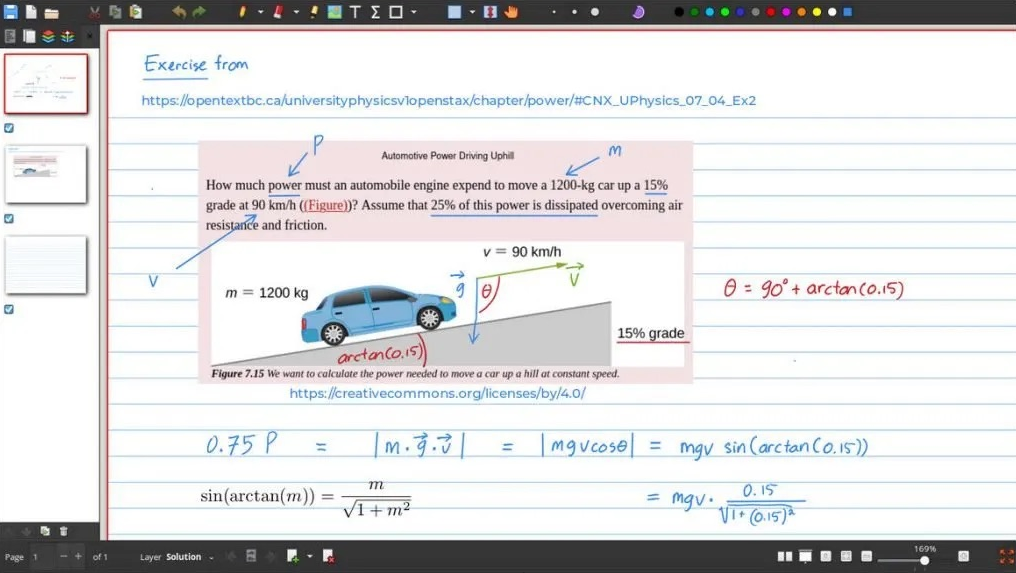
“我们将介绍几款适用于Linux系统的白板应用程序,相信这些信息对您会非常有帮助。请继续阅读!”一般来说,数字白板是一种用于大型互动显示面板的工具,常见的设备类型包括平板电脑、大屏手机、触控笔记本和表面显示设备等。当教师使用白板时,您可以使用触控笔、手写笔、手指甚至鼠标在设备屏幕上进行绘画、书写或操作元素。这意味着您可以在白板上拖动、点击、删除和绘画,就像在纸上使用笔一样。然而,要实现这一切,需要有一款软件来支持这些功能,并实现触控和显示之间的精细协调。目前市面上有许多商业应用可以完成这项工作。
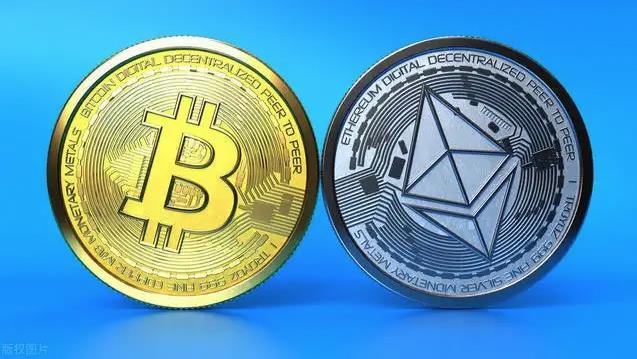
ZRX(0x)是一个基于以太坊区块链的开放协议,用于实现分布式交易和去中心化交易所(DEX)功能。作为0x协议的原生代币,ZRX可用于支付交易费用、治理协议变更和获取平台优惠。1.ZRX币升值空间展望:从技术角度来看,ZRX作为0x协议的核心代币,在去中心化交易所的应用逐渐增多,市场对其认可度也在增加。以下是几个关键因素,有助于提升ZRX币的价值空间:市场需求潜力大、社区活跃度高、开发者生态繁荣等。这些因素共同促进了ZRX的广泛应用和使用,进而推动了其市场价格的上升。市场需求的增长潜力,意味着更
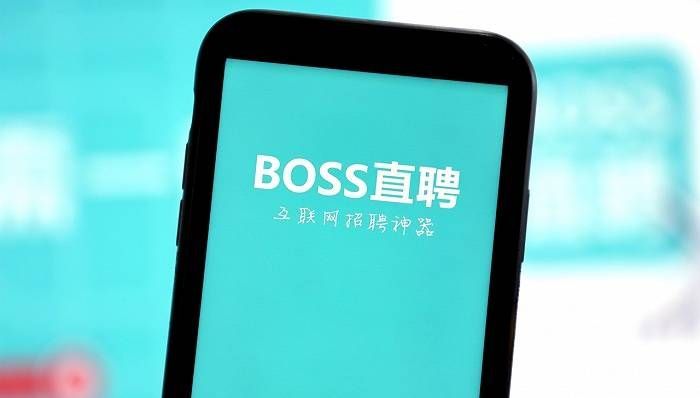
BOSS直聘怎么创建多个简历?BOSS直聘是很多小伙伴找工作的一大招聘平台,为用户们提供了非常多便利的求职服务。各位在使用BOSS直聘的时候,可以创建多个不同的简历,以便投送到不同的工作岗位上,获取到更高成功率的求职操作,各位如果对此感兴趣的话,就随小编一起来看看BOSS直聘双简历创建教程吧。BOSS直聘怎么创建多个简历1.登录Boss直聘:在您的电脑或手机上,登录您的Boss直聘账户。2.进入简历管理:在Boss直聘首页,点击“简历管理”,进入简历管理页面。3.创建新简历:在简历管理页面,点击
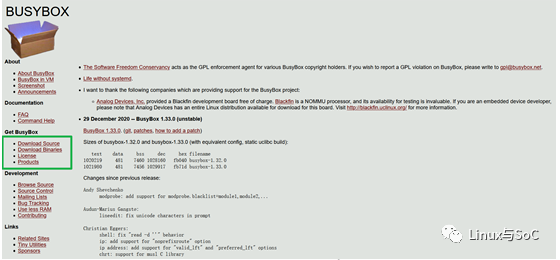
busybox概述众所周知,在Linux环境下,一切皆文件,文件可以表示一切。而文件系统则是这些普通组件的集合。在嵌入式领域中,常常使用基于busybox构建的rootfs来构建文件系统。busybox诞生至今已有近20年的历史,如今已成为嵌入式行业中主流的rootfs构建工具。busybox的代码是完全开源的。你可以进入官方网站,点击”GetBusyBox”下面的”DownloadSource”进入源码下载界面。“官方网站链接:https://busybox.net/”2.busybox的配置

最近,我正在进行一个项目,遇到了一个问题。在ARM上运行的ThreadX与DSP通信时采用了消息队列的方式传递消息(最终实现使用了中断和共享内存的方法)。然而,在实际的操作过程中,发现ThreadX经常崩溃。经过排查,发现问题出在传递消息的结构体没有考虑字节对齐的问题上。我想顺便整理一下关于C语言中字节对齐的问题,并与大家分享。一、概念字节对齐与数据在内存中的位置有关。如果一个变量的内存地址恰好是它长度的整数倍,那么它就被称为自然对齐。例如,在32位CPU下,假设一个整型变量的地址为0x0000
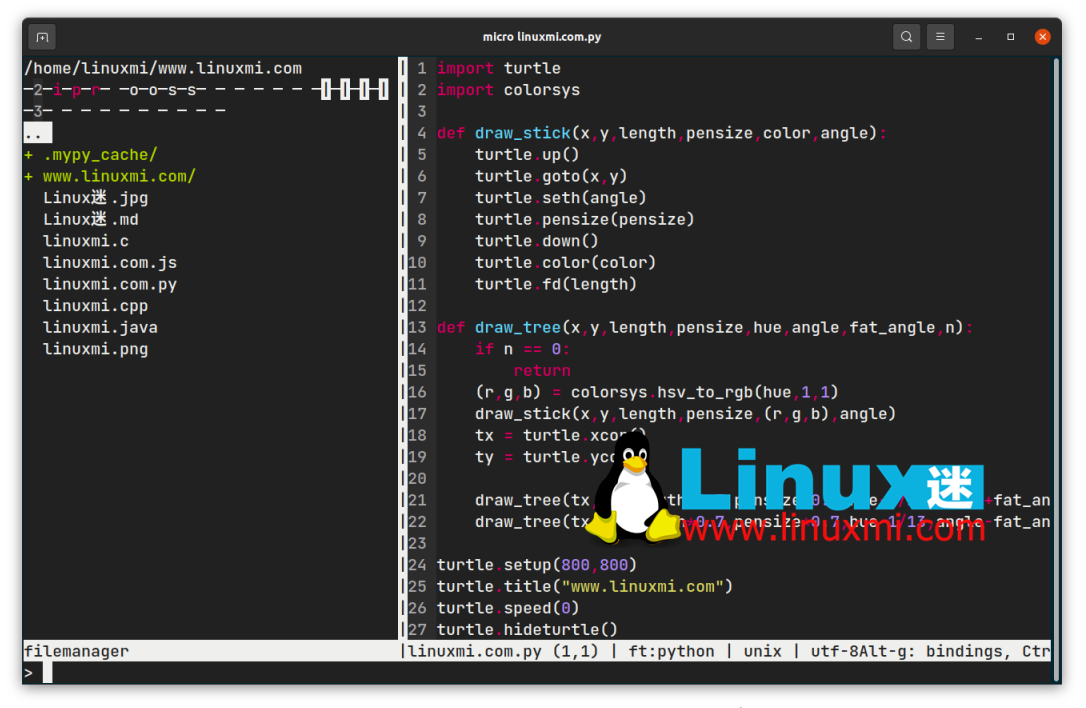
如果你厌倦了Vi和Vim的奇怪界面和繁琐的键绑定,为什么不试试Micro编辑器呢?命令行文本编辑器证明了Linux终端的实用性,让您可以在不离开终端的情况下进行文件编辑。这些编辑器使用的资源更少,速度也非常快,非常适合进行一些快速编辑。一些流行的命令行文本编辑器包括Vi、Vim和Nano。它们在大多数Linux发行版中都预装了。然而,对于初学者来说,学习Vi或Vim的曲线和键绑定可能有些困难。这时,Micro文本编辑器就成为了一个更简单的选择。Micro与其他编辑相比的表现如何Micro宣称自己

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
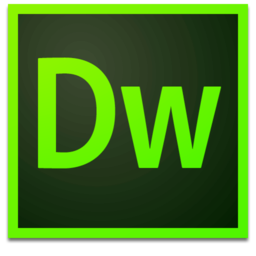
Dreamweaver Mac version
Visual web development tools
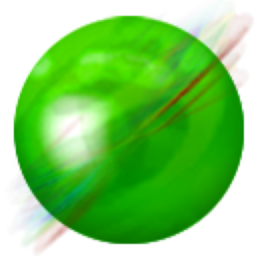
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
