


Use MyBatis annotation dynamic SQL to improve the flexibility of SQL statements
When using Java for database interaction, the writing of SQL statements is an important link. MyBatis is an excellent Java persistence framework that provides a flexible way to write SQL statements and has good maintainability. In MyBatis, we can use annotations to dynamically construct SQL statements to meet different query requirements or dynamic business logic changes. This article will introduce how to use MyBatis to annotate dynamic SQL to improve the flexibility of SQL statements, and give specific code examples.
Before using MyBatis to annotate dynamic SQL, we need to add MyBatis-related libraries to the project's dependencies, and configure MyBatis data sources and other necessary configuration items. Next, we will introduce a simple user query function as an example.
Suppose we have a User entity class, including attributes such as id, name, age, etc. We need to implement a function to query users based on different query conditions. The traditional approach is to dynamically construct query statements by splicing SQL strings. This involves certain security risks and makes the code less readable. Using MyBatis to annotate dynamic SQL can solve these problems well.
First, define the method getUserList to query users in our UserMapper interface, as shown below:
import org.apache.ibatis.annotations.Mapper; import org.apache.ibatis.annotations.Select; import java.util.List; @Mapper public interface UserMapper { @Select("SELECT * FROM user WHERE 1=1" + "<if test="name != null"> AND name = #{name}</if>" + "<if test="age != null"> AND age = #{age}</if>") List<User> getUserList(String name, Integer age); }
In the above code, we use the MyBatis annotation @Select to mark this A query method. In the value attribute of the annotation, we use a dynamic SQL statement with conditional judgment to query. Among them, "<if test="name != null"> AND name = #{name}</if>"
means that if name is not empty, then concatenate AND name = #{name}
. This allows you to dynamically build SQL statements based on the incoming query conditions.
Next, we need to define the corresponding attributes and getter and setter methods in our User entity class. In this way, MyBatis can automatically map the query results to the User object.
Finally, we can call this getUserList method in our business logic:
@Autowired private UserMapper userMapper; public List<User> searchUsers(String name, Integer age) { return userMapper.getUserList(name, age); }
In this example, we pass the incoming query conditions as parameters to the getUserList method and get the query result. In this way, we can easily query users based on different conditions, and the readability of the code has also been greatly improved.
In addition to the splicing of dynamic conditions, MyBatis annotations also provide other functions, such as dynamic sorting, dynamic paging, etc. We can flexibly use these functions in annotations according to specific business needs.
To summarize, using MyBatis to annotate dynamic SQL can improve the flexibility of SQL statements, allowing us to dynamically construct SQL statements according to different business needs. Using annotated dynamic SQL can improve the readability and maintainability of the code and avoid the risk of manually splicing SQL strings. When using annotated dynamic SQL, we only need to define the conditional judgment and dynamic splicing in the SQL statement, and MyBatis will automatically generate the corresponding SQL statement based on the incoming parameters. In this way, we can focus more on the development of business logic and improve development efficiency.
I hope that through the introduction of this article, readers can understand how to use MyBatis to annotate dynamic SQL to improve the flexibility of SQL statements, and get started quickly through specific code examples. Hope this article helps you!
The above is the detailed content of Use MyBatis to annotate dynamic SQL to improve the flexibility of SQL statements. For more information, please follow other related articles on the PHP Chinese website!
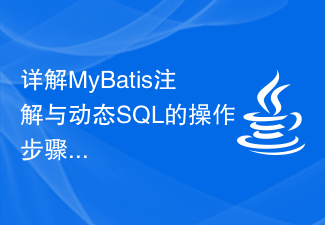
MyBatis注解动态SQL的使用方法详解IntroductiontotheusageofMyBatisannotationdynamicSQLMyBatis是一个持久层框架,为我们提供了便捷的持久化操作。在实际开发中,通常需要根据业务需求来动态生成SQL语句,以实现灵活的数据操作。MyBatis注解动态SQL正是为了满足这一需求而设计的,本
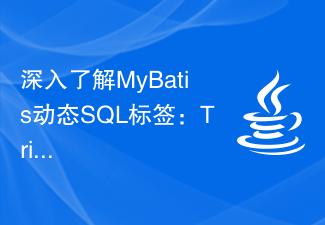
MyBatis是一个轻量级的Java持久层框架,它提供了许多方便的SQL语句拼接功能,其中的动态SQL标签是其强大之处之一。在MyBatis中,Trim标签是一种很常用的标签,用于动态地拼接SQL语句。在本文中,我们将深入了解MyBatis中的Trim标签的功能,并提供一些具体的代码示例。1.Trim标签简介在MyBatis中,Trim标签用于去除生成的S
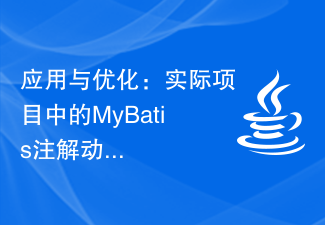
MyBatis注解动态SQL在实际项目中的应用与优化引言:MyBatis是一款优秀的持久层框架,它提供了多种SQL映射的方式,包括XML配置文件和注解。其中注解动态SQL是MyBatis的一项强大的功能,可以在运行时根据条件动态生成SQL语句,适用于处理复杂的业务逻辑。本文将介绍MyBatis注解动态SQL在实际项目中的应用,同时分享一些优化技巧与代码示例。
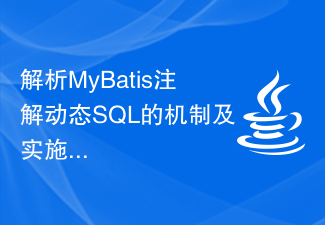
深入理解MyBatis注解动态SQL的原理与实现MyBatis是一个流行的Java持久化框架,它提供了一种方便的方式来处理数据库操作,同时也支持动态SQL。动态SQL是指根据不同的条件,在运行时动态地生成不同的SQL语句。MyBatis提供了两种实现动态SQL的方式,分别是XML配置方式和注解方式。本文将深入解析MyBatis注
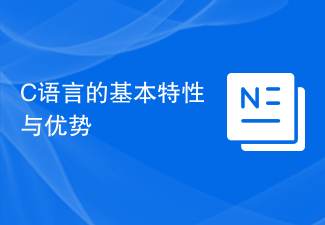
C语言的基本特性与优势作为一门被广泛应用的编程语言,C语言具有许多独特的特性和优势,使其成为编程领域中的重要工具。本文将探讨C语言的基本特性和其所具有的优势,并结合具体的代码示例进行解释。一、C语言的基本特性简洁高效:C语言的语法简洁明了,能够用较少的代码实现复杂的功能,因此编写的程序具有高效性和可读性。过程化编程:C语言主要支持过程化编程,即按照顺序执行语
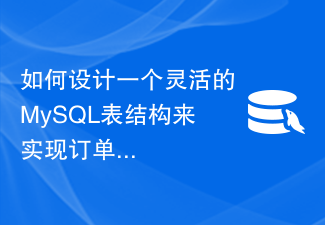
如何设计一个灵活的MySQL表结构来实现订单管理功能?订单管理是许多企业和电商网站的核心功能之一。为了实现这个功能,一个重要的步骤是设计一个灵活的MySQL表结构来存储订单相关的数据。一个好的表结构设计能够提高系统的性能和可维护性。本文将介绍如何设计一个灵活的MySQL表结构,并提供具体的代码示例来辅助理解。订单表(Order)订单表是存储订单信息的主要表。
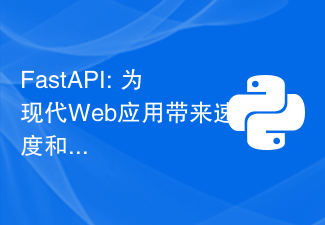
FastAPI:为现代Web应用带来速度和灵活性,需要具体代码示例引言:如今,Web应用的需求日益增长,用户对速度和灵活性的要求也越来越高。为了满足这种需求,开发人员需要选择合适的框架来构建高性能的Web应用。FastAPI是一个新兴的PythonWeb框架,它提供了出色的性能和灵活性,使得开发人员能够快速构建高效的Web应用。本文将介绍FastAPI框
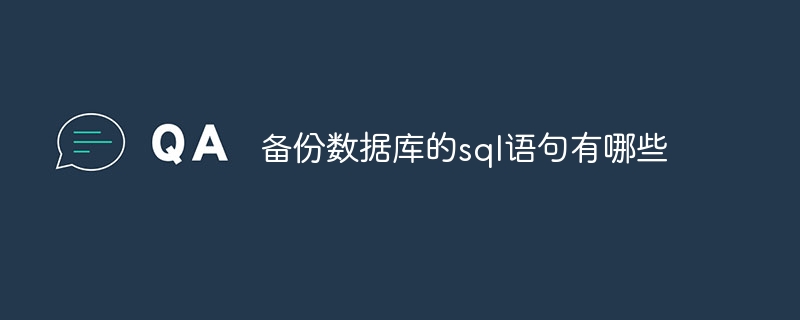
备份数据库的sql语句有mysqldump命令、pg_dump命令、expdp命令、BACKUP DATABASE命令、mongodump命令和redis-cli命令。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!
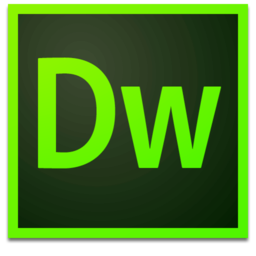
Dreamweaver Mac version
Visual web development tools
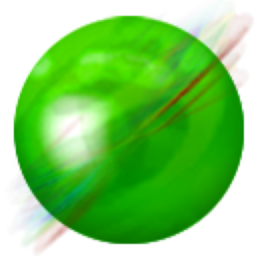
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
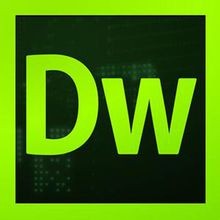
Dreamweaver CS6
Visual web development tools