


Anatomy of PHP Design Patterns: A Powerful Tool for Solving Common Programming Problems
PHP design pattern is an indispensable tool for programmers in the development process and can help solve various common programming problems. PHP editor Apple will take you through an in-depth dissection of PHP design patterns in this article, exploring its principles, application scenarios and actual case analysis. By learning and mastering design patterns, we can make our code more flexible and maintainable, and improve development efficiency. Let us explore the secrets of design patterns together!
PHP Design patterns are a set of general programming solutions for solving common software development problems. They provide a structured approach to solving common challenges such as creating reusable code, handling object interactions, and managing complexity.
Types of PHP design patterns
php Design patterns are divided into three categories:
- Creative pattern: Used to create objects, such as singleton pattern, factory method pattern and builder pattern.
- Structural pattern: Used to organize and combine objects, such as adapter pattern, decorator pattern and composition pattern.
- Behavioral mode: Used to coordinate object interaction, such as command mode, strategy mode and observer mode.
Creational pattern example: Factory method pattern
interface ShapeInterface { public function draw(); } class Square implements ShapeInterface { public function draw() { echo "Drawing a square.<br>"; } } class Circle implements ShapeInterface { public function draw() { echo "Drawing a circle.<br>"; } } class ShapeFactory { public static function create($shapeType) { switch ($shapeType) { case "square": return new Square(); case "circle": return new Circle(); default: throw new InvalidArgumentException("Invalid shape type."); } } } // Usage $square = ShapeFactory::create("square"); $square->draw(); // Output: Drawing a square.
Structural pattern example: Adapter pattern
class TargetInterface { public function operation() { echo "Target operation.<br>"; } } class Adaptee { public function specificOperation() { echo "Adaptee operation.<br>"; } } class Adapter implements TargetInterface { private $adaptee; public function __construct(Adaptee $adaptee) { $this->adaptee = $adaptee; } public function operation() { $this->adaptee->specificOperation(); } } // Usage $adaptee = new Adaptee(); $adapter = new Adapter($adaptee); $adapter->operation(); // Output: Adaptee operation.
Behavioral pattern example: Strategy pattern
interface StrategyInterface { public function calculate($a, $b); } class AdditionStrategy implements StrategyInterface { public function calculate($a, $b) { return $a + $b; } } class SubtractionStrategy implements StrategyInterface { public function calculate($a, $b) { return $a - $b; } } class Context { private $strategy; public function setStrategy(StrategyInterface $strategy) { $this->strategy = $strategy; } public function executeStrategy($a, $b) { return $this->strategy->calculate($a, $b); } } // Usage $context = new Context(); $context->setStrategy(new AdditionStrategy()); echo $context->executeStrategy(10, 5); // Output: 15 $context->setStrategy(new SubtractionStrategy()); echo $context->executeStrategy(10, 5); // Output: 5
benefit
Using PHP design patterns can bring the following benefits:
- Improve code quality: Design patterns follow established best practices, thereby reducing errors and improving code reliability.
- Enhance readability: By using common patterns, the code is easier to understand and maintain.
- Improve reusability: Design patterns provide reusable solutions and reduce code duplication.
- Promote collaboration: Developers can communicate on design patterns to promote team collaboration.
- Improve design flexibility: Design patterns allow you to modify your code without affecting the rest of the code.
in conclusion
PHP design patterns are practical tools for solving common programming problems. By understanding and effectively utilizing these patterns, you can significantly improve code quality, readability, maintainability, and reusability. Be sure to carefully select and apply a design pattern to your specific needs to get the most out of it.
The above is the detailed content of Anatomy of PHP Design Patterns: A Powerful Tool for Solving Common Programming Problems. For more information, please follow other related articles on the PHP Chinese website!
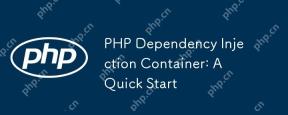
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
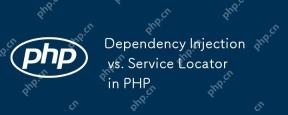
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
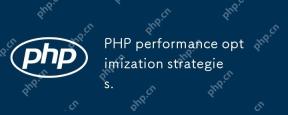
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
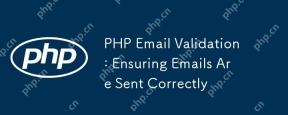
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl
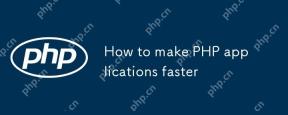
TomakePHPapplicationsfaster,followthesesteps:1)UseOpcodeCachinglikeOPcachetostoreprecompiledscriptbytecode.2)MinimizeDatabaseQueriesbyusingquerycachingandefficientindexing.3)LeveragePHP7 Featuresforbettercodeefficiency.4)ImplementCachingStrategiessuc
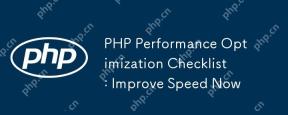
ToimprovePHPapplicationspeed,followthesesteps:1)EnableopcodecachingwithAPCutoreducescriptexecutiontime.2)ImplementdatabasequerycachingusingPDOtominimizedatabasehits.3)UseHTTP/2tomultiplexrequestsandreduceconnectionoverhead.4)Limitsessionusagebyclosin
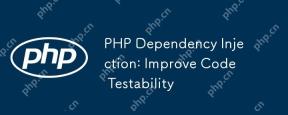
Dependency injection (DI) significantly improves the testability of PHP code by explicitly transitive dependencies. 1) DI decoupling classes and specific implementations make testing and maintenance more flexible. 2) Among the three types, the constructor injects explicit expression dependencies to keep the state consistent. 3) Use DI containers to manage complex dependencies to improve code quality and development efficiency.
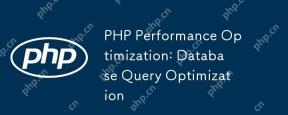
DatabasequeryoptimizationinPHPinvolvesseveralstrategiestoenhanceperformance.1)Selectonlynecessarycolumnstoreducedatatransfer.2)Useindexingtospeedupdataretrieval.3)Implementquerycachingtostoreresultsoffrequentqueries.4)Utilizepreparedstatementsforeffi


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
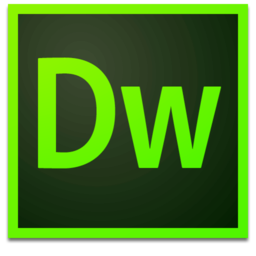
Dreamweaver Mac version
Visual web development tools
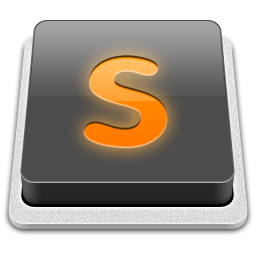
SublimeText3 Mac version
God-level code editing software (SublimeText3)
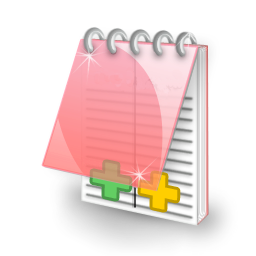
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
